How to call methods in js files in vuejs
vuejs calls the method in the js file: 1. Create a new js file under the assets file; 2. Use "export default {...}" to reference it in the component that needs to use the method.
The operating environment of this article: Windows7 system, Vue2.9.6 version, DELL G3 computer
How does vuejs call the method in the js file ?
How to reference js files in vue
In many components of vue, axios is used to post data, and each component is written with a The post method is also possible, just copy it, but it always feels a bit inconvenient. Wouldn't it be better to write the axios post into a separate js file and then reference it in the required components?
1. Create a new js file under the assets file
Create a new file named webpost.js
import axios from 'axios' //Post方法的封装 function axiosPost(url,params){ return new Promise((resolve, reject) => { axios({ url: url, method: 'post', data: params, headers: { 'Content-Type':'application/json' } }) .then(res=>{ resolve(res.data); }); }); } export { axiosPost }
This needs to specifically quote axios, which is the first line. Then you can use it. The last sentence is very important, otherwise you will not be able to call
in other components. 2. Reference
<script> import {axiosPost} from '../assets/webpost'; export default { }
in the component that needs to use this method. Pay attention to the path of the reference. The content in import {} is the content in the export above.
When using it, you don’t even need this, just axiosPost.
axiosPost(url,params) .then(res=>{ if (res===401){ this.$message.error('哦,对不起,你所输入的用户名或密码有误!'); }else{ }
3. Another way to write js
The following is the re-edited part. In the past few days, I have sorted out the axios part and added an interceptor to bring token verification when requesting the api. There is only one more export, and you can write multiple. The structure is clearer and easier to understand.
import axios from 'axios' //Post方法的封装 export function axiosPost(url,params){ return new Promise((resolve, reject) => { //以下部分是拦截器功能 axios.interceptors.request.use(config=>{ const token=localStorage.getItem('token') if(token){ config.headers.authorization=token } return config },err=>{ }) //下面是正常的 axios({ url: url, method: 'post', data: params, headers: { 'Content-Type':'application/json' } }) .then(res=>{ resolve(res.data); }); }); }
Recommended: "The latest 5 vue.js video tutorial selections"
The above is the detailed content of How to call methods in js files in vuejs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


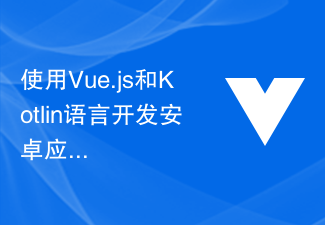
Some tips for developing Android applications using Vue.js and Kotlin language. With the popularity of mobile applications and the continuous growth of user needs, the development of Android applications has attracted more and more attention from developers. When developing Android apps, choosing the right technology stack is crucial. In recent years, Vue.js and Kotlin languages have gradually become popular choices for Android application development. This article will introduce some techniques for developing Android applications using Vue.js and Kotlin language, and give corresponding code examples. 1. Set up the development environment at the beginning
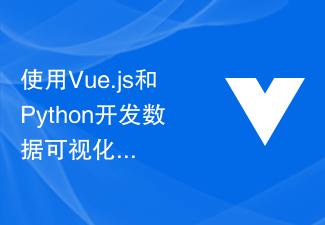
Some tips for developing data visualization applications using Vue.js and Python Introduction: With the advent of the big data era, data visualization has become an important solution. In the development of data visualization applications, the combination of Vue.js and Python can provide flexibility and powerful functions. This article will share some tips for developing data visualization applications using Vue.js and Python, and attach corresponding code examples. 1. Introduction to Vue.js Vue.js is a lightweight JavaScript
