A brief discussion on how to use dynamic components in Vue?
本文文章我们来了解一下Vue中的组件,介绍一下动态组件的用法,希望对大家有所帮助!
动态组件在开发的过程中大多数情况下都会用到,当我们需要在不同的组件之间进行状态切换时,动态组件可以很好的满足我们的需求,其中的核心是component
标签和is
属性的使用。【相关推荐:《vue.js教程》】
<div id="app"> <button @click="changeTabs('child1')">child1</button> <button @click="changeTabs('child2')">child2</button> <button @click="changeTabs('child3')">child3</button> <component :is="chooseTabs"> </component> </div>
// js var child1 = { template: '<div>content1</div>', } var child2 = { template: '<div>content2</div>' } var child3 = { template: '<div>content3</div>' } var vm = new Vue({ el: '#app', components: { child1, child2, child3 }, methods: { changeTabs(tab) { this.chooseTabs = tab; } } })
例子是一个动态组件的基本使用场景,当点击按钮时,视图根据this.chooseTabs
值在组件child1,child2,child3
间切换。
AST解析
<component>
的解读和前面几篇内容一致,会从AST
解析阶段说起,过程也不会专注每一个细节,而是把和以往处理方式不同的地方特别说明。针对动态组件解析的差异,集中在processComponent
上,由于标签上is
属性的存在,它会在最终的ast
树上打上component
属性的标志。
// 针对动态组件的解析 function processComponent (el) { var binding; // 拿到is属性所对应的值 if ((binding = getBindingAttr(el, 'is'))) { // ast树上多了component的属性 el.component = binding; } if (getAndRemoveAttr(el, 'inline-template') != null) { el.inlineTemplate = true; } }
render函数
有了ast
树,接下来是根据ast
树生成可执行的render
函数,由于有component
属性,render
函数的产生过程会走genComponent
分支。
// render函数生成函数 var code = generate(ast, options); // generate函数的实现 function generate (ast,options) { var state = new CodegenState(options); var code = ast ? genElement(ast, state) : '_c("div")'; return { render: ("with(this){return " + code + "}"), staticRenderFns: state.staticRenderFns } } function genElement(el, state) { ··· var code; // 动态组件分支 if (el.component) { code = genComponent(el.component, el, state); } }
针对动态组件的处理逻辑其实很简单,当没有内联模板标志时(后面会讲),拿到后续的子节点进行拼接,和普通组件唯一的区别在于,_c
的第一个参数不再是一个指定的字符串,而是一个代表组件的变量
// 针对动态组件的处理 function genComponent ( componentName, el, state ) { // 拥有inlineTemplate属性时,children为null var children = el.inlineTemplate ? null : genChildren(el, state, true); return ("_c(" + componentName + "," + (genData$2(el, state)) + (children ? ("," + children) : '') + ")") }
普通组件和动态组件的对比
"with(this){return _c('div',{attrs:{"id":"app"}},[_c('child1',[_v(_s(test))])],1)}"
动态组件的render函数
with(this){return _c('div',{attrs:{"id":"app"}},[_c(chooseTabs,{tag:"component"})],1)}
简单的总结,动态组件和普通组件的区别在于:
ast
阶段新增了component
属性,这是动态组件的标志产生
render
函数阶段由于component
属性的存在,会执行genComponent
分支,genComponent
会针对动态组件的执行函数进行特殊的处理,和普通组件不同的是,_c
的第一个参数不再是不变的字符串,而是指定的组件名变量。render
到vnode
阶段和普通组件的流程相同,只是字符串换成了变量,并有{ tag: 'component' }
的data
属性。例子中chooseTabs
此时取的是child1
。
有了render
函数,接下来从vnode到真实节点的过程和普通组件在流程和思路上基本一致,这一阶段可以回顾之前介绍组件流程的分析
更多编程相关知识,请访问:编程入门!!
