Is promise based on es6?
Yes. Promise is a new reference type in ECMAScript 6, which represents the final completion or failure of an asynchronous operation. Promise solves the problem of overly complex logic writing in asynchronous programming calling code. When the network request is very complex, callback hell will occur. In this way, if these codes are written together, they will look very complicated and not conducive to reading. If you use Promises will make the code look more beautiful and elegant.
The operating environment of this tutorial: Windows 7 system, ECMAScript version 6, Dell G3 computer.
ECMAScript 6 adds complete support for the Promises/A specification, that is, the Promise type. Once launched, Promise became extremely popular and became the dominant asynchronous programming mechanism. All modern browsers support ES6 expectations, and many other browser APIs are based on expectations.
Promise is a new reference type in ECMAScript 6, which represents the final completion or failure of an asynchronous operation.
1. What does the promise function do?
The promise function solves the problem of overly complex writing of asynchronous programming calling code logic. When the network request is very complex, it will Callback hell appears, so if these codes are written together, they will look very complicated and not conducive to reading. If you use promise, the code will look more beautiful and elegant.
2. Promise 3 A state
First of all, when we have an asynchronous operation in development, we can wrap a Promise for the asynchronous operation
There will be three states after the asynchronous operation
pending:等待状态,比如正在进行网络请求,或者定时器没有到时间。 fulfill:满足状态,当我们主动回调了resolve时,就处于该状态,并且会回调.then() reject:拒绝状态,当我们主动回调了reject时,就处于该状态,并且会回调.catch()
3. Implement
1, then and catch
1. Functions in a pending state are synchronous and will be executed immediately
2.then and catch are asynchronous. Even if there is no asynchronous operation in the promise object and the then method or catch is executed immediately, the two methods here may be added to the event queue to wait for execution
//参数 函数(resolve,reject) new Promise((resolve, reject) => { setTimeout(() => { //请求成功的时候调用resolve resolve('22222') //请求失败的时候调用reject reject('error message') }, 1000) }).then((data) => { //请求成功处理函数 console.log(data) }).catch((err) => { //请求失败处理函数 console.log(err) })
2. Determine the status
1. If an uncaught error occurs in the pending status processing function, the status will be pending and will directly become the rejected status and can be caught. Capture
var pro = new Promise((resolve, reject) => { throw new Error("123"); // try{ // throw new Error("123"); // } catch(e) {} resolve(12); reject(34); }) // pro.then(data => { // console.log(data); // }, err => { // console.log(err); // }) console.log(pro); pro.then(data => { console.log(data); }) pro.catch(data => { console.log(data); })
3.async and await
1.Use Promise:
const makeRequest = () => getJSON().then(data => { console.log(data) return "done" }) makeRequest()
2.Use Async :
async and await are proposed by ES7
The role of async:Simplify the creation of promise objects in function return values
General situation Below, async is written at the front of the function, and the return value of the modified function must be a promise object. Only in some special cases will a promise object be returned manually.
Function: Solve asynchronous problems like promise, but its advantage is to make asynchronous code the same as synchronous!!
Note: In synchronous method, we get the result through the return value , the asynchronous method gets the result by relying on the callback function.
Basic syntax used by async and await:
It is to add an async in front of the ordinary function and the call is the same as the ordinary function
Async is generally used in conjunction with await
await is followed by a promise object await must be used in an asynchronous function
const makeRequest = async () => { // await getJSON()表示console.log会等到getJSON的promise成功reosolve之后再执行。 console.log(await getJSON) return "done" } makeRequest()
3. Difference
1. There is an extra aync keyword in front of the function. The await keyword can only be used within functions defined by aync. The async function will implicitly return a promise, and the resolve value of the promise is the value of the function return. (The reosolve value in the example is the string "done")
2. We cannot use await in the outermost code because it is not within the async function.
4.promise method
var r1 = new Promise((resolve,reject) => { setTimeout(function(){ resolve("我是第一个请求"); },1000) }) var r2 = new Promise((resolve,reject) => { setTimeout(function(){ resolve("我是第二个请求"); },3000) }) var r3 = new Promise((resolve,reject) => { setTimeout(function(){ resolve("我是第三个请求"); },4000) }) var r4 = new Promise((resolve,reject) => { setTimeout(function(){ resolve("我是第四个请求"); },500) })
1.all method
Sometimes we need to wait for two or more requests to return successfully before proceeding In the next step, the all method of promise is to wait for all asynchronous requests to be completed before making the next callback
Promise.all([r1,r2,r3,r4]).then(data => { console.log(data); })
2.race method
The requests are sent out at the same time. Whoever comes back first will use whose data .
