##3 | 131000 |
3 | 125000 |
4 | 235000 |
##4
265000 |
|
5
535000 |
|
Then, display these data on a two-dimensional graph, and treat each parameter (price, number of rooms) as 1 dimension:
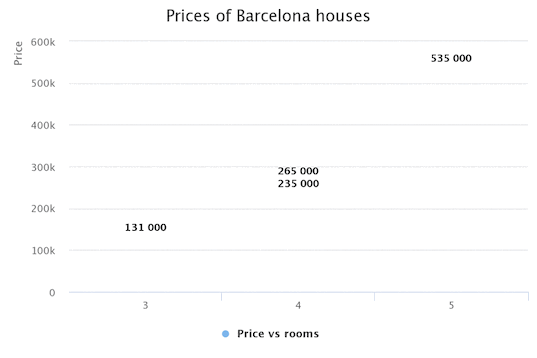
Then we can Draw a line and predict the rental price of a house with more rooms. This model is called linear regression, and it is one of the simplest models in machine learning. But this model is not good enough:
- There are only 5 data, so it is not reliable enough.
- There are only 2 parameters (price, room), but there are more factors that may affect the price: such as area, decoration, etc.
The first problem can be solved by adding more data, say a million. For the second question, more dimensions can be added. In a two-dimensional chart it is easy to understand the data and draw a line, in a three-dimensional chart you can use a plane:
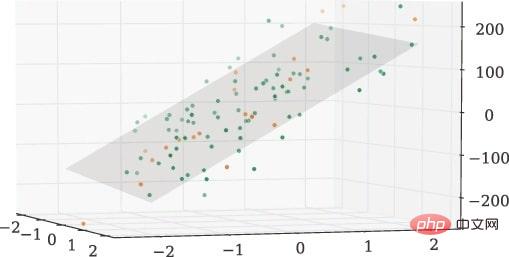
But what about when the dimensions of the data are three dimensions, four dimensions or even 1000000 When the dimension exceeds three dimensions, the brain has no way to visualize it on a chart, but the hyperplane can be calculated mathematically when the dimension exceeds three dimensions, and neural networks were born to solve this problem.
What is a neural network?
To understand what a neural network is, you need to know what a neuron is. A real neuron looks like this:
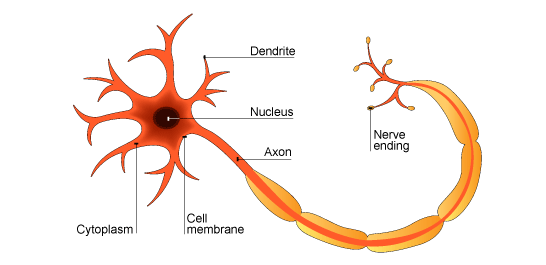
A neuron is composed of the following parts:
-
Dendrite : This is the input end of the data.
-
Axon: This is the output end.
-
Synapse (not represented in the diagram): This structure allows communication between one neuron and another. It is responsible for transmitting electrical signals between the nerve endings of axons and the dendrites of nearby neurons. These synapses are key to learning because they increase or decrease electrical activity depending on their use.
Neurons in machine learning (simplified):
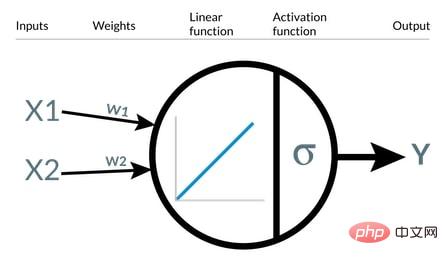
-
Inputs (inputs) : Inputs parameter.
-
Weights: Like synapses, used to better establish linear regression by adjusting neurons.
-
Linear function: Each neuron is like a linear regression function. For a linear regression model, only one neuron is enough.
-
Activation function: Some activation functions can be used to change the output from a scalar to another non-linear function. Common ones are sigmoid, RELU and tanh.
-
Output (output) : The calculated output after applying the activation function.
