What is the difference between make and new in go language
Jan 09, 2023 am 11:44 AMDifference: 1. Make can only be used to allocate and initialize data of types slice, map, and chan; while new can allocate any type of data. 2. New allocation returns a pointer, which is the type "*Type"; while make returns a reference, which is Type. 3. The space allocated by new will be cleared; after make allocates the space, it will be initialized.
The operating environment of this tutorial: Windows 7 system, GO version 1.18, Dell G3 computer.
new and make are primitives for memory allocation in the Go language. Simply put, new only allocates memory, and make is used to initialize slices, maps, and channels.
new
new(T) function is a built-in function that allocates memory. It allocates a piece of memory for each type and initializes it to zero. value and returns its memory address.
The syntax is func new(Type) *Type
As we all know, an existing variable can be assigned to its pointer.
var p int var v *int v = &p *v = 11 fmt.Println(*v)
So what if it’s not a variable yet? Can you assign it directly?
func main() { var v *int *v = 8 fmt.Println(*v) // panic: runtime error: invalid memory address or nil pointer dereference // [signal SIGSEGV: segmentation violation code=0x1 addr=0x0 pc=0x47df36] // goroutine 1 [running]: // main.main() // /tmp/sandbox1410772957/prog.go:9 +0x16 }
The error result is as shown in the comments in the code.
How to solve? This can be solved by Go providing new initialization address.
func main() { var v *int // v 是一个 int 类型的指针,v 的地址和 v 的值 0xc0000ba018 <nil> fmt.Println("v 是一个 int 类型的指针,v 的地址和 v 的值 ", &v, v) // 分配给 v 一个指向的变量 v = new(int) // v 是一个 int 类型的指针,v 的地址和 v 的值 0xc0000ba018 0xc000018030 0,此时已经分配给了 v 指针一个指向的变量,但是变量为零值 fmt.Println("v 是一个 int 类型的指针,v 的地址, v 的值和 v 指向的变量的值 ", &v, v, *v) *v = 8 // v 是一个 int 类型的指针,v 的地址和 v 的值 0xc0000ba018 0xc000018030 8,此时又像这个变量中装填了一个值 8 fmt.Println("v 是一个 int 类型的指针,v 的地址, v 的值和 v 指向的变量的值 ", &v, v, *v) // 整个过程可以理解为给 v 指针指向了一个匿名变量 }
We can see that initializing a pointer variable with a value of nil is not a direct assignment. Return a pointer with a value of 0xc000018030 through new. points to the newly assigned int type, and zero value is its value.
Additionally, it is important to note that the zero value is different for different pointer types. For details, you can refer to this article. Or you can browse the code below.
type Name struct { P string } var av *[5]int var iv *int var sv *string var tv *Name av = new([5]int) fmt.Println(*av) //[0 0 0 0 0 0] iv = new(int) fmt.Println(*iv) // 0 sv = new(string) fmt.Println(*sv) // tv = new(Name) fmt.Println(*tv) //{}
The above describes how to assign values after processing ordinary types new(). Here is how to assign values after processing composite types (array, struct). But here, I think the author of the original article is wrong, because for slice, map and channel, new can only open
array instance
func main() { // 声明一个数组指针 var a *[5]int fmt.Printf("a: %p %#v \n", &a, a) //a: 0xc04200a180 [5]int{0, 0, 0, 0, 0} // 分配一个内存地址给 a(数组指针)指向 a = new([5]int) fmt.Printf("a: %p %#v \n", &a, a) //av: 0xc000074018 &[5]int{0, 0, 0, 0, 0} // 修改这个数组中的值 (*a)[1] = 8 fmt.Printf("a: %p %#v \n", &a, a) //av: 0xc000006028 &[5]int{0, 8, 0, 0, 0} }
structure instance
type mystruct struct { name string age int } func main() { var people *mystruct people = new(mystruct) people.name = "zhangsan" people.age = 11 fmt.Printf("%v, %v", people.name, people.age) // zhangsan, 11 }
make
make is specially used to create three types of content allocation: chan, map and slice, and can initialize them. The return type of make is the same type as its argument, not a pointer to it, because these three data types are themselves reference types.
The syntax is: func make(t Type, size ...IntegerType) Type
, you can see that the second parameter is a variable length parameter, used to specify the size of the allocated memory, such as For slice, cap and length need to be specified (cap represents capacity, length represents length, that is, the size that can be used), and cap is required to be larger than length.
