Introduction to inherited features and keywords
Inherited features:
(Recommended tutorial: java introductory tutorial)
Subclass Possess non-private properties and methods of the parent class.
Subclasses can have their own properties and methods, that is, subclasses can extend parent classes.
Subclasses can implement the methods of the parent class in their own way.
Java's inheritance is single inheritance, but it can be multiple inheritance. Single inheritance means that a subclass can only inherit one parent class. Multiple inheritance means, for example, class A inherits class B, and class B Inherit class C, so according to the relationship, class C is the parent class of class B, and class B is the parent class of class A. This is a feature that distinguishes Java inheritance from C inheritance.
Improves the coupling between classes (the disadvantage of inheritance is that high coupling will cause the closer the connection between codes and the worse the code independence).
Keywords:
Inheritance can be achieved using the two keywords extends and implements, and all classes inherit from java.lang .Object, when a class does not inherit the two keywords, it inherits the object ancestor class by default (this class is in the java.lang package, so there is no need to import).
extends keyword
In Java, class inheritance is single inheritance, that is to say, a subclass can only have one parent class, so extends can only inherit one class.
Example:
public class Animal { private String name; private int id; public Animal(String myName, String myid) { //初始化属性值 } public void eat() { //吃东西方法的具体实现 } public void sleep() { //睡觉方法的具体实现 } } public class Penguin extends Animal{ }
(Video tutorial recommendation: java course)
implements keyword
Use the implements keyword in disguise Java has the feature of multiple inheritance. When a class inherits an interface, it can inherit multiple interfaces at the same time (the interfaces are separated by commas).
public interface A { public void eat(); public void sleep(); } public interface B { public void show(); } public class C implements A,B { }
The above is the detailed content of Introduction to inherited features and keywords. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


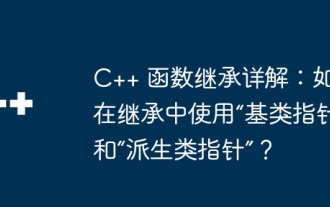
In function inheritance, use "base class pointer" and "derived class pointer" to understand the inheritance mechanism: when the base class pointer points to the derived class object, upward transformation is performed and only the base class members are accessed. When a derived class pointer points to a base class object, a downward cast is performed (unsafe) and must be used with caution.
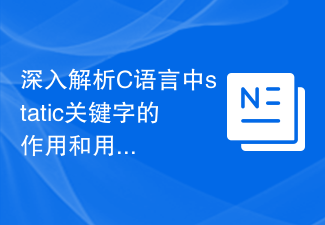
In-depth analysis of the role and usage of the static keyword in C language. In C language, static is a very important keyword, which can be used in the definition of functions, variables and data types. Using the static keyword can change the link attributes, scope and life cycle of the object. Let’s analyze the role and usage of the static keyword in C language in detail. Static variables and functions: Variables defined using the static keyword inside a function are called static variables, which have a global life cycle
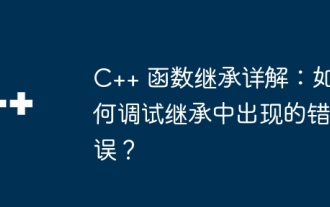
Inheritance error debugging tips: Ensure correct inheritance relationships. Use the debugger to step through the code and examine variable values. Make sure to use the virtual modifier correctly. Examine the inheritance diamond problem caused by hidden inheritance. Check for unimplemented pure virtual functions in abstract classes.
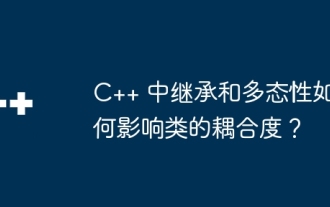
Inheritance and polymorphism affect the coupling of classes: Inheritance increases coupling because the derived class depends on the base class. Polymorphism reduces coupling because objects can respond to messages in a consistent manner through virtual functions and base class pointers. Best practices include using inheritance sparingly, defining public interfaces, avoiding adding data members to base classes, and decoupling classes through dependency injection. A practical example showing how to use polymorphism and dependency injection to reduce coupling in a bank account application.
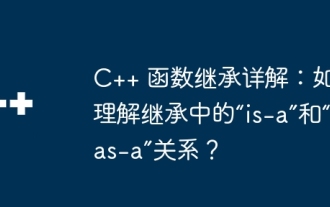
Detailed explanation of C++ function inheritance: Master the relationship between "is-a" and "has-a" What is function inheritance? Function inheritance is a technique in C++ that associates methods defined in a derived class with methods defined in a base class. It allows derived classes to access and override methods of the base class, thereby extending the functionality of the base class. "is-a" and "has-a" relationships In function inheritance, the "is-a" relationship means that the derived class is a subtype of the base class, that is, the derived class "inherits" the characteristics and behavior of the base class. The "has-a" relationship means that the derived class contains a reference or pointer to the base class object, that is, the derived class "owns" the base class object. SyntaxThe following is the syntax for how to implement function inheritance: classDerivedClass:pu
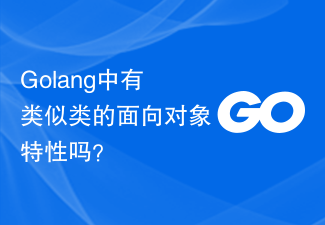
There is no concept of a class in the traditional sense in Golang (Go language), but it provides a data type called a structure, through which object-oriented features similar to classes can be achieved. In this article, we'll explain how to use structures to implement object-oriented features and provide concrete code examples. Definition and use of structures First, let's take a look at the definition and use of structures. In Golang, structures can be defined through the type keyword and then used where needed. Structures can contain attributes
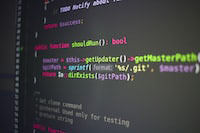
What is object-oriented programming? Object-oriented programming (OOP) is a programming paradigm that abstracts real-world entities into classes and uses objects to represent these entities. Classes define the properties and behavior of objects, and objects instantiate classes. The main advantage of OOP is that it makes code easier to understand, maintain and reuse. Basic Concepts of OOP The main concepts of OOP include classes, objects, properties and methods. A class is the blueprint of an object, which defines its properties and behavior. An object is an instance of a class and has all the properties and behaviors of the class. Properties are characteristics of an object that can store data. Methods are functions of an object that can operate on the object's data. Advantages of OOP The main advantages of OOP include: Reusability: OOP can make the code more
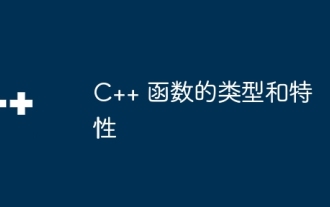
C++ functions have the following types: simple functions, const functions, static functions, and virtual functions; features include: inline functions, default parameters, reference returns, and overloaded functions. For example, the calculateArea function uses π to calculate the area of a circle of a given radius and returns it as output.
