How to rename files in python
In python, you can use the rename() function of the os package to rename files (directories). The syntax "os.rename(src_path,dst_path)" can rename the file of src_path to dst_path. Because rename() is a function provided by the os package, if you want to use this function, you need to introduce the os package first, with the syntax "import os".
Yes Rename files
Directly paste the code:
import os
srcFile = './actwork/linkFile/allExtLinks - 副本.txt' dstFile = './actwork/linkFile/allExtLinks - copy.txt' try: os.rename(srcFile,dstFile) except Exception as e: print(e) print('rename file fail\r\n') else: print('rename file success\r\n')
Rename the files in the folder in order
When processing machine learning pictures, you need to put the pictures into different folders according to categories. In the folders, you also want to rename the pictures with increasing numbers to facilitate subsequent processing.
First, let’s take a look at python’s rename function:
os.rename(src,dst)
src
: The directory name to be modifieddst
: Modified directory name
If dst is an existing directory, OSError will be thrown.
Note: src and dst are both full path file names
First, let’s rename the folder
Use the simplest method to try it first
import os def myrename(path): file_list=os.listdir(path) i=0 for fi in file_list: old_name=os.path.join(path,fi) new_name=os.path.join(path,str(i)) os.rename(old_name,new_name) i+=1 if __name__=="__main__": path="D:/test/121" myrename(path)
We can see that the folders have been renamed in order
Slightly changed, it seems not so low
import os def myrename(path): file_list=os.listdir(path) for i,fi in enumerate(file_list): old_name=os.path.join(path,fi) new_name=os.path.join(path,"N0."+str(i)) os.rename(old_name,new_name) if __name__=="__main__": path="D:/test/121" myrename(path)
The effect is the same, the enumerate function no longer has the effect To go into details, we can also use python zip to make modifications:
import os def myrename(path): file_list=os.listdir(path) for i,fi in zip(range(len(file_list)),file_list): old_name=os.path.join(path,fi) new_name=os.path.join(path,"The."+str(i)) os.rename(old_name,new_name) if __name__=="__main__": path="D:/test/121" myrename(path)
The effect is the same:
## Next we Rename files
The above three methods can all be used, just need to be slightly modified. I will use the function enumerate as an example to demonstrate:Create a text document first
Copy and paste a bunch of them for testing. Add a try-except to the function to prevent file renaming errors. Here The rename needs to be a file in the same format, otherwise an error will be reported:import os def myrename(path): file_list=os.listdir(path) for i,fi in enumerate(file_list): old_dir=os.path.join(path,fi) filename="my"+str(i+1)+"."+str(fi.split(".")[-1]) new_dir=os.path.join(path,filename) try: os.rename(old_dir,new_dir) except Exception as e: print(e) print("Failed!") else: print("SUcess!") if __name__=="__main__": path="D:/test/121" myrename(path)
##[Related recommendations:
The above is the detailed content of How to rename files in python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


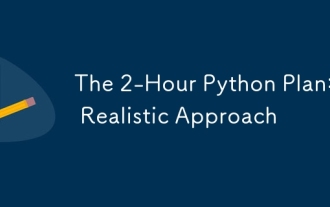
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
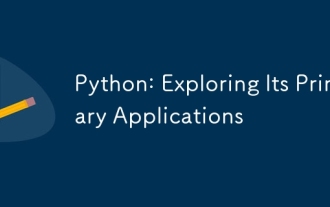
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
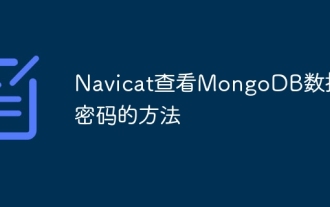
It is impossible to view MongoDB password directly through Navicat because it is stored as hash values. How to retrieve lost passwords: 1. Reset passwords; 2. Check configuration files (may contain hash values); 3. Check codes (may hardcode passwords).
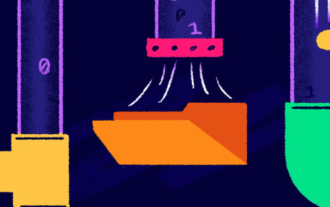
As a data professional, you need to process large amounts of data from various sources. This can pose challenges to data management and analysis. Fortunately, two AWS services can help: AWS Glue and Amazon Athena.
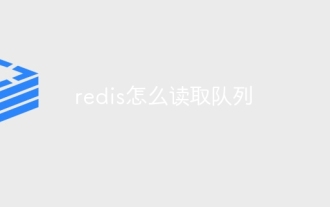
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
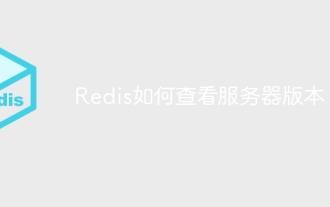
Question: How to view the Redis server version? Use the command line tool redis-cli --version to view the version of the connected server. Use the INFO server command to view the server's internal version and need to parse and return information. In a cluster environment, check the version consistency of each node and can be automatically checked using scripts. Use scripts to automate viewing versions, such as connecting with Python scripts and printing version information.
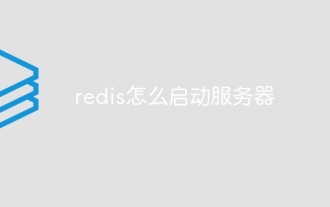
The steps to start a Redis server include: Install Redis according to the operating system. Start the Redis service via redis-server (Linux/macOS) or redis-server.exe (Windows). Use the redis-cli ping (Linux/macOS) or redis-cli.exe ping (Windows) command to check the service status. Use a Redis client, such as redis-cli, Python, or Node.js, to access the server.
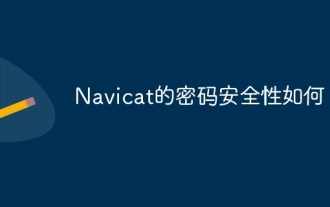
Navicat's password security relies on the combination of symmetric encryption, password strength and security measures. Specific measures include: using SSL connections (provided that the database server supports and correctly configures the certificate), regularly updating Navicat, using more secure methods (such as SSH tunnels), restricting access rights, and most importantly, never record passwords.