I won’t introduce too much about the cap and length of slice here. You can understand that there is a house now. This house is a rough house. All its rooms have 3 rooms (cap), and they have been decorated. 1 room (length).
As for why not use new to allocate memory for these three types? Let's do an experiment.
func main() { var s *[]int fmt.Printf("s 的地址是: %p, s 的值是 %p\n", &s, s) // s 的地址是: 0xc00000e028, s 的值是 0x0 s = new([]int) fmt.Printf("s 的地址是: %p, s 的值是 %p\n", &s, s) // s 的地址是: 0xc00000e028, s 的值是 0xc00011a018 (*s)[0] = 1 fmt.Println("s 的地址是: %p, s 的值是 %p\n", &s, s) // panic: runtime error: index out of range [0] with length 0 } }
You can see that the length is 0 when assigning a value to the slice. As for the specific reason, friends who know it can leave a message in the comment area.
Therefore, it is often recommended to use make to perform these three types of creation.
slice instance
func main() { // 第一个 size 是 length,第二个 size 是 cap a := make([]int, 5, 10) // a: 0xc00011a018 []int{0, 0, 0, 0, 0},cap: 10, length: 5 fmt.Printf("a: %p %#v,cap: %d, length: %d \n", &a, a, cap(a), len(a)) }
map instance
func main() { // 第一个 string 是 key,第二个 string 是 value mapInstance := make(map[string]string, 5) mapInstance["第一名"] = "张三" mapInstance["第二名"] = "李四" mapInstance["第三名"] = "王五" fmt.Println(mapInstance) // map[第一名:张三 第三名:王五 第二名:李四] }
channel instance
func countNum(temp int, ch chan int) { i := temp + 1 ch <- i fmt.Println("已经将 i 发往通道 c 中") } func main() { ch := make(chan int) go countNum(1, ch) res := <-ch fmt.Println("已经从 ch 中获取 i 并保存在 res 中") fmt.Println("res 是", res) }
Summary:
The make function is only used for map, slice and channel, and does not return a pointer. If you want to get an explicit pointer, you can use the new function to allocate, or explicitly use the address of a variable.
The main differences between new and make in Go language are as follows:
make can only be used to allocate and initialize data of type slice, map, and chan; new can allocate Any type of data.
new allocation returns a pointer, which is the type *Type; make returns a reference, which is Type.
The space allocated by new is cleared; after make allocates the space, it will be initialized.
[Related recommendations: Go video tutorial, Programming teaching】
The above is the detailed content of What is the difference between make and new in go language. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
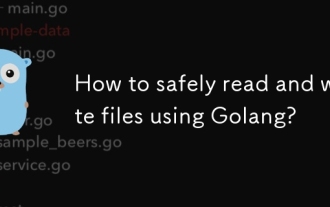
How to safely read and write files using Golang?
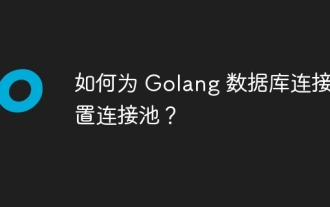
How to configure connection pool for Golang database connection?
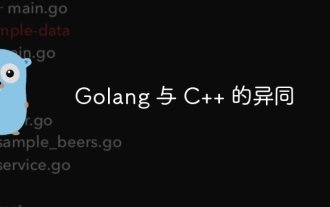
Similarities and Differences between Golang and C++
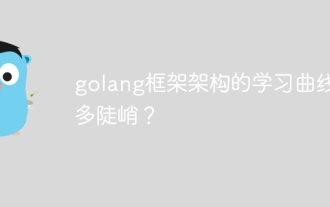
How steep is the learning curve of golang framework architecture?
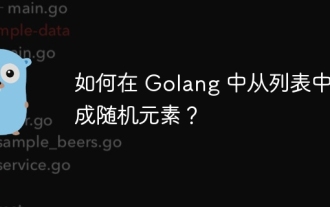
How to generate random elements from list in Golang?
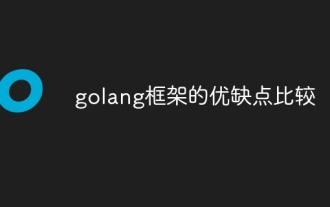
Comparison of advantages and disadvantages of golang framework

What are the best practices for error handling in Golang framework?
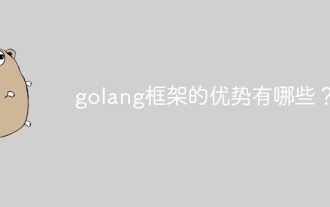
What are the advantages of golang framework?
