Is async an es6 attribute?
Belongs to, async is a new feature of es6, which is used to indicate that there may be asynchronous processes in the program. The function declared with the async keyword returns a Promise object. If a direct value is returned in the function, async will encapsulate the direct value into a Promise object through Promise.resolve(); when the async function does not return a value, it returns " Promise.resolve(undefined)".
The operating environment of this tutorial: Windows 7 system, ECMAScript version 6, Dell G3 computer.
ES6 new features async and await keywords
1. Preliminary understanding
Let’s understand this literally Two keywords, async is the abbreviation of asynchronous (asynchronous), and await can be thought of as the abbreviation of async wait. So async can be understood as being used to declare a function to be asynchronous, while await is used to wait for an asynchronous task to complete.
The async and await keywords allow us to write promise-based asynchronous behavior in a more concise way without deliberately chaining promises.
Next, let’s take a preliminary look at the functions of async and await through a few examples.
Knowledge point 1: Functions declared with the async keyword return a Promise object. If you return
a direct value in a function, async will encapsulate the direct value into a Promise object through Promise.resolve()
. When the async
function does not return a value, it returns Promise.resolve(undefined)
//定义一个普通函数,返回一个字符串 function test() { return "hello async"; } const result1 = test(); console.log(result1); //输出一个字符串 hello async //定义一个使用了async修饰的函数,同样返回一个字符串 async function testAsync() { return "hello async"; } const result2 = testAsync(); console.log(result2); //输出一个Promise对象 Promise {<fulfilled>: 'hello async'}
//async较好的用法 async function testAsync(){ //返回一个Promise对象 return new Promise((resolve, reject)=>{ //处理异步任务 setTimeout(function () { resolve("testAsync") }, 1000); }) } //async通常用于声明一个处理异步任务且返回了Promise对象的函数
Knowledge point 2: The await keyword can only be used Within the function declared by async, it is used to modify a Promise object so that the asynchronous tasks processed by the Promise object are executed synchronously in sequence on the current coroutine.
//定义一个使用async修饰的函数,处理异步任务 async function testAsync(){ return new Promise((resolve, reject)=>{ setTimeout(function () { resolve("testAsync") }, 1000); }) }
//定义一个函数,直接调用testAsync函数 function testAwait(){ console.log('testAsync调用前') testAsync().then(res=>{ console.log(res) //输出"testAsync" }) console.log('testAsync调用后') } /***** 输出如下 *****/ testAsync调用前 testAsync调用后 testAsync //尽管代码按顺序写,但不按顺序执行,因为testAsync()是异步函数
//定义一个函数(不使用async声明该函数)用await修饰调用testAsync函数 function testAwait(){ console.log('testAsync调用前') await testAsync().then(res=>{ //使用await关键字修饰 console.log(res) }) console.log('testAsync调用后') } //调用testAwait()函数 testAwait() //报错:Uncaught SyntaxError: await is only valid in async functions and the top level bodies of modules,因为await只能使用在被async修饰的函数内。
//定义一个函数(使用async声明该函数)用await修饰调用testAsync函数 async function testAwait(){ console.log('testAsync调用前') await testAsync().then(res=>{ console.log(res) }) console.log('testAsync调用后') } /***** 输出如下 *****/ testAsync调用前 testAsync testAsync调用后 //使用了await关键字修饰,使得代码按照顺序执行,即同步执行
2. async keyword
(1) Used to indicate that there may be asynchronous processes in the program
(2) async function return value The type is Promise object: This is essentially different from ordinary functions, and it is also the key point to pay attention to when using it;
- return newPromise( ), this usage is in line with the original intention of the async function;
- return data, especially note that writing like this is equivalent to Promise.resolve(data). The returned data is encapsulated into a Promise object, but when the async function is called, the return value data cannot be obtained through a simple = , because the return value is a Promise object, you need to use .then(data => { }) to get this data;
- If there is no return value, it is equivalent to returning Promise.resolve(undefined );
(3) No waiting, non-blocking: If there is an asynchronous process in a function declared with the async keyword, it may wait, but the function itself will return immediately and will not block the current main thread. If the asynchronous process modified with the await keyword is used in the function, it will work on the corresponding coroutine and will block and wait for the completion of the asynchronous task before returning.
