How to make subquery in mysql?
In mysql, a subquery refers to nesting a query statement within another query statement. It can be implemented with the WHERE clause in the SELECT, UPDATE and DELETE statements. The syntax format of the WHERE clause is: "WHERE
(another query statement)".
(Recommended tutorial: mysql video tutorial)
Subquery is a comparison in MySQL Commonly used query methods, multi-table queries can be implemented through subqueries. Subquery refers to nesting one query statement within another query statement. Subqueries can be used in SELECT, UPDATE, and DELETE statements and can be nested at multiple levels. In actual development, subqueries often appear in the WHERE clause.
The syntax format of subquery in WHERE is as follows:
WHERE <表达式> <操作符> (子查询语句)
Among them, the operator can be a comparison operator and keywords such as IN, NOT IN, EXISTS, NOT EXISTS.
1) IN | NOT IN
When the expression is equal to a value in the result set returned by the subquery, TRUE is returned, otherwise FALSE is returned; if used Keyword NOT, the return value is exactly the opposite.
2) EXISTS | NOT EXISTS
is used to determine whether the result set of the subquery is empty. If the result set of the subquery is not empty, TRUE is returned, otherwise it is returned FALSE; if keyword NOT is used, the returned value is exactly the opposite.
Example 1
Use subquery to query the names of students taking Java courses in the tb_students_info table and tb_course table. The SQL statement and running results are as follows.
mysql> SELECT name FROM tb_students_info -> WHERE course_id IN (SELECT id FROM tb_course WHERE course_name = 'Java'); +-------+ | name | +-------+ | Dany | | Henry | +-------+ 2 rows in set (0.01 sec)
The results show that only Dany and Henry are taking Java courses. The above query process can also be divided into the following two steps to achieve the same effect.
1) First execute the inner query separately to find out the ID of the course Java in the tb_course table. The SQL statement and running results are as follows.
mysql> SELECT id FROM tb_course -> WHERE course_name = 'Java'; +----+ | id | +----+ | 1 | +----+ 1 row in set (0.00 sec)
You can see that the value of the id field that meets the conditions is 1.
2) Then execute the outer query to query the names of students whose course_id is equal to 1 in the tb_students_info table. The SQL statements and running results are as follows.
mysql> SELECT name FROM tb_students_info -> WHERE course_id IN (1); +-------+ | name | +-------+ | Dany | | Henry | +-------+ 2 rows in set (0.00 sec)
Conventionally, the outer SELECT query is called the parent query, and the query embedded in parentheses is called a subquery (subqueries must be placed within parentheses). When MySQL processes the SELECT statement in the above example, the execution flow is: first execute the subquery, and then execute the parent query.
Example 2
Similar to Example 1, use the NOT IN keyword in the SELECT statement to query the names of students who have not taken Java courses. The SQL statement and running results are as follows .
mysql> SELECT name FROM tb_students_info -> WHERE course_id NOT IN (SELECT id FROM tb_course WHERE course_name = 'Java'); +--------+ | name | +--------+ | Green | | Jane | | Jim | | John | | Lily | | Susan | | Thomas | | Tom | | LiMing | +--------+ 9 rows in set (0.01 sec)
It can be seen that the running result is exactly the opposite of Example 1. The students who have not studied Java courses are students other than Dany and Henry.
Example 3
Use the = operator to query the names of all students studying Python courses in the tb_course table and tb_students_info table. The SQL statement and running results are as follows.
mysql> SELECT name FROM tb_students_info -> WHERE course_id = (SELECT id FROM tb_course WHERE course_name = 'Python'); +------+ | name | +------+ | Jane | +------+ 1 row in set (0.00 sec)
The results show that the only student studying the Python course is Jane.
Example 4
Use the <> operator to query the names, SQL statements and running results of students who have not taken Python courses in the tb_course table and tb_students_info table as follows.
mysql> SELECT name FROM tb_students_info -> WHERE course_id <> (SELECT id FROM tb_course WHERE course_name = 'Python'); +--------+ | name | +--------+ | Dany | | Green | | Henry | | Jim | | John | | Lily | | Susan | | Thomas | | Tom | | LiMing | +--------+ 10 rows in set (0.00 sec)
It can be seen that the running result is just the opposite of Example 3. The students who have not studied Python courses are students other than Jane.
Example 5
Query whether there is a course with id=1 in the tb_course table. If it exists, query the records in the tb_students_info table. The SQL statement and running results are as follows .
mysql> SELECT * FROM tb_students_info -> WHERE EXISTS(SELECT course_name FROM tb_course WHERE id=1); +----+--------+------+------+--------+-----------+ | id | name | age | sex | height | course_id | +----+--------+------+------+--------+-----------+ | 1 | Dany | 25 | 男 | 160 | 1 | | 2 | Green | 23 | 男 | 158 | 2 | | 3 | Henry | 23 | 女 | 185 | 1 | | 4 | Jane | 22 | 男 | 162 | 3 | | 5 | Jim | 24 | 女 | 175 | 2 | | 6 | John | 21 | 女 | 172 | 4 | | 7 | Lily | 22 | 男 | 165 | 4 | | 8 | Susan | 23 | 男 | 170 | 5 | | 9 | Thomas | 22 | 女 | 178 | 5 | | 10 | Tom | 23 | 女 | 165 | 5 | | 11 | LiMing | 22 | 男 | 180 | 7 | +----+--------+------+------+--------+-----------+ 11 rows in set (0.01 sec)
As can be seen from the results, there is a record with id=1 in the tb_course table, so the EXISTS expression returns TRUE. After receiving TRUE, the outer query statement queries the table tb_students_info and returns all records.
