


Basic knowledge of using literals and functions in JavaScript_Basic knowledge
JavaScript literal
In programming languages, a literal is a constant, as in 3.14.
Number literals can be integers, decimals, or scientific notation (e).
3.14 1001 123e5
String literal can be written with double or single quotes:
"John Doe" 'John Doe'
Expression literal used for calculation:
5 + 6 5 * 10
Array literal defines an array:
[40, 100, 1, 5, 25, 10]
Object literal defines an object:
{firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"}
Function literal defines a function:
function myFunction(a, b) { return a * b;}
JavaScript function definition
JavaScript uses the keyword function to define functions.
A function can be defined by a declaration or it can be an expression.
Function declaration
In the previous tutorial, you already learned the syntax of function declaration:
function functionName(parameters) { 执行的代码 }
The function will not be executed immediately after it is declared, but will be called when we need it.
Example
function myFunction(a, b) { return a * b; }
Note semicolons are used to separate executable JavaScript statements.
Since a function declaration is not an executable statement, it does not end with a semicolon.
Function expression
JavaScript functions can be defined through an expression.
Function expressions can be stored in variables:
Example
var x = function (a, b) {return a * b};
After the function expression is stored in the variable, the variable can also be used as a function:
Example
var x = function (a, b) {return a * b}; var z = x(4, 3);
The above function is actually an anonymous function (the function has no name).
Functions are stored in variables and do not require a function name. They are usually called through the variable name.
Note The above function ends with a semicolon because it is an execution statement.
Function() constructor
In the above example, we learned that functions are defined through the keyword function.
Functions can also be defined through the built-in JavaScript function constructor (Function()).
Example
var myFunction = new Function("a", "b", "return a * b"); var x = myFunction(4, 3);
Actually, you don't have to use a constructor. The above example can be written as:
Example
var myFunction = function (a, b) {return a * b} var x = myFunction(4, 3);
Note In JavaScript, many times, you need to avoid using the new keyword.
Function Hoisting (Hoisting)
We already learned about "hoisting" in a previous tutorial.
Hoisting is JavaScript's default behavior of hoisting the current scope to the front.
Hoisting applies to variable declarations and function declarations.
Therefore, functions can be called before declaration:
myFunction(5); function myFunction(y) { return y * y; }
Hoisting is not possible when defining a function using an expression.
Self-calling function
Function expressions can "call themselves".
Self-calling expressions are called automatically.
This is automatically called if the expression is immediately followed by () .
Y cannot call the declared function itself.
Indicate that it is a function expression by adding parentheses:
Example
(function () { var x = "Hello!!"; // 我将调用自己 })();
The above function is actually an anonymous self-calling function (without a function name).
Function can be used as a value
JavaScript function used as a value:
Example
function myFunction(a, b) { return a * b; } var x = myFunction(4, 3);
JavaScript functions can be used as expressions:
Example
function myFunction(a, b) { return a * b; } var x = myFunction(4, 3) * 2;
Functions are objects
Using the typeof operator in JavaScript to determine the function type will return "function".
However, it is more accurate to describe a JavaScript function as an object.
JavaScript functions have properties and methods.
The arguments.length property returns the number of arguments received by the function calling process:
Example
function myFunction(a, b) { return arguments.length; }
The toString() method returns the function as a string:
Example
function myFunction(a, b) { return a * b; } var txt = myFunction.toString();