//定义一个函数,处理异步任务(使用定时器模拟),返回一个Promise对象 async function testAsync(){ return new Promise((resolve, reject) => { setTimeout(function () { resolve("成功调用testAsync") }, 1000); }); } //定义一个函数,使用await关键字修饰调用testAsync()函数 async function testAwait(){ //使用了await关键字修饰调用testAsyn()函数 await this.testAsync().then(res=>{ console.log(res) //输出的是testAsync()函数resolve的值 }); console.log("helloAsync"); } //主线程 console.log('testAwait调用前') testAwait(); console.log('testAwait调用后') /***** 输出结果如下 *****/ testAwait调用前 testAwait调用后 //因为testAwait()函数使用了async关键字修饰,所以不会阻塞主线程的执行,所以这两句话会先直接输出,然后再执行testAwait()函数 成功调用testAsync //因为testAwait()函数在内部调用testAsync()函数时使用了await关键字修饰,所以在对应的协程上会阻塞,等待testAsync()函数执行完,再输出下面那句'helloAsync' helloAsync
3. await keyword
(1) await can only be used inside async functions: it cannot be placed inside ordinary functions, otherwise an error will be reported.
(2) The await keyword is followed by a Promise object. If it is followed by a function, this function should return a Promise object. If it is followed by a non-Promise object, it will be automatically packaged into a Promise object through the Promise.resolve() function and placed in the fulfilled state.
//例如: const a = await 'Hello Await' // 相当于 const a = await Promise.resolve('Hello Await'); console.log(a) //输出 'Hello Await'
(3) The essence of await is to wait for the fulfilled status of the Promise object it modifies and return the data of resolve(data).
means that if await is followed by a Promise
object, await
will block the following code and wait for the Promise
objectresolve
, and then get the value of resolve
as the result of the await
expression.
async function testAsync(){ return new Promise((resolve, reject) => { setTimeout(function () { resolve("成功调用testAsync") }, 1000); }); } const a = await testAsync() //这里的a就会拿到testAsync函数resolve的数据 console.log(a) //在一秒后输出'成功调用testAsync'
(4) await does not care about the rejected status of the Promise object it modifies, that is, the data of reject (data) will not be processed by await, so it is recommended to call catch through the Promise object to capture it.
async testAwait(){ //变量a用于接收testAsync()函数resolve的数据 let a = await testAsync().catch(err=>{ //处理异常和reject的数据 }) }
4. In-depth explanation of async and await
(1) Execution sequence
//定义一个函数,该函数接收一个参数,1s后再返回参数的两倍 async function double(num) { return new Promise((resolve, reject) => { setTimeout(() => { //使用定时器模拟异步任务 resolve(2 * num) //将运算结果交给resolve }, 1000); }) } async function getResult () { console.log('double调用前') //顺序:2 let result = await double(10); //将10作为参数传递给double函数 //result变量用于接收double()函数resolve的值 console.log(result); //顺序:4 console.log('double调用后') //顺序:4 } console.log('getResult调用前') //顺序:1 getResult(); console.log('getResult调用后') //顺序:3 /***** 依次输出如下 *****/ getResult调用前 double调用前 getResult调用后 20 //1s后输出 double调用后
①First print the outputbefore calling getResult
, synchronous code, executed sequentially;
