


How does JavaScript handle parallel requests? Brief analysis of four methods
This article will let you know how JavaScript handles parallel requests? Introducing the four ways in which JS handles parallel requests, I hope it will be helpful to everyone!
Requirements
Two asynchronous requests are issued at the same time, and processing will be done when both requests return
Implementation
The method here only provides ideas and only handles successful request processing
Method 1
Use Promise.all
const startTime = new Date().getTime() function request(time) { return new Promise(resolve => { setTimeout(() => { resolve(time) }, time) }) } let request1 = request(3000) let request2 = request(2000) Promise.all([request1, request2]).then(res => { console.log(res, new Date() - startTime) // [ 3000, 2000 ] 3001 })
Method 2
Customize the status, judge the return status in the callback, and wait until both requests have return values before processing
const startTime = new Date().getTime() function request(time) { return new Promise(resolve => { setTimeout(() => { resolve(time) }, time) }) } let state = [undefined, undefined] let request1 = request(3000) let request2 = request(2000) request1.then(res => { state[0] = res process() }) request2.then(res => { state[1] = res process() }) function process() { if (state[0] && state[1]) { console.log(state, new Date() - startTime) // [ 3000, 2000 ] 3001 } }
Method 3
generator, yield
const startTime = new Date().getTime() function ajax(time, cb) { setTimeout(() => cb(time), time) } function request(time) { ajax(time, data => { it.next(data); }) } function* main() { let request1 = request(3000); let request2 = request(2000); let res1 = yield request1 let res2 = yield request2 console.log(res1, res2, new Date() - startTime) // 2000 3000 3001 } let it = main(); it.next();
There is something wrong with this place, Because request2 takes a short time, it will return first,
that is, execute it.next(2000) first, causing res1 to obtain the return value of request2
If you use the co function, this problem will not exist , because co executes it.next() in the promise.then function, which is equivalent to it.next() being a chain call
generator uses the co function
const co = require('co') const startTime = new Date().getTime() function request (time) { return new Promise(resolve => { setTimeout(() => { resolve(time) }, time) }) } co(function* () { let request1 = request(3000); let request2 = request(2000); let res1 = yield request1 let res2 = yield request2 console.log(res1, res2, new Date() - startTime) // 3000 2000 3001 })
With the co function, there is no need to generate it and execute the next method; The principle of co is actually simple, that is, recursively execute next until done is true; If the value returned by next is a Promise, execute next in the then function. If it is not a Promise, execute the next function directly. The following is a simplified handwritten implementation of the co function
function co(func) { let it = func() let t = it.next() next() function next() { if (t.done) return if (t.value instanceof Promise) { t.value.then(res => { t = it.next(res) next() }) } else { t = it.next(t.value) next() } } }
Method 4
With generator, it is easy to think of async/await, after all async/ Await is implemented by generator
// setTimeout模拟异步请求,time为请求耗时 const startTime = new Date().getTime() function request (time) { return new Promise(resolve => { setTimeout(() => { resolve(time) }, time) }) } (async function () { let request1 = request(3000) let request2 = request(2000) let res1 = await request1 console.log(res1, new Date() - startTime) // 3000 3001 let res2 = await request2 console.log(res2, new Date() - startTime) // 2000 3005 })()
For more programming-related knowledge, please visit:Programming Video! !
The above is the detailed content of How does JavaScript handle parallel requests? Brief analysis of four methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


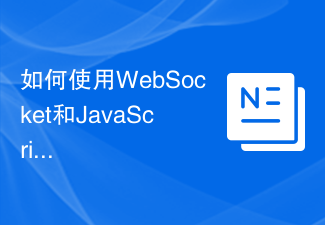
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
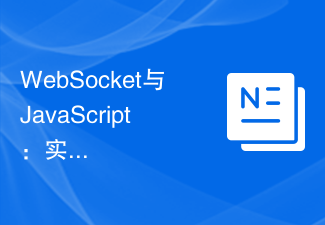
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
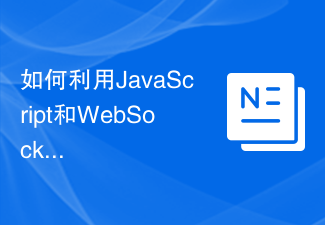
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
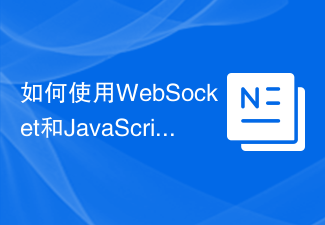
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
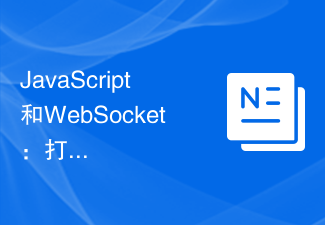
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
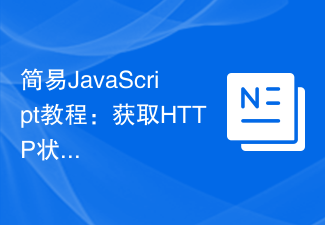
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
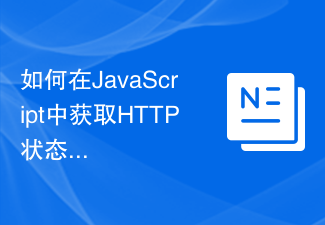
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
