How to add request interceptor in uniapp
Uniapp method to add a request interceptor: 1. Define the LsxmRequest class and add the default configuration, interceptor and request method; 2. Subsequently, you need to customize the config and obtain the interface address, and add get and set methods to the class. ;3. Use the Symbol feature to define four private variables to prevent variable pollution.
The operating environment of this tutorial: windows7 system, uni-app2.5.1 version, DELL G3 computer. This method is suitable for all brands of computers.
Uniapp's method of adding a request interceptor:
1. Use the Symbol feature to define four private variables to prevent variable pollution
const config = Symbol('config') const isCompleteURL = Symbol('isCompleteURL') const requestBefore = Symbol('requestBefore') const requestAfter = Symbol('requestAfter')
2. Definition LsxmRequest class and add default configuration, interceptor and request method
class LsxmRequest { //默认配置 [config] = { baseURL: '', header: { 'content-type': 'application/json' }, method: 'GET', dataType: 'json', responseType: 'text' } //拦截器 interceptors = { request: (func) => { if (func) { LsxmRequest[requestBefore] = func } else { LsxmRequest[requestBefore] = (request) => request } }, response: (func) => { if (func) { LsxmRequest[requestAfter] = func } else { LsxmRequest[requestAfter] = (response) => response } } } static [requestBefore] (config) { return config } static [requestAfter] (response) { return response } static [isCompleteURL] (url) { return /(http|https):\/\/([\w.]+\/?)\S*/.test(url) } request (options = {}) { options.baseURL = options.baseURL || this[config].baseURL options.dataType = options.dataType || this[config].dataType options.url = LsxmRequest[isCompleteURL](options.url) ? options.url : (options.baseURL + options.url) options.data = options.data options.header = {...options.header, ...this[config].header} options.method = options.method || this[config].method options = {...options, ...LsxmRequest[requestBefore](options)} return new Promise((resolve, reject) => { options.success = function (res) { resolve(LsxmRequest[requestAfter](res)) } options.fail= function (err) { reject(LsxmRequest[requestAfter](err)) } uni.request(options) }) } get (url, data, options = {}) { options.url = url options.data = data options.method = 'GET' return this.request(options) } post (url, data, options = {}) { options.url = url options.data = data options.method = 'POST' return this.request(options) } }
3. Subsequently, you need to customize the config and obtain the interface address. Add get and set methods in the class:
setConfig (func) { this[config] = func(this[config]) } getConfig() { return this[config]; }
4. Use The custom plug-in registration method assigns the interface in apis.js (you need to import apis.js in main.js later) to the custom Vue prototype variable $lsxmApi. In order to avoid having to introduce it once on each page, in each page The beforeCreate life cycle of a page is mixed in.
LsxmRequest.install = function (Vue) { Vue.mixin({ beforeCreate: function () { if (this.$options.apis) { console.log(this.$options.apis) Vue._lsxmRequest = this.$options.apis } } }) Object.defineProperty(Vue.prototype, '$lsxmApi', { get: function () { return Vue._lsxmRequest.apis } }) } export default LsxmRequest
5. Instantiate and customize the request configuration items in config.js (add token in the header according to project needs) and interceptor
import LsxmRequest from './LsxmRequest' const lsxmRequest = new LsxmRequest() // 请求拦截器 lsxmRequest.interceptors.request((request) => { if (uni.getStorageSync('token')) { request.header['token'] = uni.getStorageSync('token'); } return request }) // 响应拦截器 lsxmRequest.interceptors.response((response) => { console.log('beforeRespone',response); // 超时重新登录 if(response.data.isOverTime){ uni.showModal({ title:'提示', content:'您已超时,请重新登录!', showCancel:false, icon:'success', success:function(e){ if(e.confirm){ uni.redirectTo({ url: '/pages/login/login' }) } } }); } else { return response; } }) // 设置默认配置 lsxmRequest.setConfig((config) => { config.baseURL = 'http://xxxxx.com' if (uni.getStorageSync('token')) { config.header['token'] = uni.getStorageSync('token'); } return config; }) export default lsxmRequest
6. In main.js Introduction, mount apis to Vue
import LsxmRequest from './service/LsxmRequest.js' import apis from './service/apis.js' import lsxmRequest from './service/config.js' Vue.use(LsxmRequest) Vue.prototype.baseDomain = lsxmRequest.getConfig().baseURL App.mpType = 'app' const app = new Vue({ store, apis, ...App }) app.$mount()