Promise.race([r1,r2,r3,r4]).then(data => { console.log(data); })
5. Promise encapsulation ajax case
<script> function toData(obj) { // 声明一个数组 来装每一组的数据 var arr = []; if(obj !== null) { for(var key in obj) { let str = key + "=" + obj[key]; arr.push(str); } return arr.join("&"); } } function ajax(obj) { return new Promise(function(resolve, reject) { // 给ajax所需要的参数设置默认值 obj.type = obj.type || "get"; obj.async = obj.async|| "true"; obj.dataType = obj.dataType || "json"; obj.data = obj.data || null; // 开始发送ajax请求 var xhr; if(window.XMLHttpRequest) { xhr = new XMLHttpRequest(); } else { // IE低版本的浏览器 xhr = new ActiveXObject("Microsoft.XMLHttp"); } // 判断是post请求 还是get请求 if(obj.type === "post") { xhr.open(obj.type, obj.url, obj.async); // 设置请求头 xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded"); xhr.send(toData(obj.data)); } else { var url = obj.url + "?" + toData(obj.data); xhr.open(obj.type, url, obj.async); xhr.send(); } // 处理响应体 xhr.onreadystatechange = function() { if(xhr.readyState == 4) { if(xhr.status >= 200 && xhr.status < 300 || xhr.status == 304) { resolve(JSON.parse(xhr.responseText)); } else { reject(xhr.status); } } } }) } ajax({ url : "./data.php", data : { name : "jack", age : 16 } }).then(res => { console.log(res); }, err => { console.log(err); }) </script>
[Related recommendations: javascript video tutorial, web front-end]
The above is the detailed content of Is promise based on es6?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


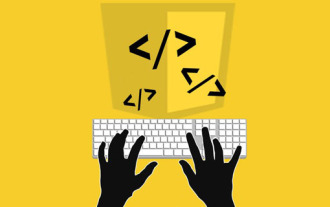
async is es7. async and await are new additions to ES7 and are solutions for asynchronous operations; async/await can be said to be syntactic sugar for co modules and generator functions, solving js asynchronous code with clearer semantics. As the name suggests, async means "asynchronous". Async is used to declare that a function is asynchronous; there is a strict rule between async and await. Both cannot be separated from each other, and await can only be written in async functions.
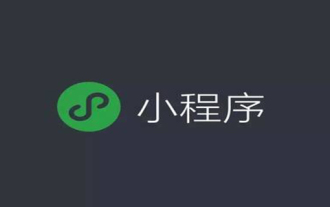
For browser compatibility. As a new specification for JS, ES6 adds a lot of new syntax and API. However, modern browsers do not have high support for the new features of ES6, so ES6 code needs to be converted to ES5 code. In the WeChat web developer tools, babel is used by default to convert the developer's ES6 syntax code into ES5 code that is well supported by all three terminals, helping developers solve development problems caused by different environments; only in the project Just configure and check the "ES6 to ES5" option.
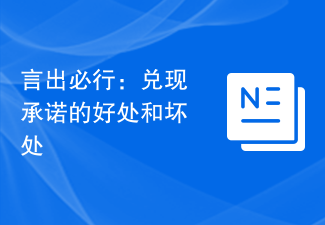
In daily life, we often encounter problems between promises and fulfillment. Whether in a personal relationship or a business transaction, delivering on promises is key to building trust. However, the pros and cons of commitment are often controversial. This article will explore the pros and cons of commitments and give some advice on how to keep your word. The promised benefits are obvious. First, commitment builds trust. When a person keeps his word, he makes others believe that he is a trustworthy person. Trust is the bond established between people, which can make people more
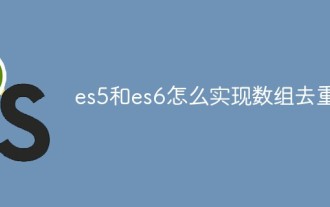
In es5, you can use the for statement and indexOf() function to achieve array deduplication. The syntax "for(i=0;i<array length;i++){a=newArr.indexOf(arr[i]);if(a== -1){...}}". In es6, you can use the spread operator, Array.from() and Set to remove duplication; you need to first convert the array into a Set object to remove duplication, and then use the spread operator or the Array.from() function to convert the Set object back to an array. Just group.
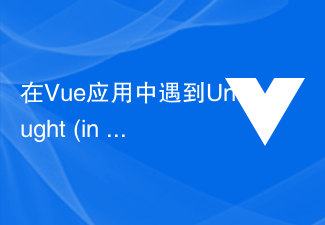
Vue is a popular front-end framework, and you often encounter various errors and problems when developing applications. Among them, Uncaught(inpromise)TypeError is a common error type. In this article, we will discuss its causes and solutions. What is Uncaught(inpromise)TypeError? Uncaught(inpromise)TypeError error usually appears in
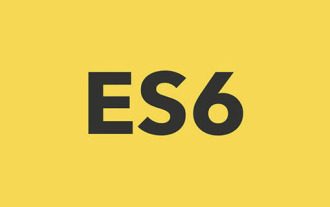
In es6, the temporary dead zone is a syntax error, which refers to the let and const commands that make the block form a closed scope. Within a code block, before a variable is declared using the let/const command, the variable is unavailable and belongs to the variable's "dead zone" before the variable is declared; this is syntactically called a "temporary dead zone". ES6 stipulates that variable promotion does not occur in temporary dead zones and let and const statements, mainly to reduce runtime errors and prevent the variable from being used before it is declared, resulting in unexpected behavior.
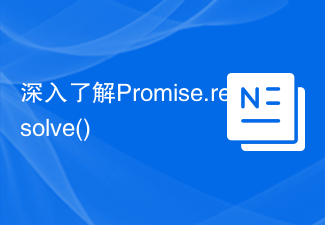
Detailed explanation of Promise.resolve() requires specific code examples. Promise is a mechanism in JavaScript for handling asynchronous operations. In actual development, it is often necessary to handle some asynchronous tasks that need to be executed in sequence, and the Promise.resolve() method is used to return a Promise object that has been fulfilled. Promise.resolve() is a static method of the Promise class, which accepts a
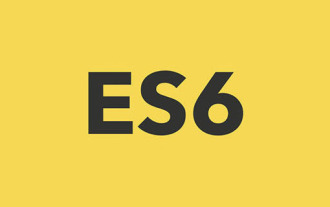
ES6 import will cause variable promotion. Variable hoisting is the promotion of a variable declaration to the very beginning of its scope. js has to go through the compilation and execution phases. During the compilation phase, all variable declarations will be collected and variables declared in advance, and other statements will not change their order. Therefore, during the compilation phase, the first step is already is executed, and the second part is executed only when the statement is executed in the execution phase.
