How to implement hooks in react? Do I have to rely on Fiber?
reactHow to implement hooks? Does the implementation of React Hooks have to rely on Fiber? The following article will show you how hooks in different frameworks are implemented. I hope it will be helpful to you!
React’s hooks are features that appeared after fiber, so many people mistakenly believe that hooks must rely on fiber to be implemented. In fact, this is not the case. There is no necessary connection between the two. . [Related recommendations: Redis Video Tutorial]
Now, hooks are not only implemented in react, but also in frameworks such as preact, react ssr, midway, etc., but their implementation is not Depends on fiber.
Let’s take a look at how the hooks in these different frameworks are implemented:
react How to implement hooks
react is described through jsx interface, it will be compiled into a render function by compilation tools such as babel or tsc, and then executed to generate vdom:
The render function here was React.createElement before React17:
Changed to jsx after React 17:
This jsx-runtime will be introduced automatically, no need to do it like before Each component must retain a React import.
Render function execution generates vdom:
The structure of vdom is like this:
In Before React16, this vdom would be rendered recursively, adding, deleting, and modifying the real dom.
After React16 introduced the fiber architecture, there was an additional step: first convert vdom to fiber, and then render the fiber.
The process of converting vdom to fiber is called reconcile, and the final process of adding, deleting, or modifying the real dom is called commit.
Why do we need to do this conversion?
Because vdom only has references to the child nodes children, and does not have references to the parent node parent and other sibling nodes, this results in the need to recursively render all vdom nodes to the dom at one time without interrupting.
What will happen if it is interrupted? Because the parent node and sibling nodes are not recorded, we can only continue to process the child nodes, but cannot process other parts of the vdom.
That’s why React introduced this kind of fiber structure, that is, there are references such as parent node return, child node child, sibling node sibling, etc., which can be interrupted, because if it is broken and then restored, all unprocessed items can be found. past node.
The structure of the fiber node is like this:
This process can be interrupted, and naturally it can be scheduled, which is the schdule process.
So the fiber architecture is divided into three stages: schdule, reconcile (convert vdom to fiber), and commit (update to dom).
Hooks can be used in function components to access some values, which are stored on fiber nodes.
For example, 6 hooks are used in this function component:
Then the corresponding fiber node has a memorizedState linked list of 6 elements:
Concatenated through next:
Different hooks access values on different elements of the memorizedState linked list, this is react The principle of hooks.
This linked list has a creation phase and an update phase, so you will find that the final implementation of useXxx is divided into mountXxx and updateXxx:
The mount phase here is Create hook nodes and assemble them into a linked list:
#The created hook linked list will be linked to the memorizedState attribute of the fiber node.
When updating, you can naturally take out the hook list from the fiber node:
In this way, in multiple renderings, the api of useXxx can be on the fiber node Find the corresponding memorizedState on .
This is the principle of react hooks. You can see that it stores hooks on fiber nodes.
What is the difference between preact?
preact How to implement hooks
preact is a more lightweight framework compatible with react code. It supports class components and function components, as well as react features such as hooks. . However, it does not implement the fiber architecture.
Because it mainly considers the ultimate size (only 3kb), not the ultimate performance.
We just learned that react stores the hook list on the fiber node. If preact does not have a fiber node, where will the hook list be stored?
In fact, it is easy to think that fiber just modified vdom to improve performance, and there is no essential difference from vdom. Then can it be enough to store the hook on vdom?
Indeed, preact puts the hook list on vdom.
For example, this function component has 4 hooks:
Its implementation is to access the corresponding hook on vdom:
It does not divide the hook into two stages, mount and update, like react, but merges them together for processing.
As shown in the figure, it stores hooks in the array of component.__hooks and accesses them through subscripts.
This component is an attribute on vdom:
That is, the value of hooks is stored in the array of vnode._component._hooks.
Compare the differences between react and preact in implementing hooks:
In react, the hook list is stored in the fiberNode.memorizedState property, while in preact, the hook list is stored in On the vnode._component._hooks attribute
the hook list in react is concatenated through next, and the hook list in preact is an array, accessed through subscripts
-
react separates the creation and update of the hook linked list, that is, useXxx will be divided into mountXxx and updateXxx to implement, while preact merges them together to handle
So, The implementation of hooks does not rely on fiber. It just finds a place to store the hook data corresponding to the component. It only needs to be retrieved during rendering. It does not matter where it is stored.
Because vdom and fiber are strongly related to component rendering, they are stored in these structures.
Like react ssr to implement hooks, they neither exist on fiber nor vdom:
How does react ssr implement hooks
In fact, react In addition to csr, the -dom package can also do ssr: Use the render method of react-dom when
csr: Use react when
ssr -The renderToString method or renderToStream method of dom/server:
Do you think vdom to fiber conversion will be done during ssr?
Definitely not. Fiber is a structure introduced to improve the rendering performance when running in the browser, make calculations interruptible, and perform calculations when idle.
Server-side rendering naturally does not require fiber.
If fiber is not needed, where does it store the hook list? vdom?
It can indeed be placed in vdom, but it is not.
For example, useRef hooks:
It is a linked list concatenated with next starting from firstWorkInProgressHook.
The first hook node created by firstWorkInProgressHook using createHook:
is not mounted to vdom superior.
why?
Because ssr only needs to be rendered once and does not need to be updated, there is no need to hang it on vdom.
