Can vue be adaptive?
vue can achieve self-adaptation. The methods to achieve self-adaptation are: 1. Install the "scale-box" component through the "npm install" or "yarn add" command, and use "scale-box" to implement self-adaptation. Equipped with zoom; 2. Adapt by setting the device pixel ratio; 3. Set the zoom attribute through JS to adjust the zoom ratio to achieve adaptation.
The operating environment of this tutorial: Windows 10 system, vue2&&vue3 version, Dell G3 computer.
Can vue be adaptive?
able.
Detailed explanation of the three implementation methods of Vue screen adaptation
Using the scale-box component
Attribute:
-
width
Width default1920
-
height
Height default1080
-
bgc
Background color default"transparent"
-
delay
Adaptive scaling anti-shake delay time (ms) Default100
vue2 Version: vue2 large screen adaptation scaling component (vue2-scale-box - npm)
npm install vue2-scale-box
or
yarn add vue2-scale-box
Usage:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
vue3 version: vue3 large screen Adapt scaling component (vue3-scale-box - npm)
npm install vue3-scale-box
or
yarn add vue3-scale-box
Usage:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
Set the device pixel ratio (device pixel ratio)
In the project Create a new devicePixelRatio.js file under utils
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
|
Introduce and use it in App.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
|
Set zoom attribute adjustment through JS Scaling ratio
Create a new monitorZoom.js file under the project's utils
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
Introduce and use it in main.js
1 2 3 4 5 6 7 |
|
Complete code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
Get the resolution of the screen
Get the width of the screen:
window.screen.width * window.devicePixelRatio
Get the height of the screen:
window.screen.height * window.devicePixelRatio
Mobile terminal adaptation (use postcss-px-to-viewport plug-in)
Official website:https://www.php.cn/link/2dd6d682870e39d9927b80f8232bd276
npm install postcss-px -to-viewport --save-dev
or
yarn add -D postcss-px-to-viewport
Configuration appropriate Configure the parameters of the plug-in (create a .postcssrc.js file in the project root directory [level with the src directory]) and paste the following code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
Recommended learning: "vue. js video tutorial》
The above is the detailed content of Can vue be adaptive?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


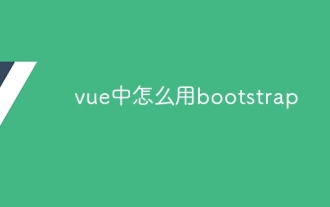
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
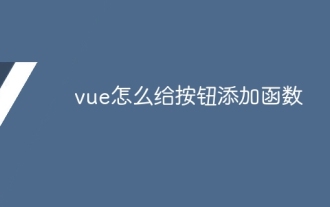
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
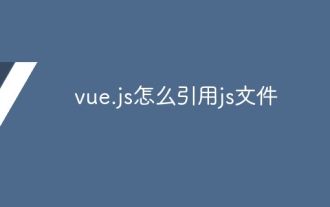
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
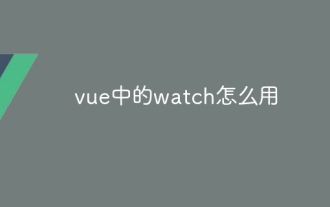
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
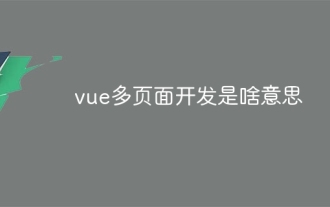
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
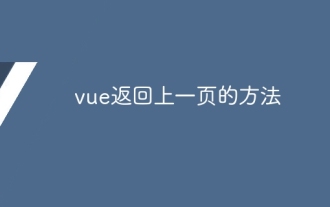
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
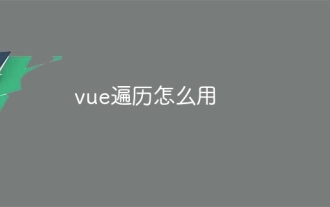
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
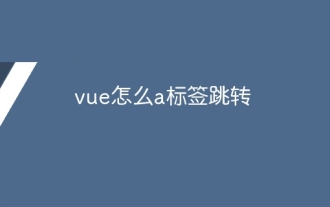
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