The activation function is very useful, and the power of neural networks is mainly attributed to it. Without any activation function, it is impossible to get an intelligent neuron network. Because even though you have multiple neurons in your neural network, the output of your neural network will always be a linear regression. Therefore, some mechanism is needed to transform each linear regression into nonlinear to solve nonlinear problems. These linear functions can be converted into nonlinear functions through the activation function:
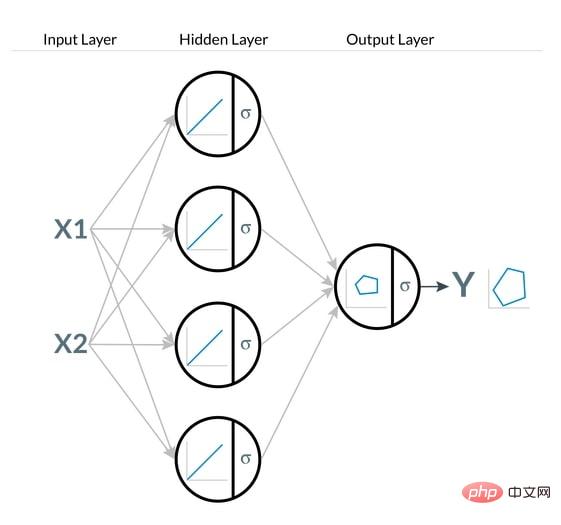
#Training model
As described in the 2D linear regression example, just Draw a line in the graph to predict new data. Still, the idea of "deep learning" is to have our neural network learn to draw this line. For a simple line, you can use a very simple neural network with only one neuron, but for a model that wants to do more complex things, such as classifying two sets of data, the network needs to be "trained" Learn how to get the following:
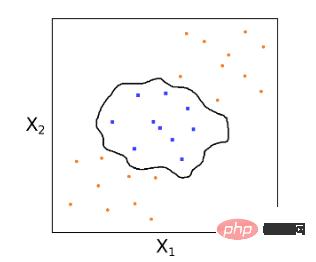
The process is not complicated because it is two-dimensional. Each model is used to describe a world, but the concept of "training" is very similar across all models. The first step is to draw a random line and improve it iteratively in the algorithm, correcting errors in the process during each iteration. This optimization algorithm is called Gradient Descent (algorithms with the same concept are also more complex SGD or ADAM, etc.). Each algorithm (linear regression, logarithmic regression, etc.) has a different cost function to measure the error, and the cost function will always converge to a certain point. It can be a convex or concave function, but it will eventually converge to a point with 0% error. Our goal is to achieve this.
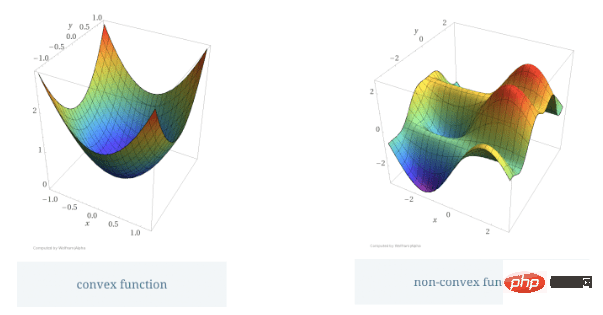
When using the gradient descent algorithm, we start from some random point in its cost function, but we don't know where it is! It's like being blindfolded and thrown on a mountain. If you want to go down the mountain, you have to go to the lowest point step by step. If the terrain is irregular (such as a concave function), the descent will be more complicated.
I won’t explain the “gradient descent” algorithm in depth here. It’s enough to remember that this is an optimization algorithm for minimizing prediction errors in the process of training AI models. This algorithm requires a lot of time and GPU for matrix multiplication. It is usually difficult to reach this convergence point on the first execution, so some hyperparameters need to be modified, such as the learning rate or adding regularization. After gradient descent iterations, the convergence point is approached when the error approaches 0%. This creates a model that can then be used to make predictions.

Training models with TensorFlow.js
TensorFlow.js provides an easy way to create neural networks. First create a LinearModel
class using the trainModel
method. We will use a sequential model. A sequential model is a model in which the output of one layer is the input to the next layer, i.e. when the model topology is a simple hierarchy with no branches or skips. Define the layers inside the trainModel
method (we use only one layer as it is enough to solve the linear regression problem):
import * as tf from '@tensorflow/tfjs';
/**
* 线性模型类
*/
export default class LinearModel {
/**
* 训练模型
*/
async trainModel(xs, ys){
const layers = tf.layers.dense({
units: 1, // 输出空间的纬度
inputShape: [1], // 只有一个参数
});
const lossAndOptimizer = {
loss: 'meanSquaredError',
optimizer: 'sgd', // 随机梯度下降
};
this.linearModel = tf.sequential();
this.linearModel.add(layers); // 添加一层
this.linearModel.compile(lossAndOptimizer);
// 开始模型训练
await this.linearModel.fit(
tf.tensor1d(xs),
tf.tensor1d(ys),
);
}
//...
}
Copy after login
Use this class for training:
const model = new LinearModel()
// xs 与 ys 是 数组成员(x-axis 与 y-axis)
await model.trainModel(xs, ys)
Copy after login
End of training Then you can start making predictions.