② Then call the method getResult(), print out double before calling
, synchronous code, executed sequentially;
③ Then call asynchronous Method double( )
If the await keyword is not used here, the output in sequence is: before getResult is called, before double is called, after double is called, after getResult is called, and output 20# after 1s
##Because the asynchronous operation will not affect the execution of other codes, other codes will be executed in order, and finally the double function will be executed
Because the await keyword is used here , so the getResult() code will be blocked when executed here. Wait until the double function resolves, and then continue execution
④尽管getResult函数内部被await阻塞了,由于getResult函数本身也是个async函数,所以它不会影响getResult函数外面的代码执行。因为调用async函数不会造成阻塞,它内部的所有阻塞都被封装在一个Promise对象中异步执行。
⑤所以在调用getResult函数后,会继续向下执行,即打印输出getResult调用后
⑥当1s之后,异步函数double执行完成,将结果交给resolve。
⑦通过await关键字接收到double函数resolve的值,赋值给result变量。打印输出20
⑧因为使用了await阻塞将异步变为同步,所以在打印输出20后再打印输出double调用后
(2)处理reject回调
//方法一:通过promise对象的catch进行捕获 function a(){ return new Promise((resolve,reject) => { setTimeout(() => { reject("something") }, 1000) }) } async function b(){ let r = await a().catch((err)=>{ console.log(err) }) }
//方法二:通过try/catch语句处理 function a(){ return new Promise((resolve,reject) => { setTimeout(() => { reject("something") }, 1000) }) } async function b(){ let r = null try{ r = await a() }catch(err){ console.log(err) } }
(3)使用await优化Promise对象的回调地狱问题
在Promise章节中我们通过了Promise对象的then( )方法链式调用解决了回调地狱问题,但看起来仍然不够美观,我们可以通过await优化一下,让它看起来更符合我们平时代码的编写习惯。
//原本的解决方案 //第二个请求依赖于第一个请求的返回值,第三个请求依赖于第二个请求的返回值 request1().then(function(data){ return request2(data) }).then(function(data){ return request3(data) }) //这里只发送了三次请求,代码看起来还不错,虽然它已经比普通的回调函数形式好了很多。 //那如果需要发送五次或十次请求呢?代码也许会没那么美观,接下来我们使用学习到的await去解决这个问题。
原本的要求是每个请求都依赖于上一个请求的返回值,那么是不是得等一个请求完,才能发送下一个请求?这时我们可以思考一下,await的作用是什么?是不是对一个Promise对象去进行阻塞,使其状态变为fulfilled后获取resolve的值。这不就正是我们所需要的。
//使用await的解决方案 var res1 = await request1() //将request1的返回值赋值给res1 var res2 = await request2(res1) //将res1作为参数传给request2,并将request2的返回值赋值给res2 var res3 = await request3(res2) //同理 //这样子写的代码更加的美观,并且更符合我们平时编写代码的习惯
【相关推荐:javascript视频教程、编程视频】
The above is the detailed content of Is async an es6 attribute?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


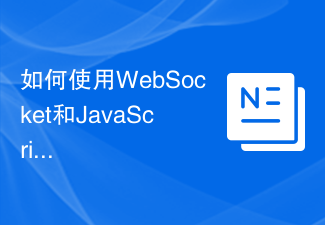
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
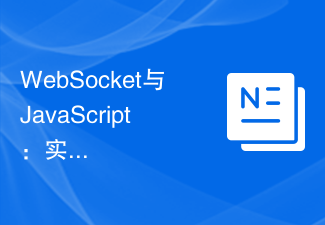
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
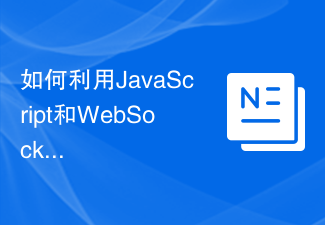
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
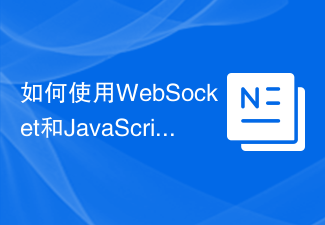
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
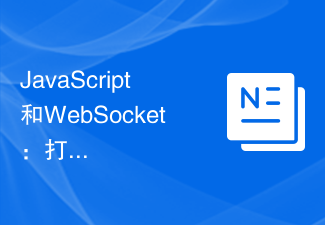
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
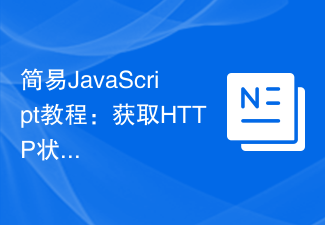
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
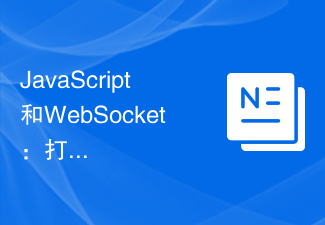
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
