Dom node vs element, what is the difference between the two?
Document Object Model (DOM) is an interface that treats HTML or XML documents as a tree structure, in which each Each node is an object of the document. DOM also provides a set of methods to query the tree, change structure, and style.
DOM also uses the term element (Element) which is very similar to a node. So, what is the difference between DOM nodes and elements?
1. DOM nodes
The key to understanding the difference between nodes and elements is to understand what a node is.
From a higher perspective, a DOM document consists of a node hierarchy. Each node can have parents and/or children.
Look at the following HTML document:
<!DOCTYPE html> <html> <head> <title>My Page</title> </head> <body> <!-- Page Body --> <h2 id="My-nbsp-Page">My Page</h2> <p id="content">Thank you for visiting my web page!</p> </body> </html>
The document contains the following node hierarchy:
## is a node in the document tree. It has 2 child nodes:
and
.
A node with 3 child nodes: Comment node
, Title
< h2>, paragraph
. The parent node of the
node is the
node.
The tag in the HTML document represents a node. Interestingly, ordinary text is also a node. Paragraph node has 1 child node: text node
"Thank you for visiting my web page!".
1.2 Node Type
How do we distinguish these different types of nodes? The answer lies in the DOM Node interface, specifically theNode.nodeType attribute.
Node.nodeType can have one of the following values representing the node type:
- Node.ELEMENT_NODENode.ATTRIBUTE_NODE
- Node.TEXT_NODENode.CDATA_SECTION_NODENode.PROCESSING_INSTRUCTION_NODENode.COMMENT_NODENode.DOCUMENT_NODENode. DOCUMENT_TYPE_NODENode.DOCUMENT_FRAGMENT_NODENode.NOTATION_NODE
Node.ELEMENT_NODE represents element nodes,
Node.TEXT_NODE represents the text node,
Node.DOCUMENT_NODE the document node, and so on.
nodeType attribute:
const paragraph = document.querySelector('p'); paragraph.nodeType === Node.ELEMENT_NODE; // => true
Node.DOCUMENT_NODE:
document.nodeType === Node.DOCUMENT_NODE; // => true
2. DOM elements
After mastering the knowledge of DOM nodes, it is now time to distinguish between DOM nodes and elements. If you understand node terminology, the answer is obvious: elements are nodes of a specific typeelement (Node.ELEMENT_NODE), as well as types such as document, comment, text, etc.
,
,
,
,
,
are all elements because they are represented by tags.
Node is the constructor of the node,
HTMLElement is Constructor for elements in JS DOM. A paragraph is both a node and an element, it is an instance of both
Node and
HTMLElement
const paragraph = document.querySelector('p'); paragraph instanceof Node; // => true paragraph instanceof HTMLElement; // => true
3. DOM attributes: nodes and elements
In addition to distinguishing between nodes and elements, it is also necessary to distinguish between DOM attributes that contain only nodes or only elements. The following properties of the node type evaluate to a node or collection of nodes (NodeList):
node.parentNode; // Node or null node.firstChild; // Node or null node.lastChild; // Node or null node.childNodes; // NodeList
HTMLCollection):
node.parentElement; // HTMLElement or null node.children; // HTMLCollection
node.childNodes and node.children return a list of children, why do you have both properties? good question!
<p> <b>Thank you</b> for visiting my web page! </p>
demo and look at the childNodes and
children# of the paragraph node ##Properties: <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>const paragraph = document.querySelector(&#39;p&#39;);
paragraph.childNodes; // NodeList: [HTMLElement, Text]
paragraph.children; // HTMLCollection: [HTMLElement]</pre><div class="contentsignin">Copy after login</div></div>paragraph.childNodes<p>The collection contains 2 nodes: <code><b>Thank you</b>
, and for visiting my web page!
Text node! However, the
collection contains only 1 item: <b>Thank you</b>
. Since
only contains elements, the text node is not included here because its type is text (Node.TEXT_NODE
), not element (Node.ELEMENT_NODE
). <p>Having both <code>node.childNodes
and node.children
, we can choose the set of children to access: all child nodes or only children that are elements.
4. Summary
A DOM document is a hierarchical collection of nodes, each node can have parents and/or children. Understanding the difference between DOM nodes and elements is easy if you understand what nodes are.
Nodes have types, and element types are one of them. Elements are represented by tags in HTML documents.
English original address: https://dmitripautin.com/dom-node-element/
Author: Shadeed
Source: dmitripavlutin
For more programming-related knowledge, please visit: Programming Teaching! !
The above is the detailed content of Dom node vs element, what is the difference between the two?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
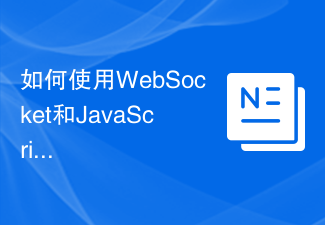
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
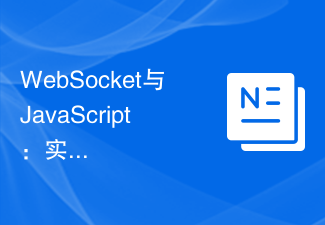
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
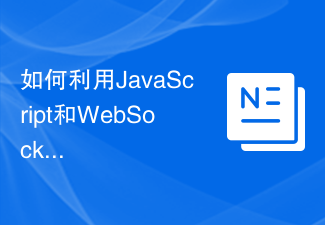
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
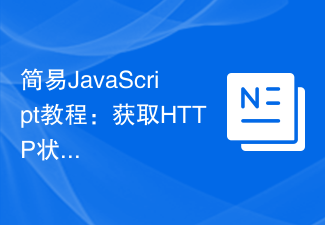
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
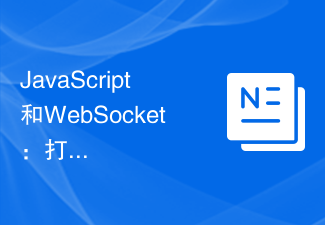
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
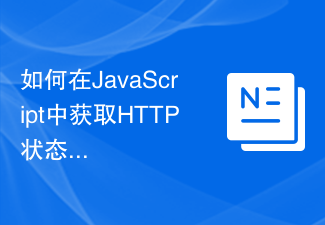
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
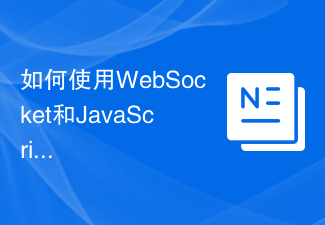
Real-time collaborative editors have become a standard feature of modern web development, especially in various team collaboration, online document editing and task management scenarios. Real-time communication technology based on WebSocket can improve communication efficiency and collaboration effects among team members. This article will introduce how to use WebSocket and JavaScript to build a simple online collaborative editor to help readers better understand the principles and usage of WebSocket. Understand the basic principles of WebSocketWebSo
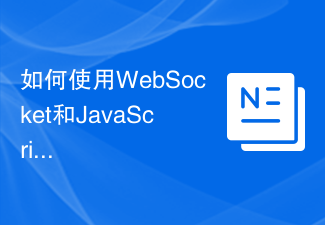
Overview of how to use WebSocket and JavaScript to implement an online electronic signature system: With the advent of the digital age, electronic signatures are widely used in various industries to replace traditional paper signatures. As a full-duplex communication protocol, WebSocket can perform real-time two-way data transmission with the server. Combined with JavaScript, an online electronic signature system can be implemented. This article will introduce how to use WebSocket and JavaScript to develop a simple online
