window.onload usage guide_javascript tips
The javascript script code in the web page often needs to be executed after the document is loaded. Otherwise, the object may not be obtained. In order to avoid this situation, you can use the following two methods:
1. Place the script code at the lower end of the web page, so that when running the script code, you can ensure that the object to be operated has been loaded.
2. Execute the script code through window.onload.
The first method feels messy (in fact, it is recommended). Often we need to place the script code in a more appropriate place, so the window.onload method is a better choice. window.onload is an event. This event will be triggered when the document is loaded. You can register an event handler for this event and put the script code to be executed in the event handler. This way you can avoid not getting the object. . Let’s look at a code example first:
<!DOCTYPE html> <html> <head> <meta charset=" utf-8"> <title>window.onload用法</title> <style type="text/css"> #bg{ width:100px; height:100px; border:2px solid red; } </style> <script type="text/javascript"> document.getElementById("bg").style.backgroundColor="#F90"; </script> </head> <body> <div id="bg"></div> </body> </html>
The original intention of the above code is to set the background color of the div to #F90, but it does not achieve this effect. This is because the code is executed sequentially. When it is executed to document.getElementById("#bg").style. When backgroundColor="#F90" is written, this div object has not been loaded yet, so the setting cannot be successful. The code is modified as follows:
<!DOCTYPE html> <html> <head> <meta charset=" utf-8"> <title>位置高度div垂直居中</title> <style type="text/css"> #bg{ width:100px; height:100px; border:2px solid red; } </style> <script type="text/javascript"> window.onload=function(){ document.getElementById("bg").style.backgroundColor="#F90"; } </script> </head> <body> <div id="bg"></div> </body> </html>
The reason is that the code for setting the background color is placed in the event processing function of window.onload. Only when the document is loaded, will the event processing function and the script code for setting the background color be executed.
Event handler function binding:
Method 1:
window.onload=function(){}, in the above code, this method is used to bind the event processing function to the window.onload event. What is bound here is an anonymous function. Of course, non-anonymous functions can also be bound. , the code example is as follows:
<!DOCTYPE html> <html> <head> <meta charset=" utf-8"> <title>window.onload用法详解</title> <script type="text/javascript"> window.onload=function myalert() { "); } </script> </head> <body> </body> </html>
The above code can bind the myalert method to the window.onload event, but cannot bind multiple event handlers to this event in the following way:
window.onload=function a(){}
window.onload=function b(){}
The above code cannot bind multiple event handling functions to the window.onload event, but the last one will overwrite all previous functions. But the code can be modified:
<!DOCTYPE html> <html> <head> <meta charset=" utf-8"> <title>window.onload用法</title> <style type="text/css"> #bg{ width:100px; height:100px; border:2px solid red; } </style> <script type="text/javascript"> window.onload=function(){ function a(){ document.getElementById("bg").style.backgroundColor="#F90"; } function b(){ document.getElementById("bg").style.width="200px"; } a(); b(); } </script> </head> <body> <div id="bg"></div> </body> </html>
The above code achieves the same effect of binding multiple event processing functions.
Method 2:
You can use addEventListener() and attachEvent() to bind event processing functions to the onload event. The following are introduced respectively:
addEventListener() is the current standard way to bind event processing functions, but browsers IE8 and below do not support this method. Examples are as follows:
<!DOCTYPE html> <html> <head> <meta charset=" utf-8"> <title>window.onload用法</title> <style type="text/css"> #bg{ width:100px; height:100px; border:2px solid red; } </style> <script type="text/javascript"> window.addEventListener("load",bg,false); window.addEventListener("load",changeW,false); function bg(){ document.getElementById("bg").style.backgroundColor="#F90"; } function changeW(){ document.getElementById("bg").style.width="200px"; } </script> </head> <body> <div id="bg"></div> </body> </html>
The above code can bind multiple event handlers to the window.onload event. A brief introduction to the syntax format:
addEventListener("type", function name, false)
The addEventListener() function has three parameters. The first parameter is the event type. It should be noted that there cannot be on in front of the event type name. For example, the window.onload event can only be written as load in this place. The second parameter is the name of the function to be bound, and the third parameter is generally false.
