An article explaining the details of using Redux Hooks
This article will talk about the details of using Redux Hooks. I hope it will be helpful to everyone!
##Redux Hooks
Introduction to Hooks in Redux
In previous redux development, in order to combine components with redux, we used connect in the react-redux library:But this method must use high-order functions to combine the returned high-order Component; and must write: mapStateToProps and mapDispatchToProps mapping functions, the specific usage is explained in the previous article; [Related recommendations:Starting from Redux7.1, the Hook method is provided. There is no need to write connect and corresponding mapping functions in the function component.
The function of useSelector is to map state to the component
:
Parameter 1: It is required to pass in a callback function, and the state will be passed to the callback function; the callback function The return value is required to be an object. Write the data to be used in the object. We can directly deconstruct the returned object and get the data in the state that we want to use.Parameter 2: Can be compared Determine whether the component is re-rendered;const { counter } = useSelector((state) => { return { counter: state.counter.counter } })Copy after login
useSelector will compare the two objects we return by default to see if they are equal;
How can we compare?In the second parameter of useSelector, passing in the
That is, we must Returning two completely equal objects will not cause re-rendering;- shallowEqual
function in the react-redux library can be compared
import { shallowEqual } from 'react-redux' const { counter } = useSelector((state) => ({ counter: state.counter.counter }), shallowEqual)Copy after login
useDispatch is very simple, just call the useDispatch Hook, and you can directly get the dispatch function. Then you can use it directly in the component
;
const dispatch = useDispatch()
Using Hooks in Redux
Let’s use Redux’s Hooks to implement a counter in the App component, and implement a case of modifying the message in the sub-component of the App:First we create a simple store// store/modules/counter.js import { createSlice } from "@reduxjs/toolkit"; const counterSlice = createSlice({ name: "counter", initialState: { counter: 10, message: "Hello World" }, reducers: { changeNumberAction(state, { payload }) { state.counter = state.counter + payload }, changeMessageAction(state, {payload }) { state.message = payload } } }) export const { changeNumberAction, changeMessageAction } = counterSlice.actions export default counterSlice.reducerCopy after login// store/index.js import { configureStore } from "@reduxjs/toolkit"; import counterSlice from "./modules/counter" const store = configureStore({ reducer: { counter: counterSlice } }) export default storeCopy after login
To use the react-redux library, you need to import Provider to wrap the App componentimport React from "react" import ReactDOM from "react-dom/client" import { Provider } from "react-redux" import App from "./12_Redux中的Hooks/App" import store from "./12_Redux中的Hooks/store" const root = ReactDOM.createRoot(document.querySelector("#root")) root.render( <Provider store={store}> <App/> </Provider> )Copy after login
Use useSelector and useDispatch in the component to implement the operations of obtaining data in the store and modifying the data in the storeimport React, { memo } from 'react' import { useDispatch, useSelector } from 'react-redux' import { changeMessageAction, changeNumberAction } from './store/modules/counter' // 子组件Home const Home = memo(() => { console.log("Home组件重新渲染") // 通过useSelector获取到store中的数据 const { message } = useSelector((state) => ({ message: state.counter.message })) // useDispatch获取到dispatch函数 const dispatch = useDispatch() function changeMessage() { dispatch(changeMessageAction("Hello ChenYq")) } return ( <div> <h2>{message}</h2> <button onClick={changeMessage}>修改message</button> </div> ) }) // 根组件App const App = memo(() => { console.log("App组件重新渲染") // 通过useSelector获取到store中的数据 const { counter } = useSelector((state) => ({ counter: state.counter.counter })) // useDispatch获取到dispatch函数 const dispatch = useDispatch() function changeNumber(num) { dispatch(changeNumberAction(num)) } return ( <div> <h2>当前计数: {counter}</h2> <button onClick={() => changeNumber(1)}>+1</button> <button onClick={() => changeNumber(-1)}>-1</button> <Home/> </div> ) }) export default AppCopy after login
Now we have used and modified it in the component The data in the store, but now there is a small problem (Performance Optimization)
When the counter is modified in the App component, the App component will re-render this There is no problem; but the Home component uses message and does not use counter, but it will be re-rendered; similarly, if the message is modified in the Home sub-component, the root component App will also be re-rendered; this is because by default UseSelector is the entire state that is monitored. When the state changes, it will cause the component to be re-renderedTo solve this problem, we need to use the second parameter of useSelector to control whether re-rendering is needed. We only need to use the useSelector function Just pass in theFor more programming-related knowledge, please visit:shallowEqual
function in the react-redux library, it will automatically perform a shallow comparison internally, and will only re-render when the data in the state used does change
import React, { memo } from 'react' import { useDispatch, useSelector, shallowEqual } from 'react-redux' import { changeMessageAction, changeNumberAction } from './store/modules/counter' // 子组件Home const Home = memo(() => { console.log("Home组件重新渲染") const { message } = useSelector((state) => ({ message: state.counter.message }), shallowEqual) const dispatch = useDispatch() function changeMessage() { dispatch(changeMessageAction("Hello ChenYq")) } return ( <div> <h2>{message}</h2> <button onClick={changeMessage}>修改message</button> </div> ) }) // 根组件App const App = memo(() => { console.log("App组件重新渲染") // 通过useSelector获取到store中的数据 const { counter } = useSelector((state) => ({ counter: state.counter.counter }), shallowEqual) // useDispatch获取到dispatch函数 const dispatch = useDispatch() function changeNumber(num) { dispatch(changeNumberAction(num)) } return ( <div> <h2>当前计数: {counter}</h2> <button onClick={() => changeNumber(1)}>+1</button> <button onClick={() => changeNumber(-1)}>-1</button> <Home/> </div> ) }) export default AppCopy after login
Programming Teaching! !
