Is es6 map ordered?
map is ordered. The map type in ES6 is an ordered list that stores many key-value pairs. The key names and corresponding values support all data types; the equivalence of key names is determined by calling the "Objext.is()" method. Implemented, so the number 5 and the string "5" will be judged as two types, and can appear in the program as two independent keys.
The operating environment of this tutorial: Windows 7 system, ECMAScript version 6, Dell G3 computer.
1. Map collection
The object of JavaScript is essentially a collection of key-value pairs, but traditionally it can only be used as a string. Key-value pairs.
To solve this problem, ES6 provides the map data structure. It is similar to an object and is also a collection of key-value pairs. However, the scope of this key is not limited to strings. Various types of values (including objects) can be used as keys. In other words, the object structure provides (string-value) correspondence, and the map structure implements
The map type in ES6 is an ordered list that stores many key-value pairs, in which the key name and the corresponding Value supports all data types. The equivalence judgment of key names is achieved by calling the Objext.is() method, so the number 5 and the string '5' will be judged as two types and can appear in the program as two independent keys.
Note: There is an exception. 0 and -0 are considered equal in the map collection, which is different from the Object.is() result. If you need "key value" For "data structure, map is more suitable than object, and has extremely fast search speed
1. Attribute: size
Returns map Number of elements
2, basic method
(1) set()
to map Add data and return the added map (assigning a value to an existing key will overwrite the previous value)
(2) get()
Get a certain The value of the key returns the value corresponding to the key. If not, returns undefined
(3) has()
Detects whether a certain key exists and returns a Boolean value
let map = new Map(); map.set('JacksonWang','123'); map.set('LEO','456'); map.set('Z-','789'); console.log(map.size); console.log(map.get('JacksonWang')); console.log(map.get('LEO')); console.log(map.has('Z-')); //输出: 3 // 123 // 456 // true
(4)delete()
Delete a key and its corresponding value and return a Boolean value. If the deletion is successful, it will be true
(5)clear()
Clear all values and return undefined
let map = new Map(); map.set('JacksonWang','123'); map.set('LEO','456'); map.set('Z-','789'); map.delete('Z-'); console.log(map.size); console.log(map.clear()) //输出: 2 // undefined
3. Traversal method
Note: The traversal order of the map is the insertion order
(1) keys()
Get all the keys of the map
( 2) values()
Get all the values of the map
(3)entries()
Get all the members of the map
let map = new Map(); map.set('JacksonWang','123'); map.set('LEO','456'); map.set('Z-','789'); console.log(map.keys())//打印所有的键 console.log(map.values())//打印所有的值 console.log(map.entries())//以键值对的方式 /*输出: [Map Iterator] { 'JacksonWang', 'LEO', 'Z-' } [Map Iterator] { '123', '456', '789' } [Map Entries] { [ 'JacksonWang', '123' ], [ 'LEO', '456' ], [ 'Z-', '789' ] }*/
(4) forEach()
Traverse all members of map
let map = new Map(); map.set('JacksonWang','123'); map.set('LEO','456'); map.set('Z-','789'); for(const [key,value] of map.entries()){ console.log(`${key}:${value}`); } /*输出: JacksonWang:123 LEO:456 Z-:789 */
4. Convert to array
Convert map structure to array destructuring
let map1 = new Map([ [1,'One'], [2,'Two'], [3,'Three'] ]) console.log([...map1.keys()]); console.log(...map1.entries()) console.log([...map1.entries()]); /*输出: [ 1, 2, 3 ] [ 1, 'One' ] [ 2, 'Two' ] [ 3, 'Three' ] [ [ 1, 'One' ], [ 2, 'Two' ], [ 3, 'Three' ] ] */
