Why did swoole go from getting started to giving up?
swoole tutorialIntroducing why from getting started to giving up
Recommended (free): swoole tutorial
1. swoole source package installation
- Download the swoole source code:
git clone https://gitee.com/swoole/swoole.git
-
Through phpize (extend php extension module, create php plug-in module):
cd swoole
- Execute:
your /phpize/path
./configure --with-php-config=your/php/path/bin/php-config
- ## make && make install
- You can see the location of swoole.so
- My location is:
- /opt/ soft/php/lib/php/extensions/no-debug-non-zts-20170718/
- /opt/ soft/php/lib/php/extensions/no-debug-non-zts-20170718/
- Configure php.ini
- Add
- extension=swoole.so
Through the - extension=swoole.so
- php -m
command, you can see the php extension module
- Detect that swoole is installed successfully and php supports swoole
- cd your/swoole/path/examples/server
- php echo.php
(If the process is blocked, it means success)
- netstat -anp | grep 9501
(View the port number opened by swoole)
- cd your/swoole/path/examples/server
2. Network Communication Engine To learn swoole, you need to read the documents. swoole document1. Create the simplest tcp service through swooletcp server (tcp_server.php)
tcp client (tcp_client.php)//创建Server对象,监听 127.0.0.1:9501端口 $serv = new swoole_server("127.0.0.1", 9501); $serv->set([ 'worker_num' => 4, // worker进程数,cpu 1-4倍 'max_request' => 100, ]); /** * 监听连接进入事件 * $fd 客户端连接服务端的唯一标识 * $reactor_id 线程id */ $serv->on('connect', function ($serv, $fd, $reactor_id) { echo "Client: {$fd} - {$reactor_id} - Connect.\n"; }); //监听数据接收事件 $serv->on('receive', function ($serv, $fd, $reactor_id, $data) { $serv->send($fd, "Server: ".$data); }); //监听连接关闭事件 $serv->on('close', function ($serv, $fd) { echo "Client: Close.\n"; }); //启动服务器 $serv->start();Copy after login
2. Expansion: Four callbacks of php// 创建tcp客户端 $client = new swoole_client(SWOOLE_SOCK_TCP); // 连接tcp服务端 if (!$client->connect("127.0.0.1", 9501)) { echo '连接失败'; exit; } // php cli fwrite(STDOUT, '请输入:'); $msg = trim(fgets(STDIN)); // 发送消息给tcp服务端 if (!$client->send($msg)) { echo '发送消息失败'; exit; } // 接收 $result = $client->recv(); echo $result;Copy after login
- Anonymous function
$server->on('Request', function ($req, $resp) { echo "hello world"; });
- Class static method
class A { static function test($req, $resp) { echo "hello world"; } } $server->on('Request', 'A::Test'); $server->on('Request', array('A', 'Test'));
- Function
function my_onRequest($req, $resp) { echo "hello world"; } $server->on('Request', 'my_onRequest');
- Object method
class A { function test($req, $resp) { echo "hello world"; } } $object = new A(); $server->on('Request', array($object, 'test'));
Tips: View the opened worker process: ps aft | grep tcp_server.php
3. UDP server and client You can create it yourself according to the document
4. http service
// 监听所有地址和9501端口
$http = new swoole_http_server('0.0.0.0', 9501);
// 动静分离配置
$http->set([
// 开启静态请求
'enable_static_handler' => true,
// 静态资源目录
'document_root' => '/opt/app/code1/',
]);
$http->on('request', function ($request, $response) {
// 获取get请求的参数
$param = json_encode($request->get);
// 设置cookie
$response->cookie('name', 'ronaldo', time() + 1800);
// 输出到页面
$response->end("<h1>Hello Swoole - {$param}</h1>");
});
// 开启http服务
$http->start();
Copy after login
// 监听所有地址和9501端口 $http = new swoole_http_server('0.0.0.0', 9501); // 动静分离配置 $http->set([ // 开启静态请求 'enable_static_handler' => true, // 静态资源目录 'document_root' => '/opt/app/code1/', ]); $http->on('request', function ($request, $response) { // 获取get请求的参数 $param = json_encode($request->get); // 设置cookie $response->cookie('name', 'ronaldo', time() + 1800); // 输出到页面 $response->end("<h1>Hello Swoole - {$param}</h1>"); }); // 开启http服务 $http->start();
5. Create websocket service through swoolewebsocket server (websocket_server.php)
websocket client (websockt_client.html)// 监听所有地址和9502端口 $server = new swoole_websocket_server('0.0.0.0', 9502); // 动静分离配置 $server->set([ // 开启静态请求 'enable_static_handler' => true, // 静态资源目录 'document_root' => '/opt/app/swoole/websocket', ]); $server->on('open', function ($server, $request) { echo "server:handshake success with fd - {$request->fd}\n"; }); $server->on('message', function ($server, $frame) { echo "receive from {$frame->fd}:{$frame->data},opcode:{$frame->opcode},fin:{$frame->finish}\n"; $server->push($frame->fd, "this is server"); }); $server->on('close', function ($server, $fd) { echo "client - {$fd} - close\n"; }); $server->start();Copy after login
