What is the use of laravel facade?
In laravel, facades are used to provide a static interface for the classes of the application's IoC service container. Laravel's facade serves as a static proxy for the underlying classes in the service container. Compared with traditional static methods, Provides easier-to-test syntax during maintenance.
The operating environment of this tutorial: Windows 10 system, Laravel 6 version, DELL G3 computer.
What is the use of laravel facade
Introduction
Facades provides a static interface for the application's IoC service container class. Laravel comes with some Facades, such as Cache, etc. Laravel's facade serves as a "static proxy" for the underlying class in the service container. Compared with traditional static methods, it can provide easier to test, more flexible, concise and elegant syntax during maintenance.
Explanation
In the context of Laravel application, a Facade is a class. Using this class, you can access an object from the container. This function is in the Facade defined in the class. Laravel's Facades and any Facades you define yourself will inherit the Facade class.
Your Facade class only needs to implement one method: getFacadeAccessor. Whatever needs to be resolved in the container is done in this method. The Facade base class uses the __callStatic() magic method, which can delay calls from Facade to the resolved object.
So, when you use Facade to call, like this: Cache:get, laravel will resolve the cache management class from the Ioc service container, and then call the get method on this class. Laravel's Facades can be used to locate services, which is a more convenient syntax for using Laravel's IoC service container.
Advantages
Facade has many advantages. It provides a simple and easy-to-remember syntax, allowing us to use Laravel provided without having to remember long class names. Functional features, in addition, make them easy to test due to their unique use of PHP's dynamic methods.
Actual use
The following example is used to call Laravel's cache system. Take a look at the following line of code first. You may think that this is a direct call to a static method called get on the Cache class.
1 |
|
However, if you look at the Illuminate\Support\Facades\Cache class, you will find that there is no get static method at all:
1 2 3 4 5 6 7 8 |
|
The Cache class inherits the Facade base class , which defines a method called getFacadeAccessor(). Note that what this method does is to return an Ioc binding name, here it is cache.
When the user references any static method on the Cache Facade, Laravel will resolve the cache binding from the Ioc service container and execute the requested method on the object. (Here is the get method).
So, when we call Cache::get, what it really means is this:
1 |
|
Import Facades
Note, When using facade, if a namespace is used in the controller, you need to import the Facade class into this namespace. All Facades are under the global namespace:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
Create Facades
You only need three things to create a Facade:
An IoC binding.
A Facade class.
Configuration of a Facade alias.
Below we define a class: PaymentGateway\Payment.
1 2 3 4 5 6 7 |
|
We need to be able to resolve this class in the Ioc service container. Therefore, first add a Service Provider binding:
1 2 3 4 |
|
The best way to register this binding is to create a new Service Provider, name it PaymentServiceProvider, and then bind it to register method. Then configure laravel and load your Service Provider in the configuration file config/app.php.
The next step is to create your own Facade class:
1 2 3 4 5 6 |
|
Finally, if you like, you can add an alias to the Facade and put it in the aliases array in the config/app.php configuration file inside.
You can call the process method on an instance of the Payment class. Like this:
1 |
|
[Related recommendations: laravel video tutorial]
The above is the detailed content of What is the use of laravel facade?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




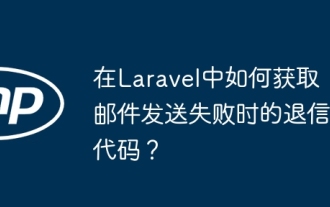
Method for obtaining the return code when Laravel email sending fails. When using Laravel to develop applications, you often encounter situations where you need to send verification codes. And in reality...
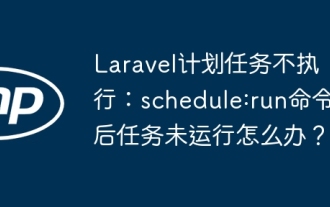
Laravel schedule task run unresponsive troubleshooting When using Laravel's schedule task scheduling, many developers will encounter this problem: schedule:run...
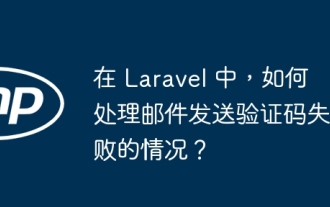
The method of handling Laravel's email failure to send verification code is to use Laravel...
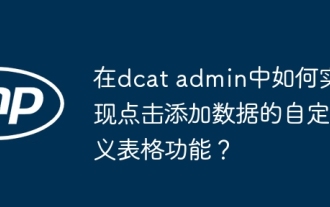
How to implement the table function of custom click to add data in dcatadmin (laravel-admin) When using dcat...
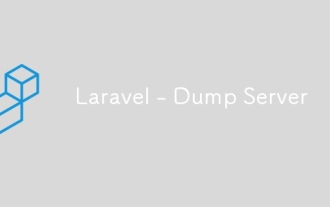
Laravel - Dump Server - Laravel dump server comes with the version of Laravel 5.7. The previous versions do not include any dump server. Dump server will be a development dependency in laravel/laravel composer file.
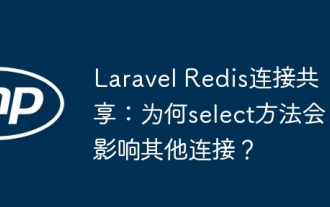
The impact of sharing of Redis connections in Laravel framework and select methods When using Laravel framework and Redis, developers may encounter a problem: through configuration...
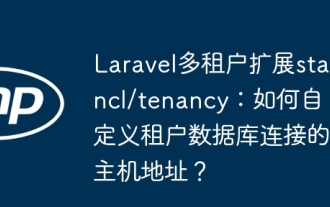
Custom tenant database connection in Laravel multi-tenant extension package stancl/tenancy When building multi-tenant applications using Laravel multi-tenant extension package stancl/tenancy,...

Laravel - Action URL - Laravel 5.7 introduces a new feature called “callable action URL”. This feature is similar to the one in Laravel 5.6 which accepts string in action method. The main purpose of the new syntax introduced Laravel 5.7 is to directl
