


Detailed explanation of how Laravel uses multiple databases (with code examples)
The following tutorial column of Laravel will introduce you to the method of using multiple databases in Laravel. I hope it will be helpful to you!
Use .env
>= 5.0 (Tested based on 5.5 Laravel 8 and also available)
In .env
File
DB_CONNECTION=mysqlDB_HOST=127.0.0.1DB_PORT=3306DB_DATABASE=database1DB_USERNAME=rootDB_PASSWORD=secretDB_CONNECTION_SECOND=mysqlDB_HOST_SECOND=127.0.0.1DB_PORT_SECOND=3306DB_DATABASE_SECOND=database2DB_USERNAME_SECOND=rootDB_PASSWORD_SECOND=secret
In config/database.php
File
'mysql' => [ 'driver' => env('DB_CONNECTION'), 'host' => env('DB_HOST'), 'port' => env('DB_PORT'), 'database' => env('DB_DATABASE'), 'username' => env('DB_USERNAME'), 'password' => env('DB_PASSWORD'),],'mysql2' => [ 'driver' => env('DB_CONNECTION_SECOND'), 'host' => env('DB_HOST_SECOND'), 'port' => env('DB_PORT_SECOND'), 'database' => env('DB_DATABASE_SECOND'), 'username' => env('DB_USERNAME_SECOND'), 'password' => env('DB_PASSWORD_SECOND'),],
Note: In
mysql2
, ifdb_username
anddb_password
are the same, then you can useenv('DB_USERNAME')
.
Mode
To specify which connection to use, just use the connection()
method
Schema::connection('mysql2')->create('some_table', function($table){ $table->increments('id'):});
Query Producer
$users = DB::connection('mysql2')->select(...);
Model
Set the $connection
variable in the model.
class SomeModel extends Eloquent { protected $connection = 'mysql2';}
You can also define the connection at runtime through the setConnection
method or the on
static method:
class SomeController extends BaseController { public function someMethod() { $someModel = new SomeModel; $someModel->setConnection('mysql2'); // non-static method $something = $someModel->find(1); $something = SomeModel::on('mysql2')->find(1); // static method return $something; }}
Note Be careful when trying to establish relationships with tables that span databases! This can be used, but it may come with some caveats and depends on the database and database setup you have.
From Laravel Docs
Using multiple database connections
When using multiple connections , you can access each connection through the connection method on the DB
facade class. The name passed to the connection
method should correspond to a connection listed in the config/database.php
configuration file:
$users = DB::connection('foo')->select(...);
You can also access the original underlying PDO instance using the getPdo
method on the connection instance:
$pdo = DB::connection()->getPdo();
Original address: https://stackoverflow.com/questions/ 31847054/how-to-use-multiple-databases-in-laravel
Translation address: https://learnku.com/laravel/t/62110
The above is the detailed content of Detailed explanation of how Laravel uses multiple databases (with code examples). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


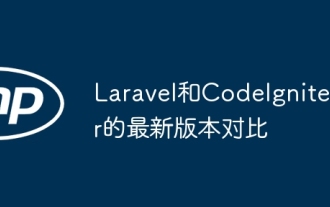
The latest versions of Laravel 9 and CodeIgniter 4 provide updated features and improvements. Laravel9 adopts MVC architecture and provides functions such as database migration, authentication and template engine. CodeIgniter4 uses HMVC architecture to provide routing, ORM and caching. In terms of performance, Laravel9's service provider-based design pattern and CodeIgniter4's lightweight framework give it excellent performance. In practical applications, Laravel9 is suitable for complex projects that require flexibility and powerful functions, while CodeIgniter4 is suitable for rapid development and small applications.
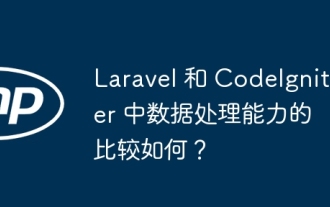
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
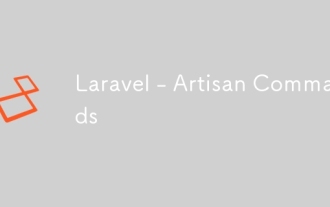
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
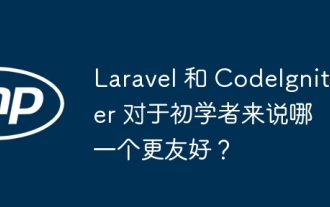
For beginners, CodeIgniter has a gentler learning curve and fewer features, but covers basic needs. Laravel offers a wider feature set but has a slightly steeper learning curve. In terms of performance, both Laravel and CodeIgniter perform well. Laravel has more extensive documentation and active community support, while CodeIgniter is simpler, lightweight, and has strong security features. In the practical case of building a blogging application, Laravel's EloquentORM simplifies data manipulation, while CodeIgniter requires more manual configuration.
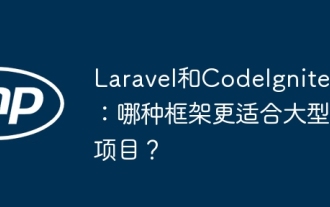
When choosing a framework for large projects, Laravel and CodeIgniter each have their own advantages. Laravel is designed for enterprise-level applications, offering modular design, dependency injection, and a powerful feature set. CodeIgniter is a lightweight framework more suitable for small to medium-sized projects, emphasizing speed and ease of use. For large projects with complex requirements and a large number of users, Laravel's power and scalability are more suitable. For simple projects or situations with limited resources, CodeIgniter's lightweight and rapid development capabilities are more ideal.
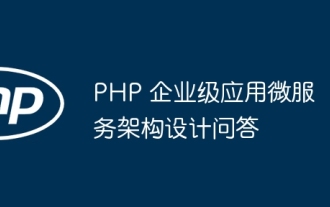
Microservice architecture uses PHP frameworks (such as Symfony and Laravel) to implement microservices and follows RESTful principles and standard data formats to design APIs. Microservices communicate via message queues, HTTP requests, or gRPC, and use tools such as Prometheus and ELKStack for monitoring and troubleshooting.
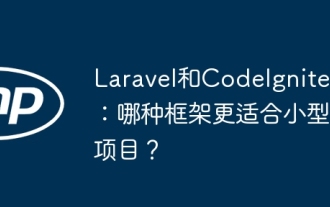
For small projects, Laravel is suitable for larger projects that require strong functionality and security. CodeIgniter is suitable for very small projects that require lightweight and ease of use.
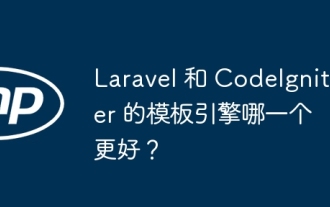
Comparing Laravel's Blade and CodeIgniter's Twig template engine, choose based on project needs and personal preferences: Blade is based on MVC syntax, which encourages good code organization and template inheritance. Twig is a third-party library that provides flexible syntax, powerful filters, extended support, and security sandboxing.