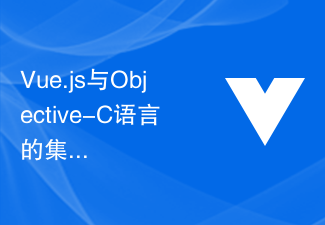
Integration of Vue.js and Objective-C language, tips and suggestions for developing reliable Mac applications. In recent years, with the popularity of Vue.js in front-end development and the stability of Objective-C in Mac application development, developers Start trying to combine the two to develop more reliable and efficient Mac applications. This article will introduce some tips and suggestions to help developers correctly integrate Vue.js and Objective-C and develop high-quality Mac applications. one
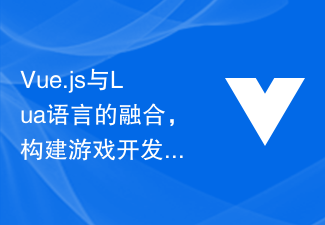
The integration of Vue.js and Lua language, best practices and experience sharing for building a front-end engine for game development Introduction: With the continuous development of game development, the choice of game front-end engine has become an important decision. Among these choices, the Vue.js framework and Lua language have become the focus of many developers. As a popular front-end framework, Vue.js has a rich ecosystem and convenient development methods, while the Lua language is widely used in game development because of its lightweight and efficient performance. This article will explore how to
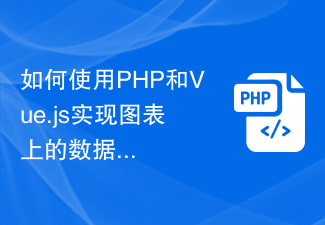
How to use PHP and Vue.js to implement data filtering and sorting functions on charts. In web development, charts are a very common way of displaying data. Using PHP and Vue.js, you can easily implement data filtering and sorting functions on charts, allowing users to customize the viewing of data on charts, improving data visualization and user experience. First, we need to prepare a set of data for the chart to use. Suppose we have a data table that contains three columns: name, age, and grades. The data is as follows: Name, Age, Grades Zhang San 1890 Li
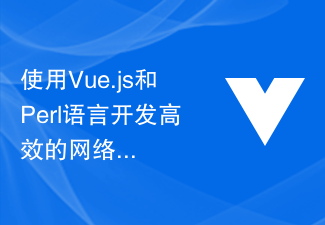
Use Vue.js and Perl languages to develop efficient web crawlers and data scraping tools. In recent years, with the rapid development of the Internet and the increasing importance of data, the demand for web crawlers and data scraping tools has also increased. In this context, it is a good choice to combine Vue.js and Perl language to develop efficient web crawlers and data scraping tools. This article will introduce how to develop such a tool using Vue.js and Perl language, and attach corresponding code examples. 1. Introduction to Vue.js and Perl language
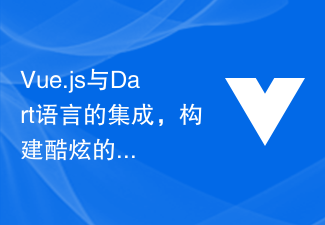
Integration of Vue.js and Dart language, practice and development skills for building cool mobile application UI interfaces Introduction: In mobile application development, the design and implementation of the user interface (UI) is a very important part. In order to achieve a cool mobile application interface, we can integrate Vue.js with the Dart language, and use the powerful data binding and componentization features of Vue.js and the rich mobile application development library of the Dart language to build Stunning mobile application UI interface. This article will show you how to
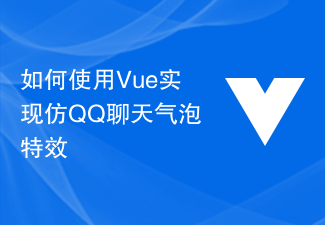
How to use Vue to implement QQ-like chat bubble effects In today’s social era, the chat function has become one of the core functions of mobile applications and web applications. One of the most common elements in the chat interface is the chat bubble, which can clearly distinguish the sender's and receiver's messages, effectively improving the readability of the message. This article will introduce how to use Vue to implement QQ-like chat bubble effects and provide specific code examples. First, we need to create a Vue component to represent the chat bubble. The component consists of two main parts