// 创建websocket实例 var websocketURL = "ws://www.rona1do.top:9502"; var websocket = new WebSocket(websocketURL); // 实例化对象的onopen属性 websocket.onopen = function (ev) { websocket.send("hello-websocket"); console.log("connect-swoole-success"); } // 实例化对象的onmessage属性,接收服务端返回的数据 websocket.onmessage = function (ev) { console.log("websockect-server-return-data:" + ev.data); } // close websocket.onclose = function (ev) { console.log("close"); }Copy after login
6. Use object-oriented to optimize websocket service code
class WebSocket {
const HOST = '0.0.0.0';
const PORT = 9502;
private $ws = null;
function __construct()
{
$this->ws = new swoole_websocket_server(self::HOST, self::PORT);
$this->ws->on('open', [$this, 'onOpen']);
$this->ws->on('message', [$this, 'onMessage']);
$this->ws->on('close', [$this, 'onClose']);
$this->ws->start();
}
// 监听websocket连接事件
function onOpen($server, $request) {
echo "server: handshake success with fd{$request->fd}\n";
}
// 监听websocket消息接收事件
function onMessage($server, $frame) {
echo "receive from {$frame->fd}:{$frame->data},opcode:{$frame->opcode},fin:{$frame->finish}\n";
$server->push($frame->fd, "this is server");
}
// 监听客户端关闭事件
function onClose($server, $fd) {
echo "Client:{$fd} closes\n";
}
}
Copy after login
class WebSocket { const HOST = '0.0.0.0'; const PORT = 9502; private $ws = null; function __construct() { $this->ws = new swoole_websocket_server(self::HOST, self::PORT); $this->ws->on('open', [$this, 'onOpen']); $this->ws->on('message', [$this, 'onMessage']); $this->ws->on('close', [$this, 'onClose']); $this->ws->start(); } // 监听websocket连接事件 function onOpen($server, $request) { echo "server: handshake success with fd{$request->fd}\n"; } // 监听websocket消息接收事件 function onMessage($server, $frame) { echo "receive from {$frame->fd}:{$frame->data},opcode:{$frame->opcode},fin:{$frame->finish}\n"; $server->push($frame->fd, "this is server"); } // 监听客户端关闭事件 function onClose($server, $fd) { echo "Client:{$fd} closes\n"; } }
7. Task in swoole Small case
onTask: is called within the task_worker process. The worker process can use the swoole_server_task function to deliver new tasks to the task_worker process. When the current Task process calls the onTask callback function, it will switch the process status to busy and will no longer receive new tasks. When the onTask function returns, it will switch the process status to idle and continue to receive new tasks.
onFinish: When the task delivered by the worker process is completed in task_worker, the task process will send the task processing result to the worker process through the swoole_server->finish() method.class Websocket { const HOST = '0.0.0.0'; const PORT = 9502; private $ws = null; public function __construct() { $this->ws = new swoole_websocket_server(self::HOST, self::PORT); $this->ws->set([ 'worker_num' => 2, 'task_worker_num' => 2, // 要想使用task必须要指明 ]); $this->ws->on('open', [$this, 'onOpen']); $this->ws->on('message', [$this, 'onMessage']); $this->ws->on('task', [$this, 'onTask']); $this->ws->on('finish', [$this, 'onFinish']); $this->ws->on('close', [$this, 'onClose']); $this->ws->start(); } public function onOpen($server, $request) { echo "server:handshake success with fd:{$request->fd}\n"; } public function onMessage($server, $frame) { echo "receive from {$frame->fd}:{$frame->data}\n"; // 需要投递的任务数据 $data = [ 'fd' => $frame->fd, 'msg' => 'task', ]; $server->task($data); $server->push($frame->fd, 'this is server'); } // 处理投递的任务方法,非阻塞 public function onTask($server, $task_id, $worker_id, $data) { print_r($data); // 模拟大量数据的操作 sleep(10); return "task_finish"; } // 投递任务处理完毕调用的方法 public function onFinish($server, $task_id, $data) { echo "task_id:{$task_id}\n"; echo "task finish success:{$data}\n"; } public function onClose($server, $fd) { echo "Client:close"; } }Copy after login
The above is the detailed content of Why did swoole go from getting started to giving up?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


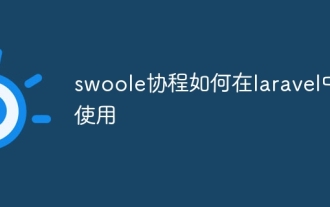
Using Swoole coroutines in Laravel can process a large number of requests concurrently. The advantages include: Concurrent processing: allows multiple requests to be processed at the same time. High performance: Based on the Linux epoll event mechanism, it processes requests efficiently. Low resource consumption: requires fewer server resources. Easy to integrate: Seamless integration with Laravel framework, simple to use.