The above is the detailed content of An article explaining the details of using Redux Hooks. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
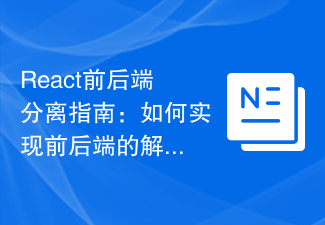
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
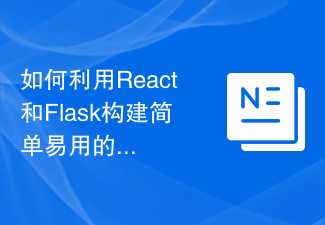
How to use React and Flask to build simple and easy-to-use web applications Introduction: With the development of the Internet, the needs of web applications are becoming more and more diverse and complex. In order to meet user requirements for ease of use and performance, it is becoming increasingly important to use modern technology stacks to build network applications. React and Flask are two very popular frameworks for front-end and back-end development, and they work well together to build simple and easy-to-use web applications. This article will detail how to leverage React and Flask
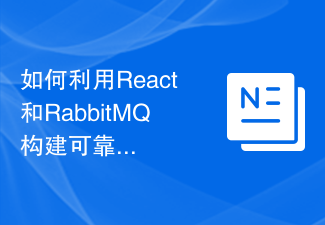
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
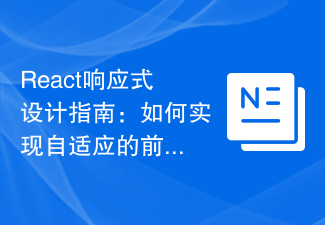
React Responsive Design Guide: How to Achieve Adaptive Front-end Layout Effects With the popularity of mobile devices and the increasing user demand for multi-screen experiences, responsive design has become one of the important considerations in modern front-end development. React, as one of the most popular front-end frameworks at present, provides a wealth of tools and components to help developers achieve adaptive layout effects. This article will share some guidelines and tips on implementing responsive design using React, and provide specific code examples for reference. Fle using React
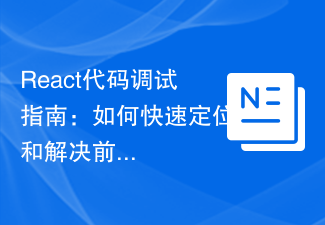
React code debugging guide: How to quickly locate and resolve front-end bugs Introduction: When developing React applications, you often encounter a variety of bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications. 1. Selection of debugging tools: In Re
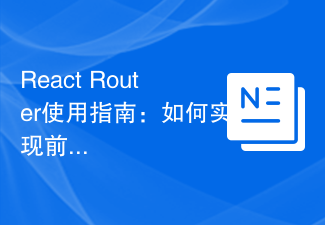
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
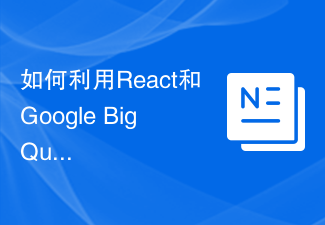
How to use React and Google BigQuery to build fast data analysis applications Introduction: In today's era of information explosion, data analysis has become an indispensable link in various industries. Among them, building fast and efficient data analysis applications has become the goal pursued by many companies and individuals. This article will introduce how to use React and Google BigQuery to build a fast data analysis application, and provide detailed code examples. 1. Overview React is a tool for building