Just clear the hook list every time you finish processing the hooks of each component:
So, when react ssr, hooks exist in global variables.
Compare the differences in the implementation principles of hooks in react csr and ssr:
csr will create fibers from vdom to make rendering interruptible , improve performance through idle scheduling, but not in ssr. vdom directly renders
hooks are saved to the fiber node in csr, and directly placed in global variables in ssr On, each component will be cleared after processing. Because it will not be used a second time
csr will divide the creation and update of the hook into two phases: mount and update, while ssr will only be processed once and only has the creation phase
#The implementation principle of hooks is actually not complicated. It is to store a linked list in a certain context, and then the hooks api accesses the corresponding data from different elements of the linked list to complete their respective logic. . This context can be a vdom, fiber or even a global variable.
However, the idea of hooks is still quite popular. The server-side framework midway produced by Taobao has introduced the idea of hooks:
midway How to implement hooks
midway is a Node.js framework:
The server-side framework naturally does not have such structures as vdom and fiber, but the idea of hooks does not rely on these. To implement the hooks API, you only need to put a linked list in a certain context.
midway has implemented an api similar to react hooks:
I don’t know where the hook list exists yet. Look, we have already mastered the implementation principle of hooks. As long as there is a context to store the hook list, it can be anywhere.
Summary
react hooks are a feature that emerged after the react fiber architecture. Many people mistakenly believe that hooks must be implemented with fiber. We have looked at react, preact, and The implementation of hooks in react ssr and midway found that this is not the case:
- react converts vdom into fiber, then stores the hook list on the fiber.memorizedState attribute, and concatenates it through next
- preact does not implement fiber. It places the hook list on the vnode._component._hooks attribute and implements it as an array. When accessing
- react ssr through subscripts, fiber is not required, but the hook is not implemented. The linked list is hung on vdom, but placed directly on a global variable, because it only needs to be rendered once, and the global variable can be cleared after rendering a component.
- midway is a Node.js framework, it also implements We haven’t gone into details about APIs similar to hooks, but as long as there is a context to store the hook list, it will be fine.
So, do react hooks have to rely on fiber to implement it?
Obviously not, it can be paired with fiber, vdom, global variables, or even any context.
For more programming related knowledge, please visit: Programming Video! !
The above is the detailed content of How to implement hooks in react? Do I have to rely on Fiber?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics





How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
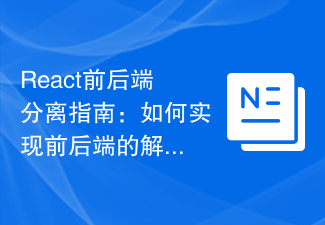
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
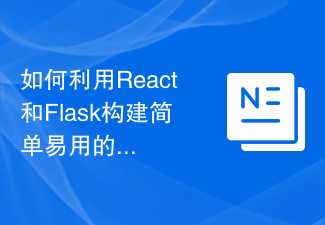
How to use React and Flask to build simple and easy-to-use web applications Introduction: With the development of the Internet, the needs of web applications are becoming more and more diverse and complex. In order to meet user requirements for ease of use and performance, it is becoming increasingly important to use modern technology stacks to build network applications. React and Flask are two very popular frameworks for front-end and back-end development, and they work well together to build simple and easy-to-use web applications. This article will detail how to leverage React and Flask
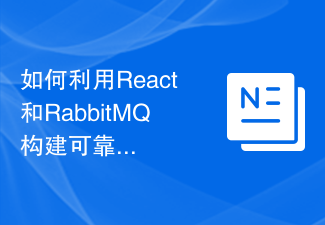
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
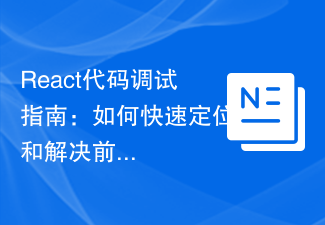
React code debugging guide: How to quickly locate and resolve front-end bugs Introduction: When developing React applications, you often encounter a variety of bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications. 1. Selection of debugging tools: In Re
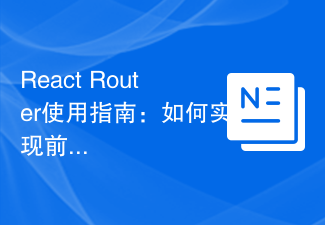
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
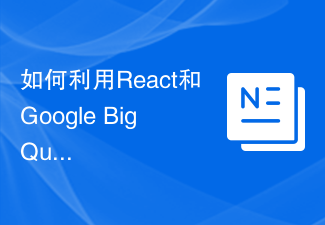
How to use React and Google BigQuery to build fast data analysis applications Introduction: In today's era of information explosion, data analysis has become an indispensable link in various industries. Among them, building fast and efficient data analysis applications has become the goal pursued by many companies and individuals. This article will introduce how to use React and Google BigQuery to build a fast data analysis application, and provide detailed code examples. 1. Overview React is a tool for building

How to use React and Apache Kafka to build real-time data processing applications Introduction: With the rise of big data and real-time data processing, building real-time data processing applications has become the pursuit of many developers. The combination of React, a popular front-end framework, and Apache Kafka, a high-performance distributed messaging system, can help us build real-time data processing applications. This article will introduce how to use React and Apache Kafka to build real-time data processing applications, and
