Share a simple example of java polymorphism
A simple example of java polymorphism: first create an interface; then create two classes to determine the content of the speech; then print the time while speaking; and finally create a test class to run.
Simple example of java polymorphism:
The three necessary conditions for polymorphism (implementation):
Need to have inheritance (inherit class, inherit abstract class, implement interface)
Need to have overriding
Reference execution of parent type subtype
Example: Using polymorphism to realize speaking and printing the time of saying this sentence
Ideas and code:
1. Since I don’t know that the content of the speech is too abstract, I created an interface
public interface TimeSpeek { public abstract void getTimeSpeek(); }
2. The methods in the interface need to be implemented, so now I need to create two classes to determine the content of the speech
第一句话 public class SpeekOne implements TimeSpeek { @Override public void getTimeSpeek() { // TODO Auto-generated method stub System.out.println("停车坐爱枫林晚"); } } 第二句话 public class SpeekTwo implements TimeSpeek { @Override public void getTimeSpeek() { // TODO Auto-generated method stub System.out.println("霜叶红于二月花"); } }
3. After the content of the speech is determined, you need to print the time while speaking
import java.util.Date; public class GetTime { public GetTime(TimeSpeek getTimeSpeek) { this.getTime(getTimeSpeek); } public void getTime(TimeSpeek getTimeSpeek) { System.out.println("开始时间:" + new Date().getTime()); getTimeSpeek.getTimeSpeek(); } }
4. Create a test class and run
public class Test { public static void main(String[] args) { SpeekOne so = new SpeekOne(); SpeekTwo st = new SpeekTwo(); new GetTime(so); new GetTime(st); } }
Get the result through the above steps:
开始时间:1565510695244 停车坐爱枫林晚 开始时间:1565510695245 霜叶红于二月花
Related learning recommendations: java basics
The above is the detailed content of Share a simple example of java polymorphism. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


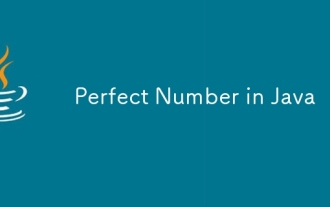
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
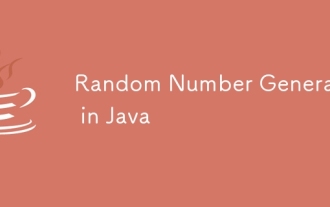
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
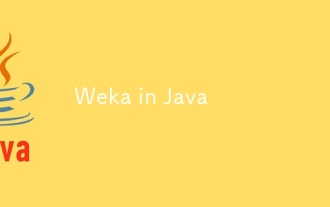
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
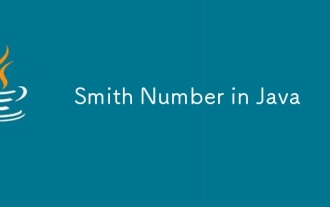
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
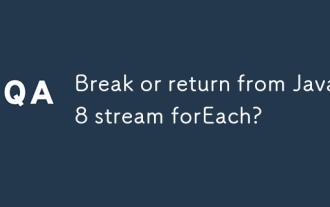
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
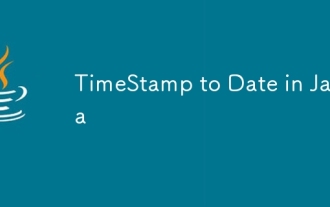
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
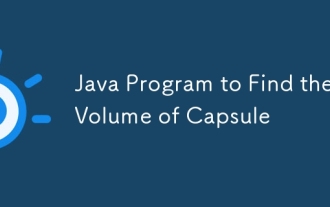
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
