How to modify golang map
Modification method: 1. Use the "map["key"]=value" statement to add or update elements. If "key" exists, update the element. If "key" does not exist, add the element. ; 2. Use the delete() function to delete the specified key-value pair from the map, the syntax is "delete(map, key name)"; 3. Re-create a new map object, which can clear all elements in the map, the syntax is "var mapname map[keytype]valuetype".
The operating environment of this tutorial: Windows 7 system, GO version 1.18, Dell G3 computer.
Map in Go language is a special data structure, an unordered collection of element pairs (pair). Pair corresponds to a key (index) and a value (value), so this structure is also called Associative array or dictionary is an ideal structure that can quickly find values. Given a key, the corresponding value can be quickly found.
map This data structure is also called dictionary (Python), hash, HashTable, etc. in other programming languages.
Introduction of map
[1] Map, a type built into the Go language, which relates key-value pairs Connection, we can get the corresponding value value through the key key. Similar to collections in other languages
[2]Basic syntax
var map变量名 map[keytype]valuetype
PS: Key and value types: bool, number, string, pointer, channel, and can also be Contains only the interfaces, structures, and arrays of the previous types
PS: key is usually int, string type, value is usually number (integer, floating point number), string, map, structure
PS: key: Slice, map, and function are not allowed
[3] Code:
Characteristics of map:
(1) Make
must be made before using the map collection (2) ) The key-value of the map is unordered
(3) The key cannot be repeated. If repeated, the latter value will replace the previous value
(4) The value can be repeated
package main import "fmt" func main(){ //定义map变量: var a map[int]string //只声明map内存是没有分配空间 //必须通过make函数进行初始化,才会分配空间: a = make(map[int]string,10) //map可以存放10个键值对 //将键值对存入map中: a[20095452] = "张三" a[20095387] = "李四" a[20097291] = "王五" a[20095387] = "朱六" a[20096699] = "张三" //输出集合 fmt.Println(a) }
Three ways to create a map
package main import "fmt" func main(){ //方式1: //定义map变量: var a map[int]string //只声明map内存是没有分配空间 //必须通过make函数进行初始化,才会分配空间: a = make(map[int]string,10) //map可以存放10个键值对 //将键值对存入map中: a[20095452] = "张三" a[20095387] = "李四" //输出集合 fmt.Println(a) //方式2: b := make(map[int]string) b[20095452] = "张三" b[20095387] = "李四" fmt.Println(b) //方式3: c := map[int]string{ 20095452 : "张三", 20098765 : "李四", } c[20095387] = "王五" fmt.Println(c) }
##Add, delete, modify and check the map
【1】Add and update operations:map["key"]= value ——》If the key does not exist, it is added, if the key exists, it is modified.
delete(map, "key") , delete is a built-in function. If the key exists, delete the key-value. If y of k does not exist, no operation will be performed, but no error will be reported
map = make(...), make a new one, and make the original one Become garbage and be recycled by gc
package main import "fmt" func main(){ //定义map b := make(map[int]string) //增加: b[20095452] = "张三" b[20095387] = "李四" //修改: b[20095452] = "王五" //删除: delete(b,20095387) delete(b,20089546) fmt.Println(b) //查找: value,flag := b[200] fmt.Println(value) fmt.Println(flag) }
package main import "fmt" func main(){ //定义map b := make(map[int]string) //增加: b[20095452] = "张三" b[20095387] = "李四" b[20098833] = "王五" //获取长度: fmt.Println(len(b)) //遍历: for k,v := range b { fmt.Printf("key为:%v value为%v \t",k,v) } fmt.Println("---------------------------") //加深难度: a := make(map[string]map[int]string) //赋值: a["班级1"] = make(map[int]string,3) a["班级1"][20096677] = "露露" a["班级1"][20098833] = "丽丽" a["班级1"][20097722] = "菲菲" a["班级2"] = make(map[int]string,3) a["班级2"][20089911] = "小明" a["班级2"][20085533] = "小龙" a["班级2"][20087244] = "小飞" for k1,v1:= range a { fmt.Println(k1) for k2,v2:= range v1{ fmt.Printf("学生学号为:%v 学生姓名为%v \t",k2,v2) } fmt.Println() } }
Go video tutorial、Programming teaching】
The above is the detailed content of How to modify golang map. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


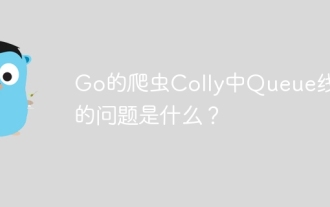
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
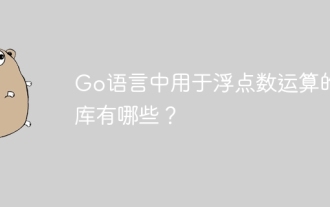
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
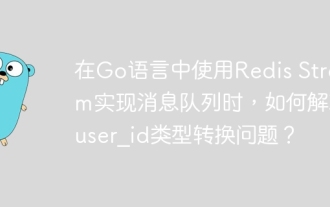
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
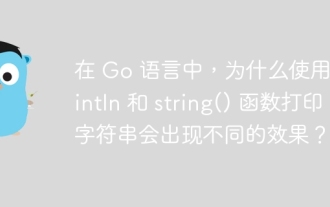
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
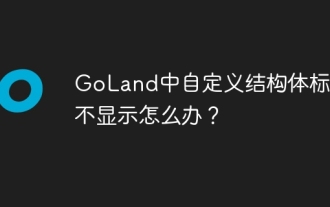
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
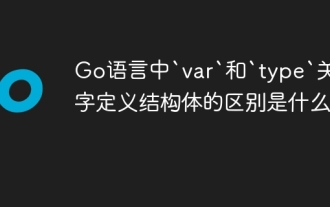
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
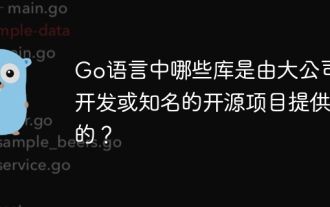
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
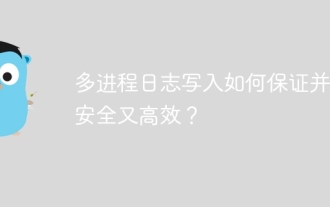
Efficiently handle concurrency security issues in multi-process log writing. Multiple processes write the same log file at the same time. How to ensure concurrency is safe and efficient? This is a...