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


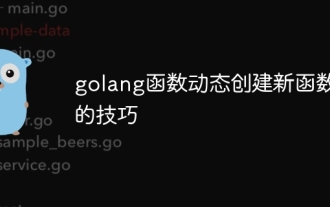
Go language provides two dynamic function creation technologies: closure and reflection. closures allow access to variables within the closure scope, and reflection can create new functions using the FuncOf function. These technologies are useful in customizing HTTP routers, implementing highly customizable systems, and building pluggable components.
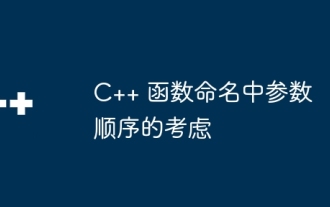
In C++ function naming, it is crucial to consider parameter order to improve readability, reduce errors, and facilitate refactoring. Common parameter order conventions include: action-object, object-action, semantic meaning, and standard library compliance. The optimal order depends on the purpose of the function, parameter types, potential confusion, and language conventions.
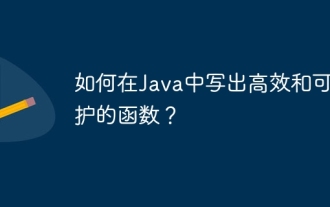
The key to writing efficient and maintainable Java functions is: keep it simple. Use meaningful naming. Handle special situations. Use appropriate visibility.
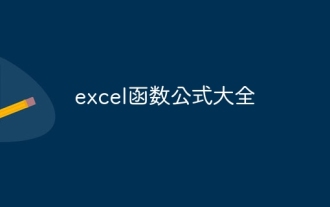
1. The SUM function is used to sum the numbers in a column or a group of cells, for example: =SUM(A1:J10). 2. The AVERAGE function is used to calculate the average of the numbers in a column or a group of cells, for example: =AVERAGE(A1:A10). 3. COUNT function, used to count the number of numbers or text in a column or a group of cells, for example: =COUNT(A1:A10) 4. IF function, used to make logical judgments based on specified conditions and return the corresponding result.
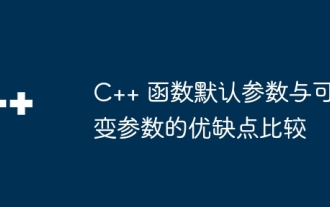
The advantages of default parameters in C++ functions include simplifying calls, enhancing readability, and avoiding errors. The disadvantages are limited flexibility and naming restrictions. Advantages of variadic parameters include unlimited flexibility and dynamic binding. Disadvantages include greater complexity, implicit type conversions, and difficulty in debugging.
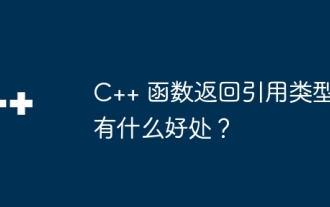
The benefits of functions returning reference types in C++ include: Performance improvements: Passing by reference avoids object copying, thus saving memory and time. Direct modification: The caller can directly modify the returned reference object without reassigning it. Code simplicity: Passing by reference simplifies the code and requires no additional assignment operations.
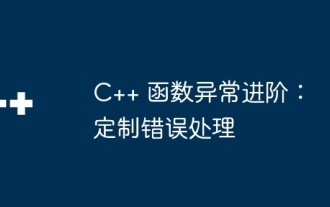
Exception handling in C++ can be enhanced through custom exception classes that provide specific error messages, contextual information, and perform custom actions based on the error type. Define an exception class inherited from std::exception to provide specific error information. Use the throw keyword to throw a custom exception. Use dynamic_cast in a try-catch block to convert the caught exception to a custom exception type. In the actual case, the open_file function throws a FileNotFoundException exception. Catching and handling the exception can provide a more specific error message.
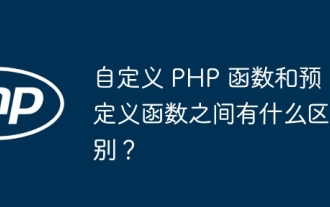
The difference between custom PHP functions and predefined functions is: Scope: Custom functions are limited to the scope of their definition, while predefined functions are accessible throughout the script. How to define: Custom functions are defined using the function keyword, while predefined functions are defined by the PHP kernel. Parameter passing: Custom functions receive parameters, while predefined functions may not require parameters. Extensibility: Custom functions can be created as needed, while predefined functions are built-in and cannot be modified.
