Webpack implements Node.js code hot replacement_node.js
For the past two days, I have asked about this issue on Gitter, Twitter, and GitHub, but there has been no response for two days
It turns out that the blogger jlongster ignored me, and I didn’t know the contact information of the author of Webpack
He seemed to have seen the last message posted on Gitter, so he roughly explained it. It was so enlightening...
https://github.com/webpack/docs/issues/45#issuecomment-149793458
1 2 3 4 5 6 7 8 9 10 11 12 |
|
The original words will not be translated. After understanding, the main thing is how to configure Webpack and how to run the script
I wrote it again. The code is only so short, and hot replacement is implemented:
https://github.com/jiyinyiyong/webpack-backend-HMR-demo
The code can be copied from jlongster’s configuration tutorial:
http://jlongster.com/Backend-Apps-with-Webpack--Part-II
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
If running in command line environment, please note that it is webpack instead of webpack-dev-server
Pay attention to the & running in the background just to avoid blocking. If you have two terminals, just open two
1 2 3 |
|
I wrote two test files, one is the modified code src/lib.coffee:
1 2 3 4 |
|
Another entry file src/main.coffee contains code to handle module replacement:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
Run the demo and you will know the effect. setInterval is not affected by substitution
In the build/ directory, each modification will generate a JSON file to record the modified content:
0.1dadeb2eb7b01e150126.hot-update.js 0.c1d0d73de39660806d0c.hot-update.js 2849b61a15d31ffe5e08.hot-update.json 0.99ea3ea7633f6b3750e6.hot-update.js 0.ea a7b323eba37ae58997.hot-update.js 9b4a5ad617ec1dbc48a3.hot-update.json fb584971920454f9ccbe. hot-update.json
0.9abf25005c61357a0ce5.hot-update.js 0.fb584971920454f9ccbe.hot-update.js a664b5851a99ac0865ca.hot-update.json
0.9b4a5ad617ec1dbc48a3.hot-update.js 1dadeb2eb7b01e150126.hot-update.json bundle.js
0.a664b5851a99ac0865ca.hot-update.js 256267122c6d325755b0.hot-update.json c1d0d73de39660806d0c.hot-update.json
The specific file content is like this, which can be roughly considered to contain the information needed to identify updates:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
|
Other plans
I was looking for solutions on the Internet during the day, and posted a post on the forum asking about this matter. There are two main existing solutions with relatively clear explanations, which are worth learning from
One is on Baidu’s technology blog, which probably describes how to process module objects, that is, manually monitor file modifications, then clear the module cache, and remount the module
The ideas are clear and carefully considered. Although the code is a bit redundant, you can still give it a try:
http://www.jb51.net/article/73739.htm
The other one seems to be a hack on require.extensions, adding operations and events. When the module file is updated, the corresponding module is automatically updated, and an event is emitted. Through this effect, the location referenced by the module can be processed. , using new code, this should be said to be relatively crude, after all, not all codes are easy to replace
https://github.com/rlidwka/node-hotswap
Impressions
Considering that I have already hung myself on the Webpack tree, I don’t plan to study it in depth. Maybe Node.js officially optimizes lib/module.js to get good functions. However, JavaScript is not The community where the use of immutable data is popular cannot compare with Erlang, because code replacement involves the problem of status update, which is difficult to do. It is easier to restart, and restart now has three options for you to choose: node-dev supervisor nodemon
For me, the main reason is that the Cumulo solution has a huge dependence on WebSocket. Now front-end development can update the code on the server and the client automatically updates,
Through the mechanisms of Webpack and React, DOM and pure function modules are partially updated. If the development environment can also be hot-replaced, this will greatly improve development efficiency. I originally thought that hot-replacement was out of reach, but it is very possible. It’s an efficiency improvement within reach!
There may be pitfalls behind, after all, black technology... I’ll tell you when you encounter it
If you are interested, you can take a closer look at several related masterpieces written by jlongster, which are very helpful:
http://jlongster.com/archive