The EXISTS keyword can be used together with other query conditions. The conditional expression and the EXISTS keyword are connected with AND and OR.
Example 6
Query whether there is a course with id=1 in the tb_course table. If it exists, query the records with the age field greater than 24 in the tb_students_info table. SQL statement And the running results are as follows.
mysql> SELECT * FROM tb_students_info -> WHERE age>24 AND EXISTS(SELECT course_name FROM tb_course WHERE id=1); +----+------+------+------+--------+-----------+ | id | name | age | sex | height | course_id | +----+------+------+------+--------+-----------+ | 1 | Dany | 25 | 男 | 160 | 1 | +----+------+------+------+--------+-----------+ 1 row in set (0.01 sec)
The results show that a record was queried from the tb_students_info table, and the age field value of this record is 25. The inner query statement retrieves records from the tb_course table and returns TRUE. The outer query statement starts the query. According to the query conditions, query the records with age greater than 24 from the tb_students_info table.
Expand
The functionality of subqueries can also be accomplished through table joins, but subqueries make SQL statements easier to read and write.
Generally speaking, table joins (inner joins and outer joins, etc.) can be replaced by subqueries, but the reverse is not necessarily true. Some subqueries cannot be replaced by table joins. Subqueries are more flexible, convenient, and diverse in form, and are suitable as filter conditions for queries, while table joins are more suitable for viewing data in connected tables.
The above is the detailed content of How to make subquery in mysql?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
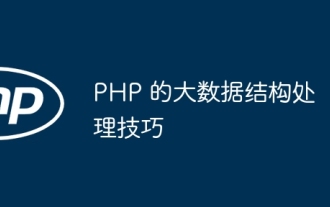
Big data structure processing skills: Chunking: Break down the data set and process it in chunks to reduce memory consumption. Generator: Generate data items one by one without loading the entire data set, suitable for unlimited data sets. Streaming: Read files or query results line by line, suitable for large files or remote data. External storage: For very large data sets, store the data in a database or NoSQL.
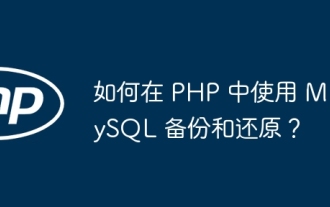
Backing up and restoring a MySQL database in PHP can be achieved by following these steps: Back up the database: Use the mysqldump command to dump the database into a SQL file. Restore database: Use the mysql command to restore the database from SQL files.
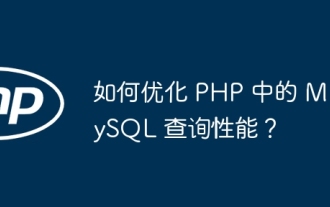
MySQL query performance can be optimized by building indexes that reduce lookup time from linear complexity to logarithmic complexity. Use PreparedStatements to prevent SQL injection and improve query performance. Limit query results and reduce the amount of data processed by the server. Optimize join queries, including using appropriate join types, creating indexes, and considering using subqueries. Analyze queries to identify bottlenecks; use caching to reduce database load; optimize PHP code to minimize overhead.
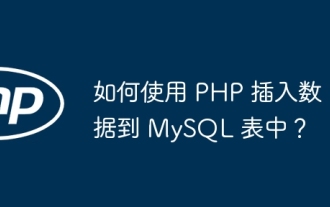
How to insert data into MySQL table? Connect to the database: Use mysqli to establish a connection to the database. Prepare the SQL query: Write an INSERT statement to specify the columns and values to be inserted. Execute query: Use the query() method to execute the insertion query. If successful, a confirmation message will be output.
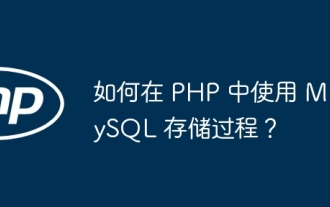
To use MySQL stored procedures in PHP: Use PDO or the MySQLi extension to connect to a MySQL database. Prepare the statement to call the stored procedure. Execute the stored procedure. Process the result set (if the stored procedure returns results). Close the database connection.

Creating a MySQL table using PHP requires the following steps: Connect to the database. Create the database if it does not exist. Select a database. Create table. Execute the query. Close the connection.

One of the major changes introduced in MySQL 8.4 (the latest LTS release as of 2024) is that the "MySQL Native Password" plugin is no longer enabled by default. Further, MySQL 9.0 removes this plugin completely. This change affects PHP and other app
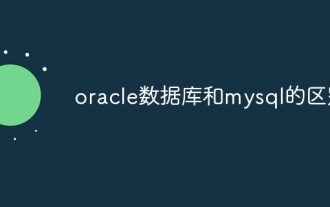
Oracle database and MySQL are both databases based on the relational model, but Oracle is superior in terms of compatibility, scalability, data types and security; while MySQL focuses on speed and flexibility and is more suitable for small to medium-sized data sets. . ① Oracle provides a wide range of data types, ② provides advanced security features, ③ is suitable for enterprise-level applications; ① MySQL supports NoSQL data types, ② has fewer security measures, and ③ is suitable for small to medium-sized applications.