7. When you need to add an interface, you only need to add the interface in apis.js (the interface in apis.js can be split according to functions later, and the module Management)
import lsxmRequest from './config.js' export default{ apis:{ //获取验证用户令牌 getLoginToken(data){ return lsxmRequest.post('/xxx/xxx/getLoginToken', data) }, //登录 login(data){ return lsxmRequest.post('/xxx/xxx/login', data) } } }
8. At this point, you can use
this.$lsxmApi.getLoginToken({}).then((resToken) => { console.log(resToken) }
on the page to learn more about other high-quality articles, please pay attention touni-appColumn~
The above is the detailed content of How to add request interceptor in uniapp. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


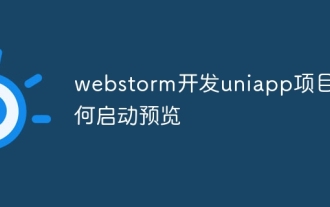
Steps to launch UniApp project preview in WebStorm: Install UniApp Development Tools plugin Connect to device settings WebSocket launch preview
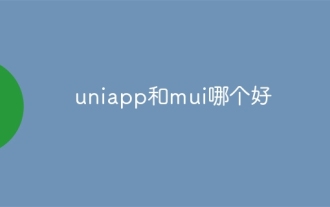
Generally speaking, uni-app is better when complex native functions are needed; MUI is better when simple or highly customized interfaces are needed. In addition, uni-app has: 1. Vue.js/JavaScript support; 2. Rich native components/API; 3. Good ecosystem. The disadvantages are: 1. Performance issues; 2. Difficulty in customizing the interface. MUI has: 1. Material Design support; 2. High flexibility; 3. Extensive component/theme library. The disadvantages are: 1. CSS dependency; 2. Does not provide native components; 3. Small ecosystem.
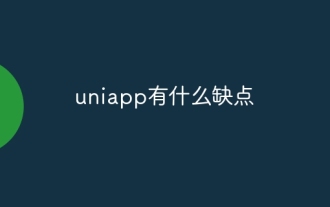
UniApp has many conveniences as a cross-platform development framework, but its shortcomings are also obvious: performance is limited by the hybrid development mode, resulting in poor opening speed, page rendering, and interactive response. The ecosystem is imperfect and there are few components and libraries in specific fields, which limits creativity and the realization of complex functions. Compatibility issues on different platforms are prone to style differences and inconsistent API support. The security mechanism of WebView is different from native applications, which may reduce application security. Application releases and updates that support multiple platforms at the same time require multiple compilations and packages, increasing development and maintenance costs.
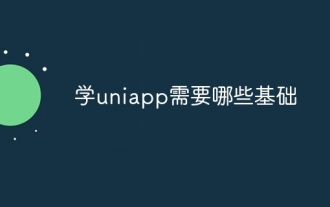
uniapp development requires the following foundations: front-end technology (HTML, CSS, JavaScript) mobile development knowledge (iOS and Android platforms) Node.js other foundations (version control tools, IDE, mobile development simulator or real machine debugging experience)
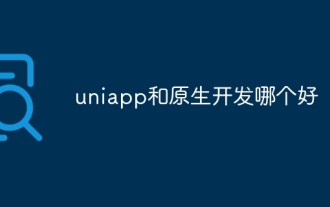
When choosing between UniApp and native development, you should consider development cost, performance, user experience, and flexibility. The advantages of UniApp are cross-platform development, rapid iteration, easy learning and built-in plug-ins, while native development is superior in performance, stability, native experience and scalability. Weigh the pros and cons based on specific project needs. UniApp is suitable for beginners, and native development is suitable for complex applications that pursue high performance and seamless experience.
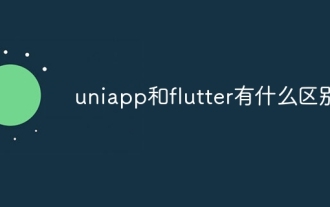
UniApp is based on Vue.js, and Flutter is based on Dart. Both support cross-platform development. UniApp provides rich components and easy development, but its performance is limited by WebView; Flutter uses a native rendering engine, which has excellent performance but is more difficult to develop. UniApp has an active Chinese community, and Flutter has a large and global community. UniApp is suitable for scenarios with rapid development and low performance requirements; Flutter is suitable for complex applications with high customization and high performance.
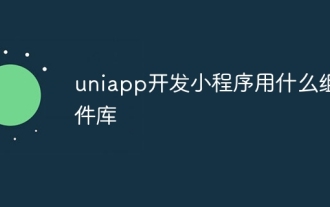
Recommended component library for uniapp to develop small programs: uni-ui: Officially produced by uni, it provides basic and business components. vant-weapp: Produced by Bytedance, with a simple and beautiful UI design. taro-ui: produced by JD.com and developed based on the Taro framework. fish-design: Produced by Baidu, using Material Design design style. naive-ui: Produced by Youzan, modern UI design, lightweight and easy to customize.
