What are java static methods
In Java, static methods refer to member methods modified by static. A static method can be called without any instance of the class to which it belongs. Therefore, the this keyword cannot be used in a static method, and the instance variables and instance methods of the class to which it belongs cannot be directly accessed. However, the static variables of the class to which it belongs can be directly accessed. and static methods.
The operating environment of this tutorial: windows7 system, java8 version, DELL G3 computer.
Static methods (or class methods) refer to member methods modified by static.
The difference between static methods and instance methods:
Static methods do not need to be called through any instance of the class to which they belong, so in static methods You cannot use the this keyword, nor can you directly access the instance variables and instance methods of the class to which it belongs, but you can directly access the static variables and static methods of the class to which it belongs. In addition, like the this keyword, the super keyword is also related to a specific instance of the class, so the super keyword cannot be used in static methods.
In instance methods, you can directly access static variables, static methods, instance variables and instance methods of the class to which they belong.
Example:
Create a class with static variables, add several static methods to modify the values of the static variables, and then in main () method calls the static method and outputs the result.
public class StaticMethod { public static int count = 1; // 定义静态变量count public int method1() { // 实例方法method1 count++; // 访问静态变量count并赋值 System.out.println("在静态方法 method1()中的 count="+count); // 打印count return count; } public static int method2() { // 静态方法method2 count += count; // 访问静态变量count并赋值 System.out.println("在静态方法 method2()中的 count="+count); // 打印count return count; } public static void PrintCount() { // 静态方法PrintCount count += 2; System.out.println("在静态方法 PrintCount()中的 count="+count); // 打印count } public static void main(String[] args) { StaticMethod sft = new StaticMethod(); // 通过实例对象调用实例方法 System.out.println("method1() 方法返回值 intro1="+sft.method1()); // 直接调用静态方法 System.out.println("method2() 方法返回值 intro1="+method2()); // 通过类名调用静态方法,打印 count StaticMethod.PrintCount(); } }
The result after running this program is as follows:
在静态方法 method1()中的 count=2 method1() 方法返回值 intro1=2 在静态方法 method2()中的 count=4 method2() 方法返回值 intro1=4 在静态方法 PrintCount()中的 count=6
In this program, the static variable count is used as shared data between instances, so count is called in different methods, and the value are different. As can be seen from this program, the non-static method method1() cannot be called in the static methods method1() and PrintCount(), but the static methods method2() and PrintCount() can be called in the method1() method.
When accessing non-static methods, you need to access them through instance objects. When accessing static methods, you can access them directly, through class names, or through instantiated objects.
Recommended related video tutorials: Java video tutorial
The above is the detailed content of What are java static methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


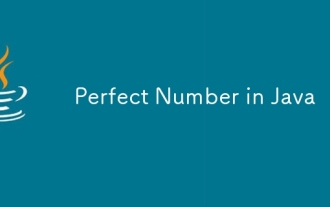
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
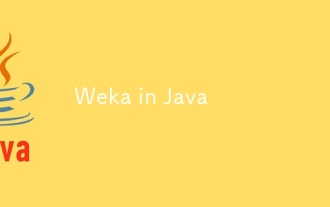
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
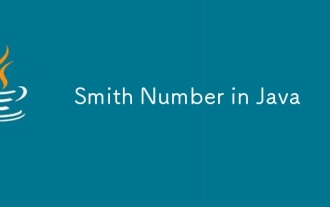
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
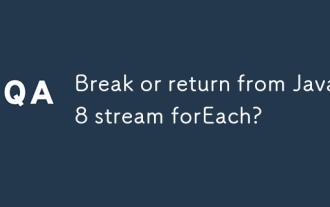
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
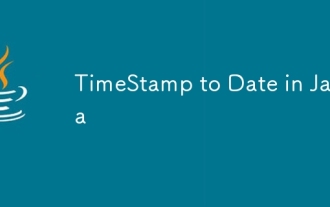
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
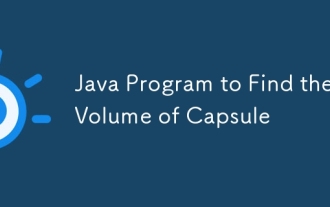
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
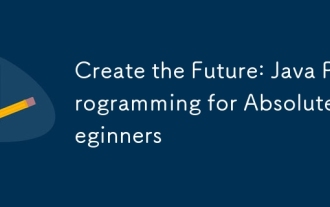
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