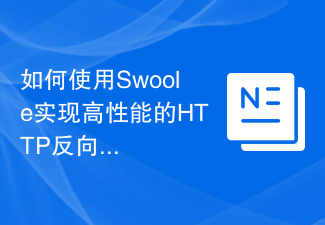
How to use Swoole to implement a high-performance HTTP reverse proxy server Swoole is a high-performance, asynchronous, and concurrent network communication framework based on the PHP language. It provides a series of network functions and can be used to implement HTTP servers, WebSocket servers, etc. In this article, we will introduce how to use Swoole to implement a high-performance HTTP reverse proxy server and provide specific code examples. Environment configuration First, we need to install the Swoole extension on the server
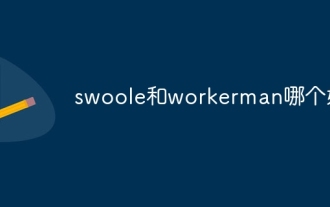
Swoole and Workerman are both high-performance PHP server frameworks. Known for its asynchronous processing, excellent performance, and scalability, Swoole is suitable for projects that need to handle a large number of concurrent requests and high throughput. Workerman offers the flexibility of both asynchronous and synchronous modes, with an intuitive API that is better suited for ease of use and projects that handle lower concurrency volumes.

Swoole Process allows users to switch. The specific steps are: create a process; set the process user; start the process.
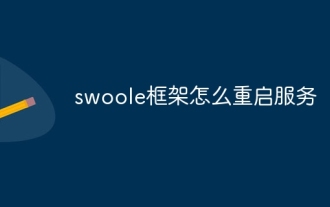
To restart the Swoole service, follow these steps: Check the service status and get the PID. Use "kill -15 PID" to stop the service. Restart the service using the same command that was used to start the service.
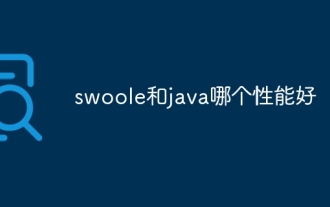
Performance comparison: Throughput: Swoole has higher throughput thanks to its coroutine mechanism. Latency: Swoole's coroutine context switching has lower overhead and smaller latency. Memory consumption: Swoole's coroutines occupy less memory. Ease of use: Swoole provides an easier-to-use concurrent programming API.
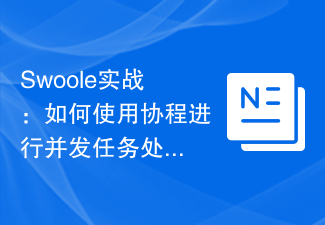
Swoole in action: How to use coroutines for concurrent task processing Introduction In daily development, we often encounter situations where we need to handle multiple tasks at the same time. The traditional processing method is to use multi-threads or multi-processes to achieve concurrent processing, but this method has certain problems in performance and resource consumption. As a scripting language, PHP usually cannot directly use multi-threading or multi-process methods to handle tasks. However, with the help of the Swoole coroutine library, we can use coroutines to achieve high-performance concurrent task processing. This article will introduce

Swoole is a high-performance PHP network development framework. With its powerful asynchronous mechanism and event-driven features, it can quickly build high-concurrency and high-throughput server applications. However, as the business continues to expand and the amount of concurrency increases, the CPU utilization of the server may become a bottleneck, affecting the performance and stability of the server. Therefore, in this article, we will introduce how to optimize the CPU utilization of the server while improving the performance and stability of the Swoole server, and provide specific optimization code examples. one,
