


A brief discussion on how to maintain separation of concerns using Angular instructions?
How to maintain separation of concerns using Angular directives? The following article will introduce to you how to maintain separation of concerns through Angular instructions. I hope it will be helpful to you!
Suppose we have a date picker component in our application. Every time the user changes the date, an event is sent to the analytics provider. So far, we have only used it once, so this analysis interface can be placed in the component that uses it: [Related tutorial recommendation: "angular tutorial"]
header- 1.ts
import { UntilDestroy, untilDestroyed } from '@ngneat/until-destroyed'; @UntilDestroy() class FooComponent { timespanControl = new FormControl(); ngOnInit() { this.timespanControl.valueChanges .pipe(untilDestroyed(this)) .subscribe(({ preset }) => { this.analyticsService.track('timespan-filter apply', { value: preset, }); }); } }
However, now that we have more places to use this analysis interface, we don’t want to write the same code repeatedly. Someone might suggest that this code could be incorporated into a date picker and passed as an input parameter.
data-picker-1.component.ts
class DatePickerComponent { @Input() analyticsContext: string; constructor(private analyticsService: AnalyticsService) {} apply() { this.analyticsService.track('timespan-filter apply', { context: this.analyticsContext, value: this.preset, }); ... } }
Indeed, this can be achieved, but this is not an ideal design. Separation of concerns means that the date picker itself has nothing to do with the analysis interface, and it does not need to know any information about the analysis interface.
In addition, because the date picker is an internal component, we can modify its source code, but what if it is a third-party component? How to solve it?
A better choice here is the Angular directive. Create a directive, obtain a reference to the form through DI, and subscribe to changes in internal values to trigger analysis events. datePickerAnalytics.directive.ts
@UntilDestroy() @Directive({ selector: '[datePickerAnalytics]', }) export class DatePickerAnalyticsDirective implements OnInit { @Input('datePickerAnalytics') analyticsContext: string; constructor( private dateFormControl: NgControl, private analyticsService: AnalyticsService ) {} ngOnInit() { this.dateFormControl .control.valueChanges.pipe(untilDestroyed(this)) .subscribe(({ preset }) => { this.analyticsService.track( 'timespan-filter apply', { value: preset, context: this.analyticsContext } ); }); } }
It can now be used every time the date picker is used.
<date-picker [formControl]="control" datePickerAnalytics="fooPage"></date-picker>
For more programming-related knowledge, please visit: Programming Video! !
The above is the detailed content of A brief discussion on how to maintain separation of concerns using Angular instructions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


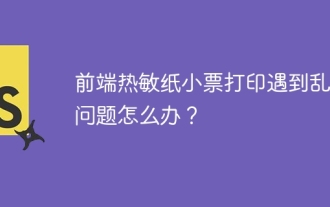
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
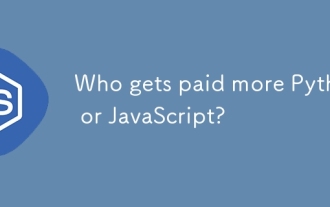
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
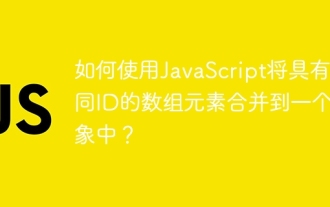
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
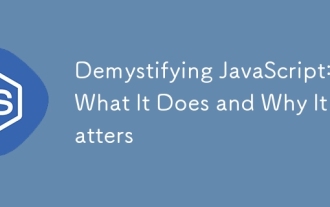
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
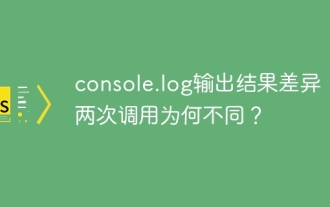
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
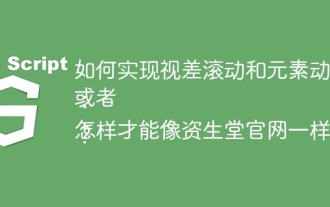
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
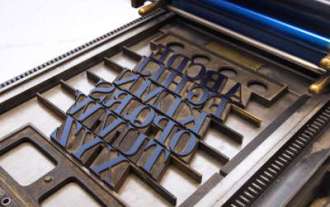
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
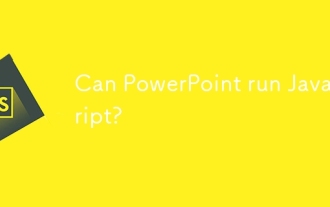
JavaScript can be run in PowerPoint, and can be implemented by calling external JavaScript files or embedding HTML files through VBA. 1. To use VBA to call JavaScript files, you need to enable macros and have VBA programming knowledge. 2. Embed HTML files containing JavaScript, which are simple and easy to use but are subject to security restrictions. Advantages include extended functions and flexibility, while disadvantages involve security, compatibility and complexity. In practice, attention should be paid to security, compatibility, performance and user experience.
