What is javascript function
In JavaScript, function refers to "function", which is a set of code blocks that perform specific tasks (with specific functions) and can be reused; functions do not run automatically and need to be called through the function name. to run. Functions can be stored in variables, objects, arrays, and passed as parameters to other functions.
The operating environment of this tutorial: windows7 system, javascript version 1.8.5, Dell G3 computer.
JS function (Function) is a set of code blocks that perform specific tasks (with specific functions) and can be reused; functions do not run automatically and need to be called through the function name to run.
Functions can also be stored in variables, objects, and arrays, and functions can also be passed as parameters to other functions, or returned from other functions.
In JavaScript, in addition to using built-in functions, we can also create our own functions (custom functions) and then call this function where needed. This not only avoids writing repeated code, but also benefits the code. later maintenance.
JS Define functions
JS function declaration needs to start with the function keyword, followed by the name of the function to be created. Use a space to separate the function keyword and the function name. , after the function name is a bracket (), the brackets are used to define the parameters to be used in the function (use commas to separate multiple parameters), a function can have up to 255 parameters, and finally a curly bracket { }, the function body in curly braces is used to define the function body (that is, the code to implement the function), as shown below:
function functionName(parameter_list) { // 函数中的代码 }
The sample code is as follows:
function sayHello(name){ document.write("Hello " + name); }
In the above example, a function sayHello is defined (), this function needs to receive a parameter name, and calling this function will output "Hello..." on the page.
JS Calling Function
Once a function is defined, we can call it anywhere in the current document. Calling a function is very simple, just add a bracket after the function name, such as alert(), write(). Note that if parameters are specified in parentheses after the function name when defining the function, then the corresponding parameters need to be provided in parentheses when calling the function.
The sample code is as follows:
function sayHello(name){ document.write("Hello " + name); } // 调用 sayHello() 函数 sayHello('PHP中文网');
Tip: JavaScript is case-sensitive, so the function keyword must be lowercase when defining a function, and the function must be called in the same size as when it was declared. Write to call the function.
Default values of parameters
When defining a function, you can set a default value for the parameters of the function, so that when we call this function, if it is not provided parameter, this default value will be used as the parameter value, as shown in the following example:
function sayHello(name = "World"){ document.write("Hello " + name); } sayHello(); // 输出:Hello World sayHello('PHP中文网'); // 输出:Hello PHP中文网
JS function return value
In the function, you can use the return statement to return a value (The running result of the function) is returned to the program that called the function. This value can be of any type, such as arrays, objects, strings, etc. For functions that return a value, we can use a variable to receive the return value of the function. The sample code is as follows:
function getSum(num1, num2){ return num1 + num2; } var sum1 = getSum(7, 12); // 函数返回值为:19 var sum2 = getSum(-5, 33); // 函数返回值为:28
Tip: The return statement is usually defined at the end of the function. When the function runs to the return statement It will stop running immediately and return to the place where the function was called to continue execution.
In addition, a function can only have one return value. If you want to return multiple values, you can put the values into an array and then return the array, as shown in the following example:
function division(dividend, divisor){ var quotient = dividend / divisor; var arr = [dividend, divisor, quotient] return arr; } var res = division(100, 4) document.write(res[0]); // 输出:100 document.write(res[1]); // 输出:4 document.write(res[2]); // 输出:25
JS function expression
Function expression is very similar to declaring variables. It is another form of declaring a function. The syntax format is as follows:
var myfunction = function name(parameter_list){ // 函数中的代码 };
Parameters The description is as follows:
myfunction: variable name, which can be used to call the function after the equal sign;
name: function name, which can be omitted (Under normal circumstances we will also omit it), if omitted, the function will become an anonymous function;
parameter_list: is the parameter list, a function can have up to 255 parameters .
The sample code is as follows:
// 函数声明 function getSum(num1, num2) { var total = num1 + num2; return total; } // 函数表达式 var getSum = function(num1, num2) { var total = num1 + num2; return total; };
The two functions in the above example are equivalent, and their functions, return values, and calling methods are the same.
Note: In a function declaration, there is no need to place a semicolon after the right curly brace, but if a function expression is used, it should end with a semicolon at the end of the expression.
Although function declarations and function expressions look very similar, they operate differently, as shown in the following example:
declaration(); // 输出: function declaration function declaration() { document.write("function declaration"); } expression(); // 报错:Uncaught TypeError: undefined is not a function var expression = function() { document.write("function expression"); };
As shown in the above example, if the function expression If the formula is called before it is defined, an exception (error) will be thrown, but the function declaration can run successfully. This is because JavaScript will parse the function declaration before the program is executed, so it is feasible to call the function before or after the function declaration. A function expression assigns an anonymous function to a variable, so before the program executes the expression, it is equivalent to the function not being defined and therefore cannot be called.
[Recommended learning: javascript advanced tutorial]
The above is the detailed content of What is javascript function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


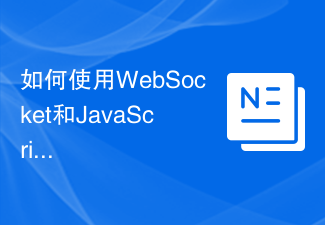
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
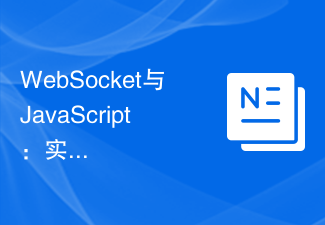
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
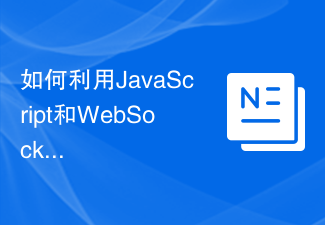
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
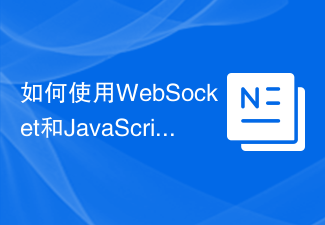
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
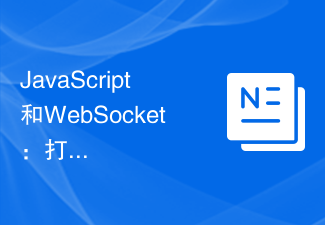
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
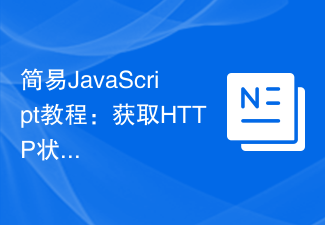
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
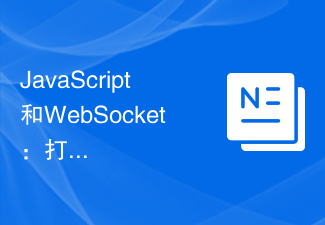
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
