How to implement array traversal in es6
Method: 1. Use the "array.keys()" method, which can traverse the array index; 2. Use the "array.values()" method, which can traverse the array elements; 3. Use " Array.entries()" method, which can traverse the index and elements of the array.
The operating environment of this tutorial: Windows 10 system, ECMAScript version 6.0, Dell G3 computer.
How to implement array traversal in es6
ES6 provides the entries(), keys(), and values() methods to return the iterator of the array. For the iterator (Iterator), you can use for.. .of for convenience, or you can use the Iterator.next() method of the iterator returned by entries() to traverse.
1. Use keys() to traverse.
keys() returns a traverser of array element index numbers.
const arr1 = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k'] for (let index of arr1.keys()) { console.log(index); }
Result:
You can see that the output is the index of each array element.
0 1 2 3 4 5 6 7 8 9 10
2. Use values() to traverse.
values() returns a traverser of array element values.
for (let val of arr1.values()) { console.log(val); }
Result:
a b c d e f g h i j k
3. Use entries() to traverse.
Used with destructuring, you can get the index and value of the element.
for (let [index, val] of arr1.entries()) { console.log(index, val); }
Result:
0 'a' 1 'b' 2 'c' 3 'd' 4 'e' 5 'f' 6 'g' 7 'h' 8 'i' 9 'j' 10 'k'
4. Use Iterator.next() to traverse.
Based on the traverser returned by entries(), calling the next() method of the traverser can obtain the access entry of each element. The entry has a done attribute to indicate whether it is convenient to end. You can get the value attribute through the entrance, which is the index of the element and the array of values.
let arrEntries=arr1.entries(); let entry=arrEntries.next(); while(!entry.done){ console.log(entry.value); entry=arrEntries.next(); }
Result:
[ 0, 'a' ] [ 1, 'b' ] [ 2, 'c' ] [ 3, 'd' ] [ 4, 'e' ] [ 5, 'f' ] [ 6, 'g' ] [ 7, 'h' ] [ 8, 'i' ] [ 9, 'j' ] [ 10, 'k' ]
[Related recommendations: javascript video tutorial, web front-end]
The above is the detailed content of How to implement array traversal in es6. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


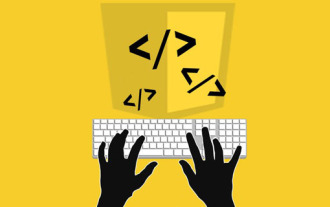
async is es7. async and await are new additions to ES7 and are solutions for asynchronous operations; async/await can be said to be syntactic sugar for co modules and generator functions, solving js asynchronous code with clearer semantics. As the name suggests, async means "asynchronous". Async is used to declare that a function is asynchronous; there is a strict rule between async and await. Both cannot be separated from each other, and await can only be written in async functions.
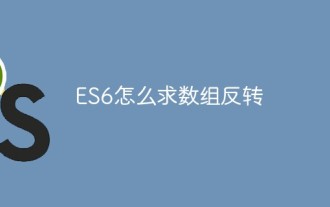
In ES6, you can use the reverse() method of the array object to achieve array reversal. This method is used to reverse the order of the elements in the array, putting the last element first and the first element last. The syntax "array.reverse()". The reverse() method will modify the original array. If you do not want to modify it, you need to use it with the expansion operator "...", and the syntax is "[...array].reverse()".
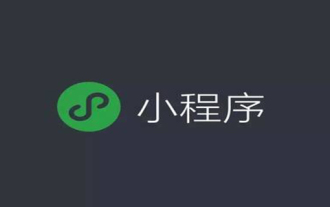
For browser compatibility. As a new specification for JS, ES6 adds a lot of new syntax and API. However, modern browsers do not have high support for the new features of ES6, so ES6 code needs to be converted to ES5 code. In the WeChat web developer tools, babel is used by default to convert the developer's ES6 syntax code into ES5 code that is well supported by all three terminals, helping developers solve development problems caused by different environments; only in the project Just configure and check the "ES6 to ES5" option.

Steps: 1. Convert the two arrays to set types respectively, with the syntax "newA=new Set(a);newB=new Set(b);"; 2. Use has() and filter() to find the difference set, with the syntax " new Set([...newA].filter(x =>!newB.has(x)))", the difference set elements will be included in a set collection and returned; 3. Use Array.from to convert the set into an array Type, syntax "Array.from(collection)".
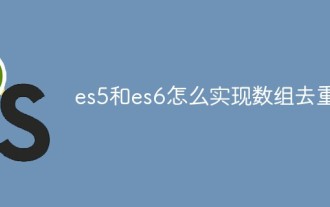
In es5, you can use the for statement and indexOf() function to achieve array deduplication. The syntax "for(i=0;i<array length;i++){a=newArr.indexOf(arr[i]);if(a== -1){...}}". In es6, you can use the spread operator, Array.from() and Set to remove duplication; you need to first convert the array into a Set object to remove duplication, and then use the spread operator or the Array.from() function to convert the Set object back to an array. Just group.
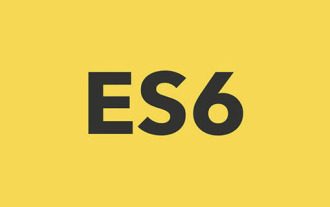
In es6, the temporary dead zone is a syntax error, which refers to the let and const commands that make the block form a closed scope. Within a code block, before a variable is declared using the let/const command, the variable is unavailable and belongs to the variable's "dead zone" before the variable is declared; this is syntactically called a "temporary dead zone". ES6 stipulates that variable promotion does not occur in temporary dead zones and let and const statements, mainly to reduce runtime errors and prevent the variable from being used before it is declared, resulting in unexpected behavior.
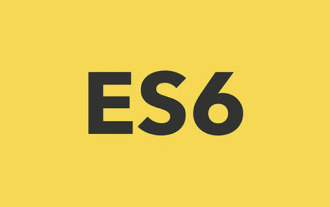
No, require is the modular syntax of the CommonJS specification; and the modular syntax of the es6 specification is import. require is loaded at runtime, and import is loaded at compile time; require can be written anywhere in the code, import can only be written at the top of the file and cannot be used in conditional statements or function scopes; module attributes are introduced only when require is run. Therefore, the performance is relatively low. The properties of the module introduced during import compilation have slightly higher performance.
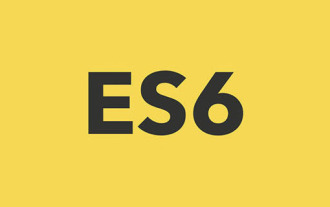
The map is ordered. The map type in ES6 is an ordered list that stores many key-value pairs. The key names and corresponding values support all data types; the equivalence of key names is determined by calling the "Objext.is()" method. Implemented, so the number 5 and the string "5" will be judged as two types, and can appear in the program as two independent keys.
