How to determine whether it is a decimal in ES6
Two judgment methods: 1. Use the test() function with the regular expression "/[.]/" to check whether the specified value contains a decimal point, the syntax is "/[.]/.test(specified value) ”, if included, it is a decimal, otherwise it is not. 2. Use the indexOf() function to check whether the specified value contains a decimal point. The syntax is "String(specified value).indexOf(".")". If the return value is greater than "-1", it is a decimal, and vice versa.
The operating environment of this tutorial: Windows 7 system, ECMAScript version 6, Dell G3 computer.
Decimals are ".
" with a decimal point. In JavaScript, you can determine whether a number is a decimal by judging whether it contains ".
" with a decimal point. .
Method 1: Use the test() function with regular expressions to check
The test() method is used to detect whether a string matches a certain pattern. , searches the string for text that matches the regular expression. If a match is found, it returns true; otherwise, it returns false.
RegExpObject.test(string)
Regular expression for checking decimals: /[.]/
Example:
function isDot(num) { var rep=/[.]/; if(rep.test(num)){ console.log(num+" 是小数"); } else{ console.log(num+" 不是小数"); } } isDot(121.121);//是小数 isDot(454.654);//是小数 isDot(454654);//不是小数
Method 2: Use the indexOf() function to check
The indexOf() method can return the position where a specified string value first appears in the string.
string.indexOf(searchvalue,start)
Parameters | Description |
---|---|
searchvalue | Required . Specifies the string value to be retrieved. |
start | Optional integer parameter. Specifies the position in the string to begin searching. Its legal values are 0 to string Object.length - 1. If this parameter is omitted, the search will start from the first character of the string. |
Return value: Find the first occurrence of the specified string. If no matching string is found, -1
will be returned.
Just use indexOf() to check the position where the character ".
" first appears in the string. If the return value is equal to -1, it is a decimal, greater than -1 is not a decimal.
Example:
function isDot(num) { if(String(num).indexOf(".")>-1){ console.log(num+" 是小数"); } else{ console.log(num+" 不是小数"); } } isDot(121.121);//含有小数点 isDot(454654);//不含小数点 isDot(45465.4);//含小数点
[Recommended learning: javascript advanced tutorial]
The above is the detailed content of How to determine whether it is a decimal in ES6. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


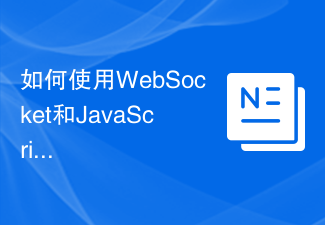
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
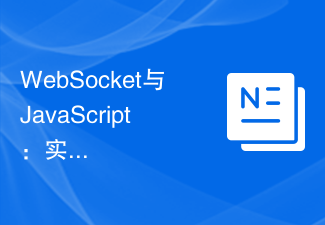
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
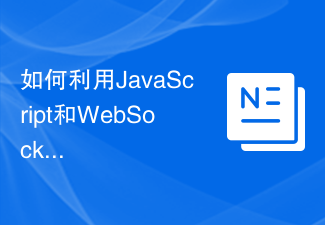
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
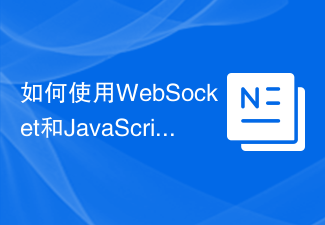
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
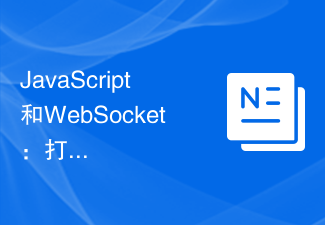
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
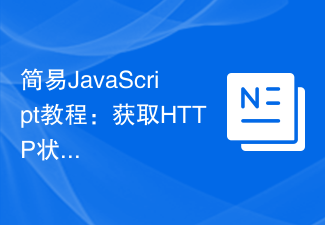
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
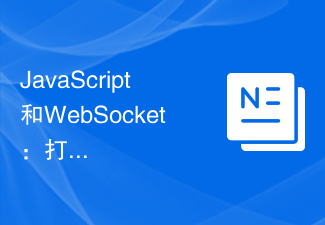
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
