How to add nodes to tree in react
How to add nodes to the tree in react: 1. Introduce antd's tree control and implement tree-shaped components through "const treeData = [...]"; 2. Pass the key value of the current node , and then traverse the data array; 3. When traversing the array to add nodes, just add nodes through the query algorithm of nested array objects.
The operating environment of this tutorial: Windows 10 system, react18.0.0 version, Dell G3 computer.
How to add nodes to the tree in react?
The React project introduces antd's tree control to implement node addition, deletion and modification
Preface
When browsing some mainstream interview questions, I found that some interview questions asked me to write the addition, deletion and modification functions of a tree control based on antd. I originally thought that it was a component of antd. It should be pretty simple, but I didn’t expect that it took a lot of effort when I started doing it. So I plan to record the entire process of realizing the requirements.
1. Introducing antd's tree control
Everyone who has used antd should know that to use antd is to paste its code example, and then use it according to your own requirements and the API provided by antd. So here I am also looking for a simple antd tree control code example, no matter what, paste it in first and see what the result is.
import React from "react"; import { Tree } from "antd";const treeData = [ { title: "0-0", key: "0-0", children: [ { title: "0-0-0", key: "0-0-0", children: [ { title: "0-0-0-0", key: "0-0-0-0" }, { title: "0-0-0-1", key: "0-0-0-1" }, { title: "0-0-0-2", key: "0-0-0-2" }, ], }, { title: "0-0-1", key: "0-0-1", children: [ { title: "0-0-1-0", key: "0-0-1-0" }, { title: "0-0-1-1", key: "0-0-1-1" }, { title: "0-0-1-2", key: "0-0-1-2" }, ], }, { title: "0-0-2", key: "0-0-2", }, ], }, { title: "0-1", key: "0-1", children: [ { title: "0-1-0-0", key: "0-1-0-0" }, { title: "0-1-0-1", key: "0-1-0-1" }, { title: "0-1-0-2", key: "0-1-0-2" }, ], }, { title: "0-2", key: "0-2", },];export default function TreeDemo() { return ( <div> <tree></tree> </div> );}
A simple tree-shaped component is implemented here, but this component has basically no function except for browsing, so it needs to be reshaped.
2. Transform the component code structure
Generally when using antd components, the most important thing is to use the API provided by antd. Although we do not You know how to write it, but it should be pretty easy if you know how to use it. When browsing the API of the tree control, I found that the <tree></tree>
component has a sub-component <treenode></treenode>
, which is the smallest unit that makes up the entire tree control. So if we want to implement additions, deletions and modifications, we must work hard on this <treenode></treenode>
component. Based on this idea, it is then transformed into the following.
import React, { useState } from "react";import { Tree } from "antd";const { TreeNode } = Tree;const treeData = [ { value: "0", key: "0", children: [ { value: "0-1", key: "0-1", }, { value: "0-2", key: "0-2", }, ], },];export default function TreeDemo() { const [data, setdata] = useState(treeData); const renderTreeNodes = (data) => { let nodeArr = data.map((item) => { item.title = <span>{item.value}</span>; if (item.children) { return ( <treenode> {renderTreeNodes(item.children)} </treenode> ); } return <treenode></treenode>; }); return nodeArr; }; return ( <div> <tree>{renderTreeNodes(data)}</tree> </div> );}
Then it looks like the following:
The point to note here is that the title of <treenode></treenode>
receives ReactNode type data, so You can use this to define your own style for displaying data, such as adding icons and the like. Then at this point, everyone should know that the requirements say that there should be addition, deletion and modification functions. You can use this title to show it. The victory is right in front of you, and then you can transform the code.
import React, { useState } from "react";import { Tree } from "antd";import { EditOutlined, PlusOutlined, MinusOutlined, } from "@ant-design/icons";const { TreeNode } = Tree;const treeData = [ { value: "0", key: "0", children: [ { value: "0-1", key: "0-1", }, { value: "0-2", key: "0-2", }, ], },];export default function TreeDemo() { const [data, setdata] = useState(treeData); const renderTreeNodes = (data) => { let nodeArr = data.map((item) => { item.title = ( <div> <span>{item.value}</span> <span> <editoutlined></editoutlined> <plusoutlined></plusoutlined> <minusoutlined></minusoutlined> </span> </div> ); if (item.children) { return ( <treenode> {renderTreeNodes(item.children)} </treenode> ); } return <treenode></treenode>; }); return nodeArr; }; return ( <div> <tree>{renderTreeNodes(data)}</tree> </div> );}
Now there are three buttons, but the specific functions are still not available, so let’s do them one by one.
3. Implement the function of adding nodes
To add nodes, the method is to pass the key value of the current node and then traverse the data array. When traversing the array to add nodes, it involves the query algorithm of nested array objects, which uses the depth-first and breadth-first traversal ideas of arrays learned in the previous two weeks.