Prediction with TensorFlow.js
Although some hyperparameters need to be defined in advance when training the model, making general predictions is still easy. It is enough to pass the following code:
import * as tf from '@tensorflow/tfjs';
export default class LinearModel {
... //前面训练模型的代码
predict(value){
return Array.from(
this.linearModel
.predict(tf.tensor2d([value], [1, 1]))
.dataSync()
)
}
}
Copy after login
Now you can predict:
const prediction = model.predict(500) // 预测数字 500
console.log(prediction) // => 420.423
Copy after login

Using a pre-trained model in TensorFlow.js
Training the model is the hardest part. First, the data is standardized for training, and all hyperparameters need to be set correctly, etc. For us beginners, we can directly use those pre-trained models. TensorFlow.js can use many pretrained models and can also import external models created with TensorFlow or Keras. For example, you can directly use the posenet model (real-time human posture assessment) to do some interesting projects:
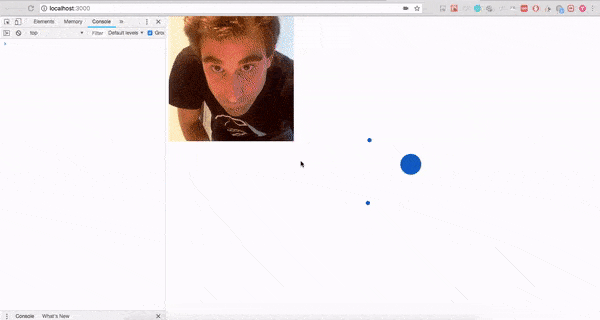
The code of this Demo: https://github.com/aralroca/posenet- d3
It is easy to use:
import * as posenet from '@tensorflow-models/posenet'
// 设置一些常数
const imageScaleFactor = 0.5
const outputStride = 16
const flipHorizontal = true
const weight = 0.5
// 加载模型
const net = await posenet.load(weight)
// 进行预测
const poses = await net.estimateSinglePose(
imageElement,
imageScaleFactor,
flipHorizontal,
outputStride
)
Copy after login
This JSON is pose Variable:
{
"score": 0.32371445304906,
"keypoints": [
{
"position": {
"y": 76.291801452637,
"x": 253.36747741699
},
"part": "nose",
"score": 0.99539834260941
},
{
"position": {
"y": 71.10383605957,
"x": 253.54365539551
},
"part": "leftEye",
"score": 0.98781454563141
}
// 后面还有: rightEye, leftEar, rightEar, leftShoulder, rightShoulder
// leftElbow, rightElbow, leftWrist, rightWrist, leftHip, rightHip,
// leftKnee, rightKnee, leftAnkle, rightAnkle...
]
}
Copy after login
You can see it from the official demo, use this model There are many interesting projects that can be developed.
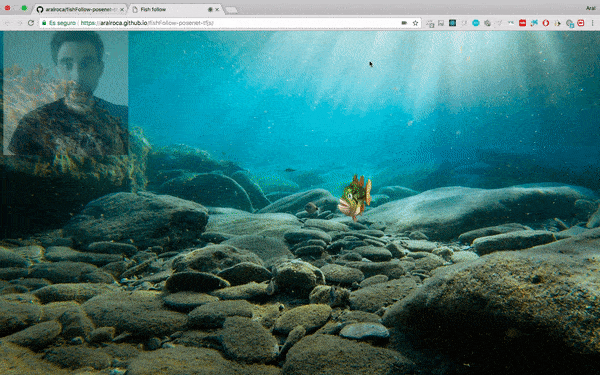
Source code of this project: https://github.com/aralroca/fishFollow-posenet-tfjs
Import Keras model
External models can be imported into TensorFlow.js. Below is a program for number recognition using Keras model (h5 format). First, use tfjs_converter to convert the format of the model.
pip install tensorflowjs
Copy after login
Use the converter:
tensorflowjs_converter --input_format keras keras/cnn.h5 src/assets
Copy after login
Finally, import the model into JS code:
// 载入模型
const model = await tf.loadModel('./assets/model.json')
// 准备图片
let img = tf.fromPixels(imageData, 1)
img = img.reshape([1, 28, 28, 1])
img = tf.cast(img, 'float32')
// 进行预测
const output = model.predict(img)
Copy after login
It only takes a few lines of code to complete. Of course, you can add more logic to the code to achieve more functions. For example, you can write numbers on canvas and then get their images for prediction.

Source code of this project: https://github.com/aralroca/MNIST_React_TensorFlowJS
Why should it be used in the browser?
Due to different devices, the efficiency may be very low when training the model in the browser. Using TensorFlow.js to use WebGL to train the model in the background is 1.5 to 2 times slower than using the Python version of TensorFlow.
But before the emergence of TensorFlow.js, there was no API that could directly use machine learning models in the browser. Now, models can be trained and used offline in browser applications. And predictions are faster because there are no requests to the server. Another benefit is low cost since all these calculations are done on the client side.
Summary
- A model is a simplified way of representing the real world that can be used to make predictions.
- You can use neural networks to create models.
- TensorFlow.js is a simple tool for creating neural networks.
English original address: https://aralroca.com/blog/first-steps-with-tensorflowjs
Author: Aral Roca
For more programming-related knowledge, please visit: Programming Courses! !