What are the functions for creating arrays in php?
The functions to create an array are: 1. array(), which accepts a certain number of "key=>value" parameter pairs separated by commas as elements, the syntax is "array(key1=>value1,key2=> ;value2...);"; 2. array_fill(), will create an array by filling key values; 3. array_fill_keys(), will create an array by filling keys and values; 4. array_combine(), Arrays are created by merging arrays.
The operating environment of this tutorial: windows7 system, PHP version 8.1, DELL G3 computer
php provides a variety of functions to create arrays . Let’s find out below.
1. Use the array() function to create an array
The array() function can create a new array. It accepts a certain number of key=>value parameter pairs separated by commas. The syntax format is as follows:
$数组变量名 = array(key1 => value1, key2 => value2, ..., keyN => valueN);
The sample code is as follows:
<?php header("Content-type:text/html;charset=utf-8"); $array = array(0 => '红色', 1 => '黄色', 2 => '蓝色', 3 => '紫色'); echo '<pre class="brush:php;toolbar:false">'; var_dump($array); ?>
If you do not use the =>
symbol to specify the subscript, The default is an indexed array. The default index value also starts from 0 and increases in sequence. The sample code is as follows:
<?php header("Content-type:text/html;charset=utf-8"); $array = array('红色','黄色','蓝色', '紫色'); echo '<pre class="brush:php;toolbar:false">'; var_dump($array); ?>
The running results are the same as those of the previous example.
2. Use the array_fill() function to create an array by filling it
array_fill(): A new array will be created by filling the key value
<?php $arr=array_fill(0,4,"hello"); var_dump($arr); ?>
array_fill($index, $number, $value)
The function accepts 3 parameters that cannot be omitted$index
(starting index), $number
(number of padding elements), and $value
(key value used for padding).
Among them, the $index
parameter supports negative values, and the array index has the following three values:
If it is a positive number, the array The index starts from the
$index
value and ends with the$index $number-1
value. For example,$index
is 2,$number
is 4, then the array index is: 2, 3, 4, 5.If 0, the array index starts from
0
and ends with the$number-1
value. For example,$index
is 0,$number
is 4, then the array index is: 0, 1, 2, 3.If it is negative, the array index is
$index
,0
,1
,2
, ...,$number-2
consists of. For example,$index
is -2,$number
is 4, then the array index is: -2, 0, 1, 2.
In the above example, the $index
of array_fill(0,4,"hello")
is 0 (the index starts from 0) , $number
is 4 (the array has four elements), so the array index is: 0, 1, 2, 3; and $value
is hello
, Then the key values of these four elements are hello
, so the output result is:
3. Use array_fill_keys() The function creates an array by filling it
array_fill_keys(): It will create a new array by filling the key name and key value
<?php $keys=array("a","b","c","d"); $arr=array_fill_keys($keys,"hello"); var_dump($arr); ?>
array_fill_keys($ keys,$value)
The function accepts two non-omitable parameters $keys
(an array containing the key name) and $value
(the key value is filled).
Simply put, the array_fill_keys() function will use the elements in the $keys
array as key names and $value
as values to fill a new array.
As can be seen from the above code example: the new array has four elements, and the key names are "a
", "b
", and "c##" #", "
d"; the key values of these four elements are all "
hello", so the output result is:
##4. Use the array_combine() function to create an array in a merged manner When using the array_combine() function to create an array, the elements in the $keys array and $values array The number must be consistent so that the key name and key value can correspond one-to-one, otherwise an error will be reported and FALSE will be returned.
<?php header("Content-type:text/html;charset=utf-8"); $keys=array("a","b","c","d"); $values=array("red","green","blue","yellow"); var_dump($keys); var_dump($values); echo "使用array_combine()合并数组后:"; var_dump(array_combine($keys,$values)); ?>
Recommended learning: "
The above is the detailed content of What are the functions for creating arrays in php?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


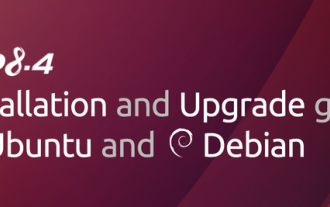
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
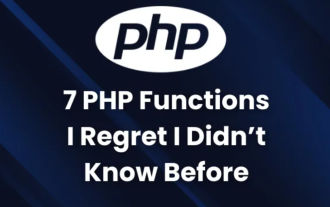
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
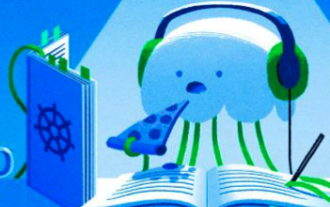
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
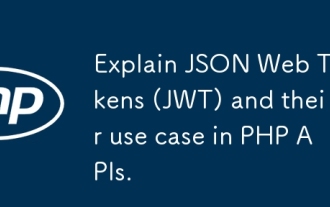
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
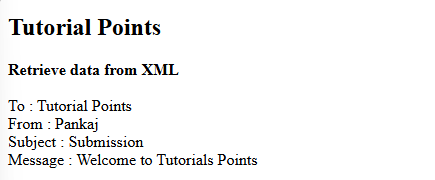
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
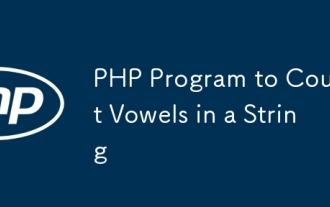
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
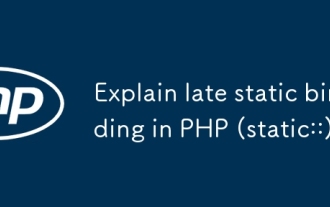
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