Use the attachEvent() function to bind the event processing function:
IE browsers before IE9 do not support the addEventListener() function, so the attachEvent() function comes into play. The code example is as follows:
<!DOCTYPE html> <html> <head> <meta charset=" utf-8"> <title>window.onload用法</title> <style type="text/css"> #bg{ width:100px; height:100px; border:2px solid red; } </style> <script type="text/javascript"> window.attachEvent("onload",bg); window.attachEvent("onload",changeW); function bg(){ document.getElementById("bg").style.backgroundColor="#F90"; } function changeW(){ document.getElementById("bg").style.width="200px"; } </script> </head> <body> <div id="bg"></div> </body> </html>
The effect of the above code is the same as that of using the addEventListener() function. Let’s briefly introduce the syntax format:
addEventListener("type", function name)
This function has only two parameters. The first parameter is the event type, but it has the same function as the first parameter of addEventListener(), but the name is different. The name has "on" in front of it. The first parameter is the name of the event handler function to be bound. In order to be compatible with various browsers, the above code needs to be modified. Examples are as follows:
<!DOCTYPE html> <html> <head> <meta charset=" utf-8"> <title>window.onload用法</title> <style type="text/css"> #bg{ width:100px; height:100px; border:2px solid red; } </style> <script type="text/javascript"> if(window.addEventListener){ window.addEventListener("load",bg,false); window.addEventListener("load",changeW,false); } else{ window.attachEvent("onload",bg); window.attachEvent("onload",changeW); } function bg(){ document.getElementById("bg").style.backgroundColor="#F90"; } function changeW(){ document.getElementById("bg").style.width="200px"; } </script> </head> <body> <div id="bg"></div> </body> </html>
The above code solves the compatibility issues of major browsers. Use the following code to judge in the above code:
if(object.addEventListener){ object.addEventListener(); } else{ object.attachEvent(); }
Use the if statement to determine whether the object has the addEventListener attribute. If so, use the addEventListener() function, otherwise use the attachEvent() function

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


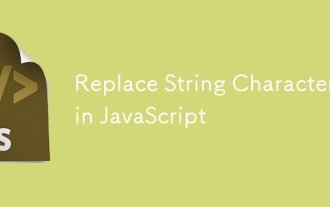
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
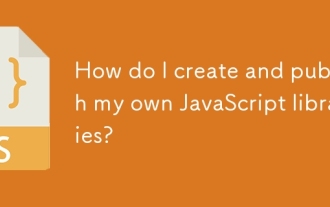
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
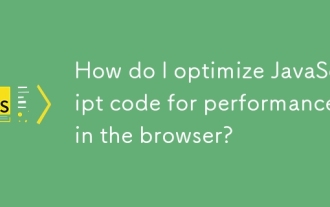
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
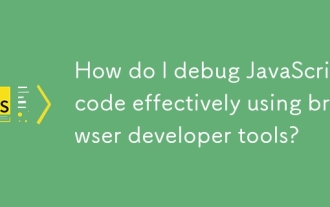
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
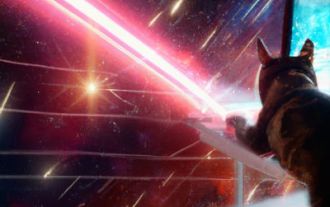
This article outlines ten simple steps to significantly boost your script's performance. These techniques are straightforward and applicable to all skill levels. Stay Updated: Utilize a package manager like NPM with a bundler such as Vite to ensure
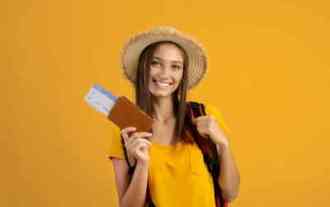
Sequelize is a promise-based Node.js ORM. It can be used with PostgreSQL, MySQL, MariaDB, SQLite, and MSSQL. In this tutorial, we will be implementing authentication for users of a web app. And we will use Passport, the popular authentication middlew
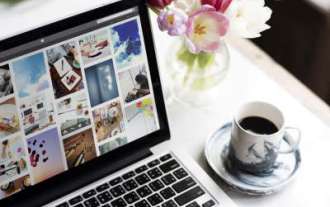
This article will guide you to create a simple picture carousel using the jQuery library. We will use the bxSlider library, which is built on jQuery and provides many configuration options to set up the carousel. Nowadays, picture carousel has become a must-have feature on the website - one picture is better than a thousand words! After deciding to use the picture carousel, the next question is how to create it. First, you need to collect high-quality, high-resolution pictures. Next, you need to create a picture carousel using HTML and some JavaScript code. There are many libraries on the web that can help you create carousels in different ways. We will use the open source bxSlider library. The bxSlider library supports responsive design, so the carousel built with this library can be adapted to any
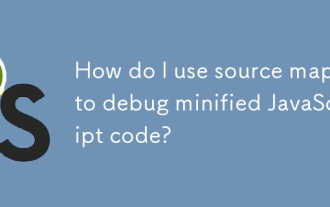
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