2. Weakmap collection
WeakMap is a weak reference Map collection, also used to store objects Weak reference. The key name in the WeakMap collection must be an object. If you use a non-object key name, an error will be reported: the collection stores weak references to these objects. If there are no other strong references other than the weak references, the engine's garbage collection mechanism This object will be automatically recycled, and the key-value pairs in the WeakMap collection will be removed. But only the key name of the collection follows this rule. If the value corresponding to the key name is an object, the strong reference of the saved object will not trigger the garbage collection device
1, WeakMap collection Purpose
#(1) Store DOM elements
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <button id="btn">WeskMap测试</button> <script> let btn = document.querySelector('#btn'); let wmap = new WeakMap(); wmap.set(btn,{timesClicked:0})//在map中放一个键值对,btn为键 btn.addEventListener('click',function(){ let temp = wmap.get(btn);//从这里获取键名为btn的值 temp.timesClicked++; console.log(temp.timesClicked) },false) </script> </body> </html>
myElement in the code is a DOM node, which is updated whenever a click event occurs status. We put this state as a key value in the WeakMap, and the corresponding key name is myElement. Once the DOM node is deleted, the state will automatically disappear, and there is no risk of memory leaks
(2) Register the listener object for listening events
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <button id="btn">WeskMap测试</button> <script> let btn = document.querySelector('#btn'); let wmap = new WeakMap(); wmap.set(btn,{timesClicked:0})//在map中放一个键值对,btn为键 // btn.addEventListener('click',function(){ // let temp = wmap.get(btn);//从这里获取键名为btn的值 // temp.timesClicked++; // console.log(temp.timesClicked) // },false) function f1(){ let temp = wmap.get(btn);//从这里获取键名为btn的值 temp.timesClicked++; console.log(temp.timesClicked) } btn.addEventListener('click',f1,false) </script> </body> </html>
The effect is the same
(3) Deploy private properties
function Person(name){ this._name = name; } Person.prototype.getName = function(){ return this._name; } //但这是,创建一个Person对象的时候,我们可以直接访问name let p = new Person('张三'); console.log(p._name) //输出:张三
We don’t want users to directly access the name attribute, so we directly use the following method to wrap name into a private attribute
let Person = (function(){ let privateData = new WeakMap(); function Person(yourname){ privateData.set(this,{_name:yourname})//this当前这个键的对象 } Person.prototype.getName = function(){ return privateData.get(this)._name;// } return Person; })();//定义好了函数就开始执行 let p = new Person('jack'); console.log(p._name)//因为name的私有型,所以在外不可访问 console.log(p.getName()) /*输出: undefined jack */
[Related recommendations: javascript video tutorial, webfrontend】
The above is the detailed content of Is es6 map ordered?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


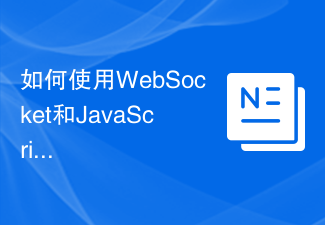
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
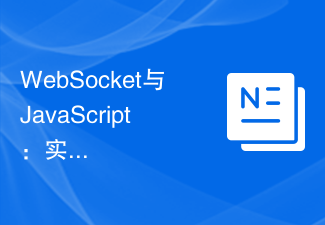
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
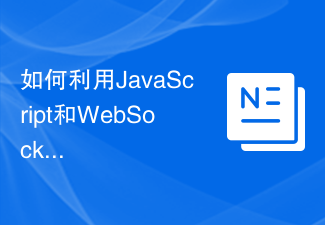
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
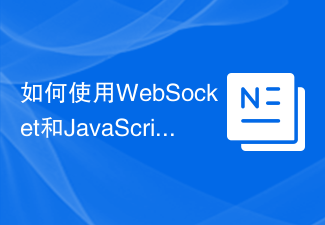
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
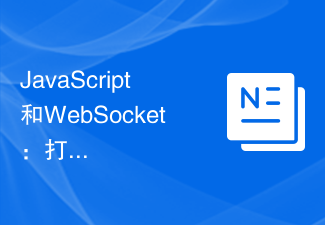
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
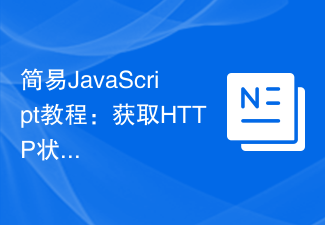
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
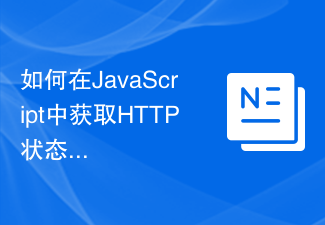
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
