How to implement the delete function in react
React method to implement the delete function: 1. Add a click event to the li tag, with code such as "
{value} "; 2. Use the list click event "handleItemClick(index) {...}" to implement the deletion function.
The operating environment of this tutorial: Windows 10 system, react18.0.0 version, Dell G3 computer.
How to implement the delete function in react?
React implements the TodoList deletion function
To realize clicking on an item in the list, delete the item
1. Add a click event to the li tag :handleItemClick
<li key= {index} onClick={this.handleItemClick.bind(this, index)}>{value}</li>
2. Click event function:
// 列表点击事件 handleItemClick(index) { const list = [...this.state.list]; list.splice(index, 1); this.setState({ list: list }) }
The complete code is as follows:
import React, {Component, Fragment} from 'react'; class TodoList extends Component { constructor(props) { super(props); this.state = { inputValue: '', list: [] } } handleInputChange(e) { this.setState({ inputValue: e.target.value }) } // 松开键盘会触发该事件 handleKeyUp(e) { // 判断是不是点的回车键 if (e.keyCode === 13) { const list = [...this.state.list, this.state.inputValue]; this.setState({ list: list, inputValue: '' }) } } // 列表点击事件 handleItemClick(index) { const list = [...this.state.list]; list.splice(index, 1); this.setState({ list: list }) } render() { return() } } export default TodoList; { this.state.list.map((value,index) => { return ( <li key= {index} onClick={this.handleItemClick.bind(this, index)}>{value}</li> ) }) }
Recommended learning: "react video tutorial"
The above is the detailed content of How to implement the delete function in react. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
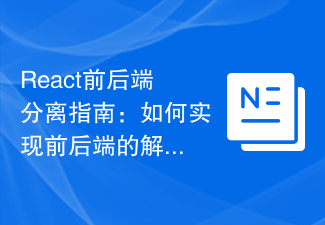
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
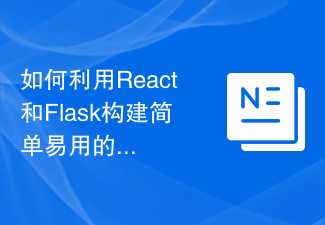
How to use React and Flask to build simple and easy-to-use web applications Introduction: With the development of the Internet, the needs of web applications are becoming more and more diverse and complex. In order to meet user requirements for ease of use and performance, it is becoming increasingly important to use modern technology stacks to build network applications. React and Flask are two very popular frameworks for front-end and back-end development, and they work well together to build simple and easy-to-use web applications. This article will detail how to leverage React and Flask
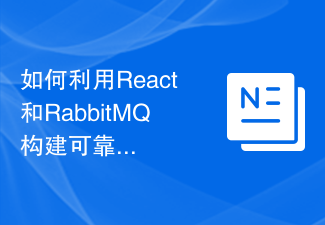
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
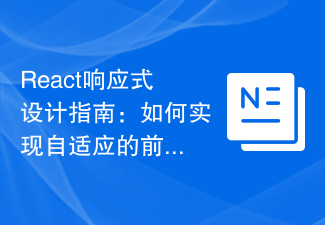
React Responsive Design Guide: How to Achieve Adaptive Front-end Layout Effects With the popularity of mobile devices and the increasing user demand for multi-screen experiences, responsive design has become one of the important considerations in modern front-end development. React, as one of the most popular front-end frameworks at present, provides a wealth of tools and components to help developers achieve adaptive layout effects. This article will share some guidelines and tips on implementing responsive design using React, and provide specific code examples for reference. Fle using React
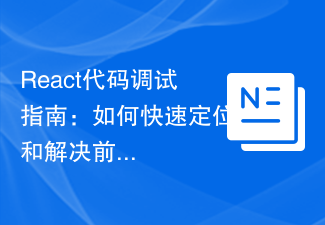
React code debugging guide: How to quickly locate and resolve front-end bugs Introduction: When developing React applications, you often encounter a variety of bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications. 1. Selection of debugging tools: In Re
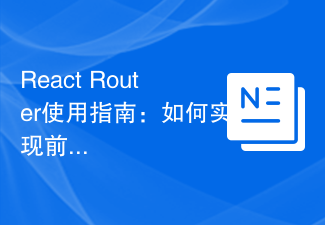
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
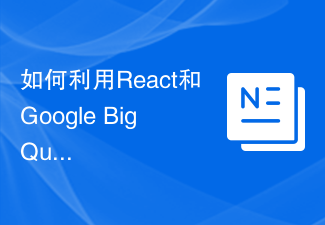
How to use React and Google BigQuery to build fast data analysis applications Introduction: In today's era of information explosion, data analysis has become an indispensable link in various industries. Among them, building fast and efficient data analysis applications has become the goal pursued by many companies and individuals. This article will introduce how to use React and Google BigQuery to build a fast data analysis application, and provide detailed code examples. 1. Overview React is a tool for building
