How to determine whether a string is an integer in php
Two methods: 1. Use intval() to convert the string into an integer value, and then use the "==" operator to determine whether the integer value is equal to the original string. The syntax "intval($str)= =$str", if equal, it is an integer. 2. Use is_numeric() and strpos() to determine whether the string is a numeric string without a decimal point. The syntax is "!is_numeric($str)||strpos($str,".")!==false" and return false is an integer, and vice versa is not.
The operating environment of this tutorial: windows7 system, PHP version 8.1, DELL G3 computer
php determines the string Two methods to determine whether it is an integer
Method 1: Use the intval() function and the "==" operator to determine
Use the intval() function to convert the string into an integer value
Use the "==" operator to determine whether the integer value is equal to the original string
<?php header("Content-type:text/html;charset=utf-8"); function f($str){ $result = intval($str); if($result==$str){ echo "$str 字符串是整数<br><br>"; } else{ echo "$str 字符串不是整数<br><br>" ; } } f("a678"); f("678"); f("3.14"); ?>
Method 2: Use is_numeric() function and strpos() function to judge
Use The is_numeric() function determines whether it is a number or a numeric string (integer and floating point number)
Use strpos() to determine whether the number contains a decimal point--exclude floating point numbers
You only need to determine whether the string is a numeric string that does not contain a decimal point
<?php header("Content-type:text/html;charset=utf-8"); function f($str){ if(!is_numeric($str)||strpos($str,".")!==false){ echo "$str 字符串不是整数<br><br>"; }else{ echo "$str 字符串是整数<br><br>"; } } f("a678"); f("3.14"); f("67854"); ?>
Instructions:
intval() function
intval() function is used to get the integer value of a variable.
intval() function returns the integer value of variable var by using the specified base conversion (default is decimal). intval() cannot be used with object, otherwise an E_NOTICE error will be generated and 1 will be returned.
int intval ( mixed $var [, int $base = 10 ] )
Parameter description:
$var: The quantity value to be converted to integer.
#$base: The base used for conversion.
If base is 0, the base used is determined by detecting the format of var:
If the string includes "0x" ( or "0X"), use hexadecimal (hex); otherwise,
If the string starts with "0", use octal (octal); otherwise,
Decimal will be used.
Return value
Returns the integer value of var on success, and returns 0 on failure. An empty array returns 0, a non-empty array returns 1.
The maximum value depends on the operating system. The maximum signed integer range on 32-bit systems is -2147483648 to 2147483647. For example, on such a system, intval('1000000000000') would return 2147483647. On a 64-bit system, the maximum signed integer value is 9223372036854775807.
String may return 0, although it depends on the leftmost character of the string.
is_numeric() function
is_numeric() function is used to detect whether a variable is a number or a numeric string.
bool is_numeric ( mixed $var )
Parameter description:
$var: Variable to be detected.
Return value
If the specified variable is a number or numeric string, it returns TRUE, otherwise it returns FALSE. Note that floating point type returns 1 , that is, TRUE.
strpos() function
strpos() function finds the first occurrence of a string in another string (size sensitive Write).
strpos($string,$find,$start)
Parameter description:
$string: required. Specifies the string to be searched for.
#$find: required. Specifies the characters to search for.
$start: Optional. Specifies the location from which to start the search.
Return value:
Returns the position of the first occurrence of a string in another string, or returns if the string is not found FALSE. Note: The string position starts from 0, not from 1.
Recommended study: "PHP Video Tutorial"
The above is the detailed content of How to determine whether a string is an integer in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


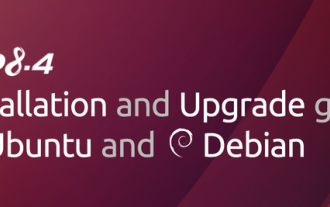
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
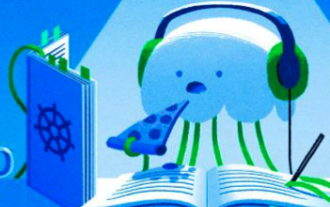
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
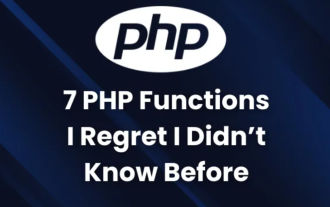
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
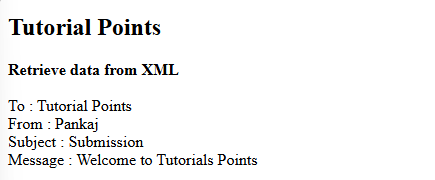
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
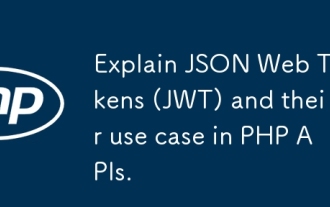
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
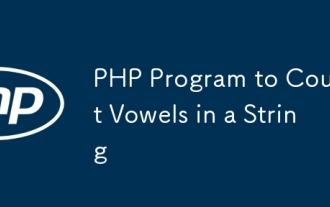
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
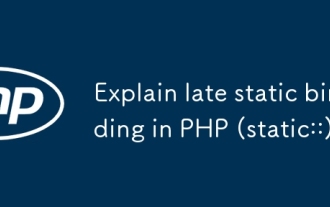
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
