What does react-dom do?
The function of "react-dom" is to render the virtual DOM into the document and turn it into the actual DOM; "react-dom" is a toolkit that needs to be used when developing react projects. It provides DOM-specific methods, which can Used at the top level of the application, it can also be used as an interface for special DOM manipulation outside of the React model.
The operating environment of this tutorial: Windows 10 system, react17.0.1 version, Dell G3 computer.
What is the role of react-dom
When using react to develop a web page, two packages will be downloaded, one is react and the other is react-dom, where the react package is the core code of react. React-dom is the part related to DOM operations stripped out of React.
The core idea of react is virtual DOM. React includes the function react.createElement that generates virtual DOM, and the Component class. When we encapsulate components ourselves, we need to inherit the Component class to use life cycle functions, etc. The core function of the react-dom package is to render these virtual DOMs into documents and turn them into actual DOMs.
react-dom is a toolkit that needs to be used when developing react projects. It is a platform implementation for dom, mainly used for rendering on the web side. The react-dom package provides DOM-specific methods that can be used at the top level of the application or as an interface for special operations on the DOM outside of the React model.
react-dom mainly contains three APIs: findDOMNode, unmountComponentAtNode and render. The following is introduced in order of triggering.
1. render
render is used to render the virtual DOM rendered by React to the browser DOM, and is generally used in top-level components. This method mounts the element into the container and returns the instance of element (that is, the refs reference). If it is a stateless component, render will return null. When the component is loaded, the callback will be called. The syntax is:
render(ReactElement element,DOMElement container,[function callback])
For example:
import React from 'react' import ReactDOM from 'react-dom' import Router from './router' import { Provider } from 'react-redux' import store from './store' // Provider react-redux的内容 ReactDOM.render( <Provider store={store}> <Router/> </Provider>, document.getElementById('root'))
2, findDOMNode
findDOMNode is used to obtain the real DOM element in order to operate on the DOM node .
Before this, you must first know: In React, the virtual DOM is actually added to HTML and converted into a real DOM after the component is mounted (render()), so we can use componentDidMount and componentDidUpdate. Obtained in two ways. An example is as follows:
import { findDOMNode } from 'react-dom'; <Example ref={ node=>{ this.node = node} }> // 利用ref获取Example组件的实例 const dom = findDOMNode(this.node); // 通过findDOMNode获取实例对应的真实DOM
Note: When it comes to complex operations, there are many element DOM APIs available. However, DOM operations will have a great impact on performance, so DOM operations should be minimized.
3. unmountComponentAtNode
unmountComponentAtNode is used to perform unmount operations and is executed before componentWillUnmount.
Recommended learning: "react video tutorial"
The above is the detailed content of What does react-dom do?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
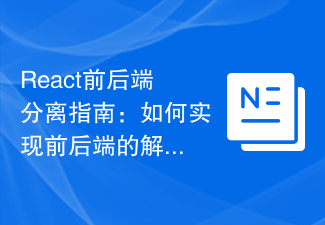
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
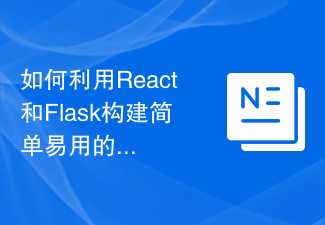
How to use React and Flask to build simple and easy-to-use web applications Introduction: With the development of the Internet, the needs of web applications are becoming more and more diverse and complex. In order to meet user requirements for ease of use and performance, it is becoming increasingly important to use modern technology stacks to build network applications. React and Flask are two very popular frameworks for front-end and back-end development, and they work well together to build simple and easy-to-use web applications. This article will detail how to leverage React and Flask
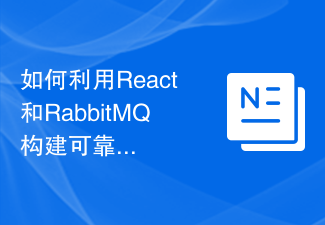
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
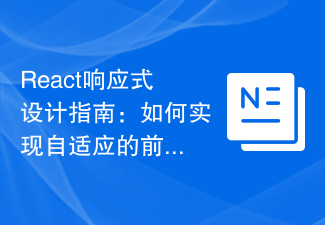
React Responsive Design Guide: How to Achieve Adaptive Front-end Layout Effects With the popularity of mobile devices and the increasing user demand for multi-screen experiences, responsive design has become one of the important considerations in modern front-end development. React, as one of the most popular front-end frameworks at present, provides a wealth of tools and components to help developers achieve adaptive layout effects. This article will share some guidelines and tips on implementing responsive design using React, and provide specific code examples for reference. Fle using React
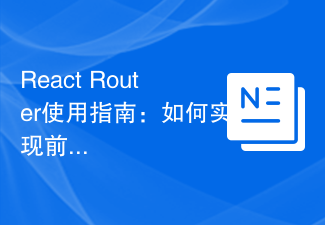
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
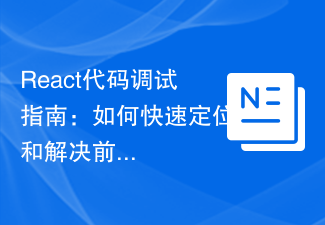
React code debugging guide: How to quickly locate and resolve front-end bugs Introduction: When developing React applications, you often encounter a variety of bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications. 1. Selection of debugging tools: In Re
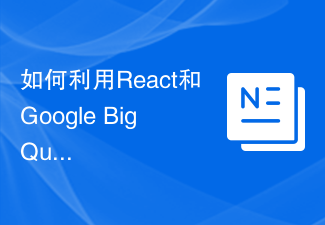
How to use React and Google BigQuery to build fast data analysis applications Introduction: In today's era of information explosion, data analysis has become an indispensable link in various industries. Among them, building fast and efficient data analysis applications has become the goal pursued by many companies and individuals. This article will introduce how to use React and Google BigQuery to build a fast data analysis application, and provide detailed code examples. 1. Overview React is a tool for building