The following is the code modified based on this idea:
import React, { useState } from "react";import { Tree } from "antd";import { EditOutlined, PlusOutlined, MinusOutlined } from "@ant-design/icons";const { TreeNode } = Tree;const treeData = [ { value: "0", key: "0", children: [ { value: "0-1", key: "0-1", }, { value: "0-2", key: "0-2", }, ], },];export default function TreeDemo() { const [data, setdata] = useState(treeData); const renderTreeNodes = (data) => { let nodeArr = data.map((item) => { item.title = ( <div> <span>{item.value}</span> <span> <editoutlined></editoutlined> <plusoutlined>onAdd(item.key)} /> <minusoutlined></minusoutlined> </plusoutlined></span> </div> ); if (item.children) { return ( <treenode> {renderTreeNodes(item.children)} </treenode> ); } return <treenode></treenode>; }); return nodeArr; }; const onAdd = (key) => { addNode(key,treeData); //useState里数据务必为immutable (不可赋值的对象),所以必须加上slice()返回一个新的数组对象 setdata(treeData.slice()) }; const addNode = (key,data) => data.forEach((item) => { if (item.key === key) { if (item.children) { item.children.push({ value: "default", key: key + Math.random(100), // 这个 key 应该是唯一的 }); } else { item.children = []; item.children.push({ value: "default", key: key + Math.random(100), }); } return; } if (item.children) { addNode(key, item.children); } }); return ( <div> <tree>{renderTreeNodes(data)}</tree> </div> );}
Click the Add button to appear a new default node:
3. Implementation Edit node function
With the idea of adding nodes above, the subsequent editing and deletion functions will be easier to do. Only code snippets are shown here, and the final version of the code is at the end of the article. When editing a node, you need to make the node editable, so you need to use a variable to manage it.
const onEdit = (key) => { editNode(key, treeData); setData(treeData.slice()) }; const editNode = (key, data) => data.forEach((item) => { if (item.key === key) { item.isEditable = true; } else { item.isEditable = false; } item.value = item.defaultValue; // 当某节点处于编辑状态,并改变数据,点击编辑其他节点时,此节点变成不可编辑状态,value 需要回退到 defaultvalue if (item.children) { editNode(key, item.children); } });const treeData = [ { value: "0", key: "0", isEditable: false, children: [ { value: "0-1", key: "0-1", isEditable: false, }, { value: "0-2", key: "0-2", isEditable: false, }, ], },];
4. Implement the delete node function
const onDelete = (key) => { deleteNode(key, treeData); setData(treeData.slice()); }; const deleteNode = (key, data) => data.forEach((item, index) => { if (item.key === key) { data.splice(index, 1); return; } else { if (item.children) { deleteNode(key, item.children); } } });
5. Complete code
import React, { useState} from "react";import { Tree } from "antd";import { EditOutlined, PlusOutlined, MinusOutlined, CloseOutlined, CheckOutlined,} from "@ant-design/icons";import {nanoid} from "nanoid";const { TreeNode } = Tree;const treeData = [ { value: "0", defaultValue: "0", key: "0", parentKey: '0', isEditable: false, children: [ { value: "0-1", key: "0-1", defaultValue: "0-1", isEditable: false, }, { value: "0-2", key: "0-2", defaultValue: "0-2", isEditable: false, }, ], },];const expandedKeyArr = ["0"];export default function TreeDemo() { const [data, setData] = useState(treeData); const [expandedKeys, setExpandedKeys] = useState(expandedKeyArr); const onExpand = (expandedKeys) => { //记录折叠的key值 setExpandedKeys(expandedKeys); }; const renderTreeNodes = (data) => { let nodeArr = data.map((item) => { if (item.isEditable) { item.title = (onChange(e, item.key)} />); } else { item.title = (onClose(item.key, item.defaultValue)} /> onSave(item.key)} /> {item.value}); } if (item.children) { return (onEdit(item.key)} /> onAdd(item.key)} /> {item.parentKey === "0" ? null : ( onDelete(item.key)} /> )} {renderTreeNodes(item.children)} ); } return <treenode></treenode>; }); return nodeArr; }; const onAdd = (key) => { if (expandedKeys.indexOf(key) === -1) { expandedKeyArr.push(key); } setExpandedKeys(expandedKeyArr.slice()); addNode(key, treeData); //useState里数据务必为immutable (不可赋值的对象),所以必须加上slice()返回一个新的数组对象 setData(treeData.slice()); }; const onEdit = (key) => { editNode(key, treeData); setData(treeData.slice()); }; const editNode = (key, data) => data.forEach((item) => { if (item.key === key) { item.isEditable = true; } else { item.isEditable = false; } item.value = item.defaultValue; // 当某节点处于编辑状态,并改变数据,点击编辑其他节点时,此节点变成不可编辑状态,value 需要回退到 defaultvalue if (item.children) { editNode(key, item.children); } }); const addNode = (key, data) => data.forEach((item) => { if (item.key === key) { if (item.children) { item.children.push({ value: "default", key: nanoid(), // 这个 key 应该是唯一的 }); } else { item.children = []; item.children.push({ value: "default", key: nanoid(), }); } return; } if (item.children) { addNode(key, item.children); } }); const onChange = (e, key) => { changeNode(key, e.target.value, treeData); setData(treeData.slice()); }; const changeNode = (key, value, data) => data.forEach((item) => { if (item.key === key) { item.value = value; } if (item.children) { changeNode(key, value, item.children); } }); const onSave = (key) => { saveNode(key, treeData); setData(treeData.slice()); }; const saveNode = (key, data) => data.forEach((item) => { if (item.key === key) { item.defaultValue = item.value; } if (item.children) { saveNode(key, item.children); } item.isEditable = false; }); const onClose = (key, defaultValue) => { closeNode(key, defaultValue, treeData); setData(treeData); }; const closeNode = (key, defaultValue, data) => data.forEach((item) => { item.isEditable = false; if (item.key === key) { item.value = defaultValue; } if (item.children) { closeNode(key, defaultValue, item.children); } }); const onDelete = (key) => { deleteNode(key, treeData); setData(treeData.slice()); }; const deleteNode = (key, data) => data.forEach((item, index) => { if (item.key === key) { data.splice(index, 1); return; } else { if (item.children) { deleteNode(key, item.children); } } }); return ();}{renderTreeNodes(data)}
The above is the detailed content of How to add nodes to tree in react. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
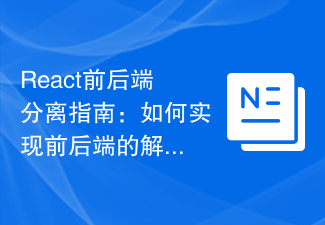
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
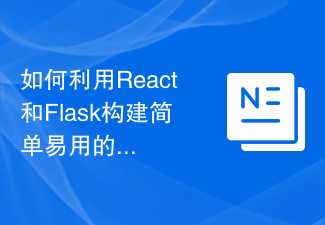
How to use React and Flask to build simple and easy-to-use web applications Introduction: With the development of the Internet, the needs of web applications are becoming more and more diverse and complex. In order to meet user requirements for ease of use and performance, it is becoming increasingly important to use modern technology stacks to build network applications. React and Flask are two very popular frameworks for front-end and back-end development, and they work well together to build simple and easy-to-use web applications. This article will detail how to leverage React and Flask
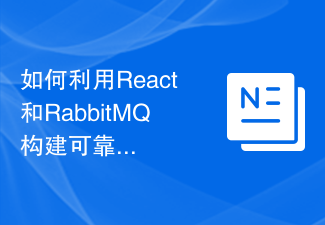
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
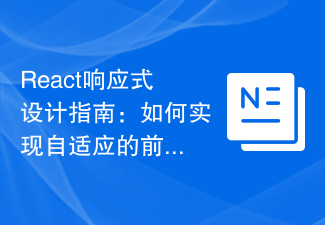
React Responsive Design Guide: How to Achieve Adaptive Front-end Layout Effects With the popularity of mobile devices and the increasing user demand for multi-screen experiences, responsive design has become one of the important considerations in modern front-end development. React, as one of the most popular front-end frameworks at present, provides a wealth of tools and components to help developers achieve adaptive layout effects. This article will share some guidelines and tips on implementing responsive design using React, and provide specific code examples for reference. Fle using React
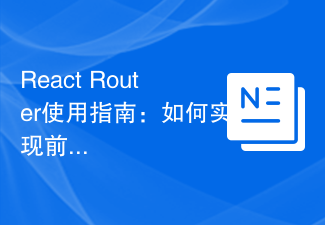
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
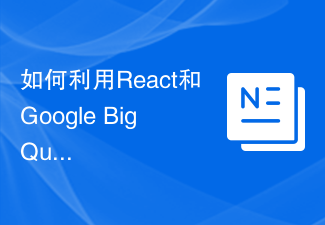
How to use React and Google BigQuery to build fast data analysis applications Introduction: In today's era of information explosion, data analysis has become an indispensable link in various industries. Among them, building fast and efficient data analysis applications has become the goal pursued by many companies and individuals. This article will introduce how to use React and Google BigQuery to build a fast data analysis application, and provide detailed code examples. 1. Overview React is a tool for building
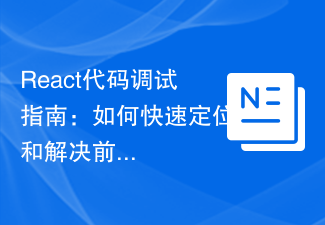
React code debugging guide: How to quickly locate and resolve front-end bugs Introduction: When developing React applications, you often encounter a variety of bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications. 1. Selection of debugging tools: In Re