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










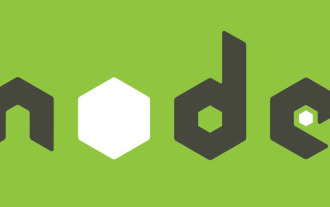
This article will give you an in-depth understanding of the memory and garbage collector (GC) of the NodeJS V8 engine. I hope it will be helpful to you!
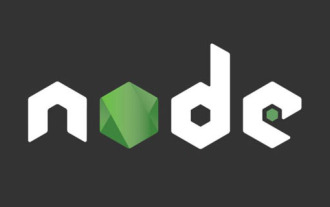
The Node service built based on non-blocking and event-driven has the advantage of low memory consumption and is very suitable for handling massive network requests. Under the premise of massive requests, issues related to "memory control" need to be considered. 1. V8’s garbage collection mechanism and memory limitations Js is controlled by the garbage collection machine
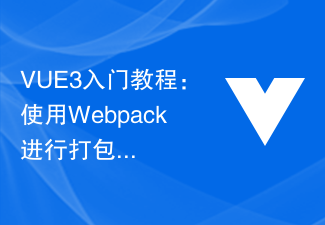
Vue is an excellent JavaScript framework that can help us quickly build interactive and efficient web applications. Vue3 is the latest version of Vue, which introduces many new features and functionality. Webpack is currently one of the most popular JavaScript module packagers and build tools, which can help us manage various resources in our projects. This article will introduce how to use Webpack to package and build Vue3 applications. 1. Install Webpack

Choosing a Docker image for Node may seem like a trivial matter, but the size and potential vulnerabilities of the image can have a significant impact on your CI/CD process and security. So how do we choose the best Node.js Docker image?
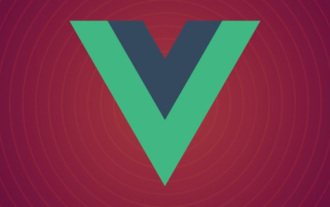
Differences: 1. The startup speed of the webpack server is slower than that of Vite; because Vite does not require packaging when starting, there is no need to analyze module dependencies and compile, so the startup speed is very fast. 2. Vite hot update is faster than webpack; in terms of HRM of Vite, when the content of a certain module changes, just let the browser re-request the module. 3. Vite uses esbuild to pre-build dependencies, while webpack is based on node. 4. The ecology of Vite is not as good as webpack, and the loaders and plug-ins are not rich enough.
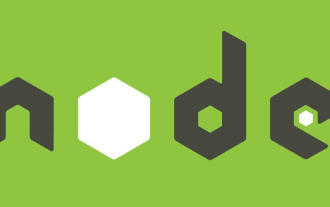
The file module is an encapsulation of underlying file operations, such as file reading/writing/opening/closing/delete adding, etc. The biggest feature of the file module is that all methods provide two versions of **synchronous** and **asynchronous**, with Methods with the sync suffix are all synchronization methods, and those without are all heterogeneous methods.
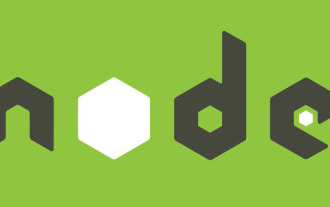
The event loop is a fundamental part of Node.js and enables asynchronous programming by ensuring that the main thread is not blocked. Understanding the event loop is crucial to building efficient applications. The following article will give you an in-depth understanding of the event loop in Node. I hope it will be helpful to you!
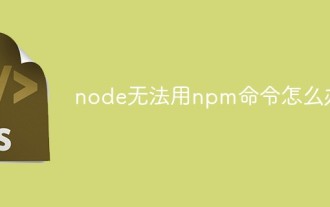
The reason why node cannot use the npm command is because the environment variables are not configured correctly. The solution is: 1. Open "System Properties"; 2. Find "Environment Variables" -> "System Variables", and then edit the environment variables; 3. Find the location of nodejs folder; 4. Click "OK".
