There are several modes for React routing
React routing has two modes, namely: 1. Hash mode, which adds a "#" sign before the path to become a hash value; 2. History mode, which allows the operation of the browser. Session history that has been accessed in tabs or frames.
The operating environment of this tutorial: Windows 10 system, react17.0.1 version, Dell G3 computer.
React routing
1. What is
In a single-page application, a web project has only one html page. Once the page is loaded, there is no need to reload or jump the page due to user operations. Its features are as follows:
Change the URL and prevent the browser from sending a request to the server
In Dynamically change the URL address in the browser address bar without refreshing the page
It is mainly divided into two modes:
hash mode: add after the url #, such as http://127.0.0.1:5500/home/#/page1
- ##history mode: allows the operation of the browser's session history that has been accessed in tabs or frames
2. Use
The components corresponding to the hash mode and history mode of React Router are:HashRouterBrowserRouterThe use of these two components is very simple, as the top-level component wraps other components, as shown below
// 1.import { BrowserRouter as Router } from "react-router-dom"; // 2.import { HashRouter as Router } from "react-router-dom"; import React from 'react'; import { BrowserRouter as Router, // HashRouter as Router Switch, Route, } from "react-router-dom"; import Home from './pages/Home'; import Login from './pages/Login'; import Backend from './pages/Backend'; import Admin from './pages/Admin'; function App() { return ( <Router> <Route path="/login" component={Login}/> <Route path="/backend" component={Backend}/> <Route path="/admin" component={Admin}/> <Route path="/" component={Home}/> </Router> ); } export default App;
3. Implementation Principle
Routing describes the mapping relationship between URL and UI. This mapping is one-way, that is, URL changes cause UI updates (no need to refresh the page)The following uses hash mode as an example. Changing the hash value will not cause the browser to send a request to the server. If the browser does not send a request, it will not refresh the page. Hash value changes trigger global The hashchange event on the window object. Therefore, hash mode routing uses the hashchange event to monitor changes in the URL, thereby performing DOM operations to simulate page jumpsreact-router also implements route jumps based on this featureThe following uses the HashRouter component Analysis and expansion:HashRouter
HashRouter wraps the entire application, monitors the hash value through window.addEventListener('hashChange', callback) Change and pass it to its nested componentsThen pass the location data to the descendant components through context, as follows:import React, { Component } from 'react'; import { Provider } from './context' // 该组件下Api提供给子组件使用 class HashRouter extends Component { constructor() { super() this.state = { location: { pathname: window.location.hash.slice(1) || '/' } } } // url路径变化 改变location componentDidMount() { window.location.hash = window.location.hash || '/' window.addEventListener('hashchange', () => { this.setState({ location: { ...this.state.location, pathname: window.location.hash.slice(1) || '/' } }, () => console.log(this.state.location)) }) } render() { let value = { location: this.state.location } return ( <Provider value={value}> { this.props.children } </Provider> ); } } export default HashRouter;
Router
Router What the component mainly does is to match the current value passed through BrowserRouter, the path passed in through props and the pathname passed in context, and then decide whether to execute the rendering componentimport React, { Component } from 'react'; import { Consumer } from './context' const { pathToRegexp } = require("path-to-regexp"); class Route extends Component { render() { return ( <Consumer> { state => { console.log(state) let {path, component: Component} = this.props let pathname = state.location.pathname let reg = pathToRegexp(path, [], {end: false}) // 判断当前path是否包含pathname if(pathname.match(reg)) { return <Component></Component> } return null } } </Consumer> ); } } export default Route;
react video Tutorial》
The above is the detailed content of There are several modes for React routing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
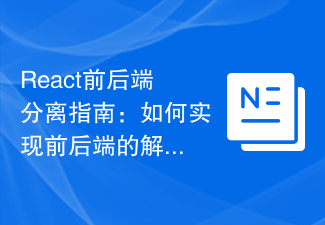
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
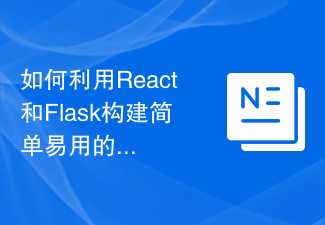
How to use React and Flask to build simple and easy-to-use web applications Introduction: With the development of the Internet, the needs of web applications are becoming more and more diverse and complex. In order to meet user requirements for ease of use and performance, it is becoming increasingly important to use modern technology stacks to build network applications. React and Flask are two very popular frameworks for front-end and back-end development, and they work well together to build simple and easy-to-use web applications. This article will detail how to leverage React and Flask
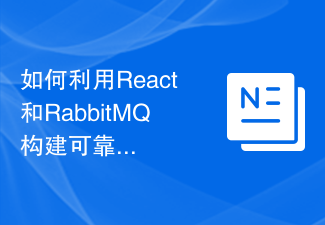
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
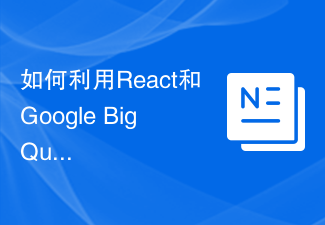
How to use React and Google BigQuery to build fast data analysis applications Introduction: In today's era of information explosion, data analysis has become an indispensable link in various industries. Among them, building fast and efficient data analysis applications has become the goal pursued by many companies and individuals. This article will introduce how to use React and Google BigQuery to build a fast data analysis application, and provide detailed code examples. 1. Overview React is a tool for building
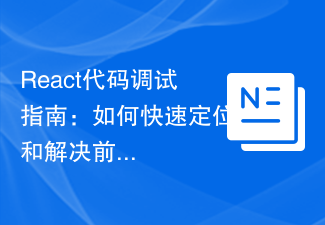
React code debugging guide: How to quickly locate and resolve front-end bugs Introduction: When developing React applications, you often encounter a variety of bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications. 1. Selection of debugging tools: In Re
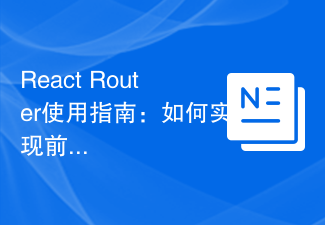
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
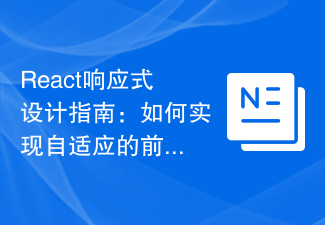
React Responsive Design Guide: How to Achieve Adaptive Front-end Layout Effects With the popularity of mobile devices and the increasing user demand for multi-screen experiences, responsive design has become one of the important considerations in modern front-end development. React, as one of the most popular front-end frameworks at present, provides a wealth of tools and components to help developers achieve adaptive layout effects. This article will share some guidelines and tips on implementing responsive design using React, and provide specific code examples for reference. Fle using React