The above is the detailed content of A brief discussion on how to use dynamic components in Vue?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
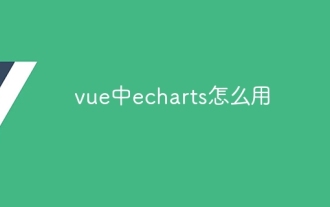
Using ECharts in Vue makes it easy to add data visualization capabilities to your application. Specific steps include: installing ECharts and Vue ECharts packages, introducing ECharts, creating chart components, configuring options, using chart components, making charts responsive to Vue data, adding interactive features, and using advanced usage.
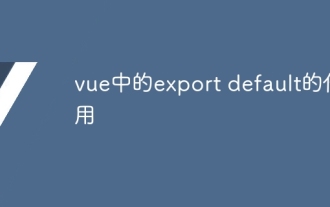
Question: What is the role of export default in Vue? Detailed description: export default defines the default export of the component. When importing, components are automatically imported. Simplify the import process, improve clarity and prevent conflicts. Commonly used for exporting individual components, using both named and default exports, and registering global components.
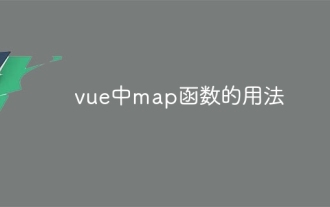
The Vue.js map function is a built-in higher-order function that creates a new array where each element is the transformed result of each element in the original array. The syntax is map(callbackFn), where callbackFn receives each element in the array as the first argument, optionally the index as the second argument, and returns a value. The map function does not change the original array.
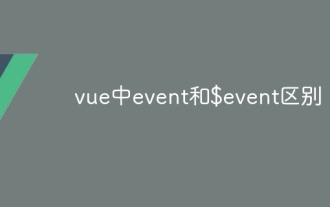
In Vue.js, event is a native JavaScript event triggered by the browser, while $event is a Vue-specific abstract event object used in Vue components. It is generally more convenient to use $event because it is formatted and enhanced to support data binding. Use event when you need to access specific functionality of the native event object.
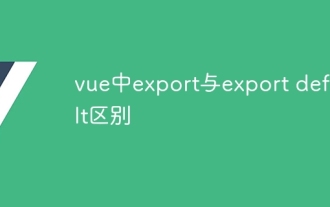
There are two ways to export modules in Vue.js: export and export default. export is used to export named entities and requires the use of curly braces; export default is used to export default entities and does not require curly braces. When importing, entities exported by export need to use their names, while entities exported by export default can be used implicitly. It is recommended to use export default for modules that need to be imported multiple times, and use export for modules that are only exported once.
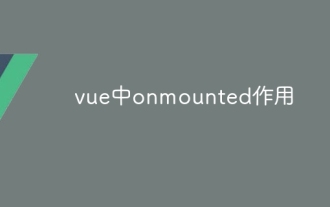
onMounted is a component mounting life cycle hook in Vue. Its function is to perform initialization operations after the component is mounted to the DOM, such as obtaining references to DOM elements, setting data, sending HTTP requests, registering event listeners, etc. It is only called once when the component is mounted. If you need to perform operations after the component is updated or before it is destroyed, you can use other lifecycle hooks.
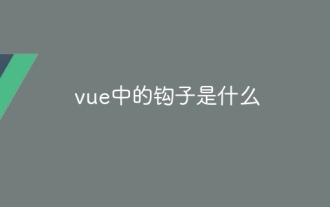
Vue hooks are callback functions that perform actions on specific events or lifecycle stages. They include life cycle hooks (such as beforeCreate, mounted, beforeDestroy), event handling hooks (such as click, input, keydown) and custom hooks. Hooks enhance component control, respond to component life cycles, handle user interactions and improve component reusability. To use hooks, just define the hook function, execute the logic and return an optional value.
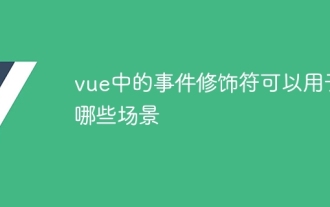
Vue.js event modifiers are used to add specific behaviors, including: preventing default behavior (.prevent) stopping event bubbling (.stop) one-time event (.once) capturing event (.capture) passive event listening (.passive) Adaptive modifier (.self)Key modifier (.key)
