Teach you step by step how to write a player using Vue3
This article brings you relevant knowledge about Vue3. It mainly talks about how to write a player with Vue3. Friends who are interested can take a look at it together. I hope Helpful to everyone.
ps: Music may fail to play. The reason is that the audio link is temporary and can be replaced manually.
#TODO
- Realize play/pause;
- Realize start/end time, start time and scroll bar to dynamically change with the playback;
- Realize click on the progress bar to jump to the specified playback Position;
- Click the dot to drag the scroll bar.
css The style is as follows
<template> <div class="song-item"> <audio src="" /> <!-- 进度条 --> <div class="audio-player"> <span>00:00</span> <div class="progress-wrapper"> <div class="progress-inner"> <div class="progress-dot" /> </div> </div> <span>00:00</span> <!-- 播放/暂停 --> <div style="margin-left: 10px; color: #409eff; cursor: pointer;" > 播放 </div> </div> </div></template><style lang="scss"> * { font-size: 14px; } .song-item { display: flex; flex-direction: column; justify-content: center; height: 100px; padding: 0 20px; transition: all ease .2s; border-bottom: 1px solid #ddd; /* 进度条样式 */ .audio-player { display: flex; height: 18px; margin-top: 10px; align-items: center; font-size: 12px; color: #666; .progress-wrapper { flex: 1; height: 4px; margin: 0 20px 0 20px; border-radius: 2px; background-color: #e9e9eb; cursor: pointer; .progress-inner { position: relative; width: 0%; height: 100%; border-radius: 2px; background-color: #409eff; .progress-dot { position: absolute; top: 50%; right: 0; z-index: 1; width: 10px; height: 10px; border-radius: 50%; background-color: #409eff; transform: translateY(-50%); } } } } }</style>
Idea: Register click event for "Play" , in the click event, use the attributes and methods ofFinally apply the attributes and events to the template.audio
Key api:to determine the status of the current song, whether to play or pause, then declare an attribute to synchronize this status, and make a judgment in the template that the current song should display "Play/ pause".
audio.paused
: Whether the current player is paused
audio.play()
:Play
audio.pause()
:Pause
const audioIsPlaying = ref(false); // 用于同步当前的播放状态const audioEle = ref<HTMLAudioElement | null>(null); // audio 元素/** * @description 播放/暂停音乐 */const togglePlayer = () => { if (audioEle.value) { if (audioEle.value?.paused) { audioEle.value.play(); audioIsPlaying.value = true; } else { audioEle.value?.pause(); audioIsPlaying.value = false; } } };onMounted(() => { // 页面点击的时候肯定是加载完成了,这里获取一下没毛病 audioEle.value = document.querySelector('audio'); });Copy after login
<div style="margin-left: 10px; color: #409eff; cursor: pointer;" @click="togglePlayer"> {{ audioIsPlaying ? '暂停' : '播放'}}</div>
Idea: Get the current played time and total duration, and then get the current duration/ The total duration and the percentage of the song played are the percentage of the scroll bar. By listening to theRealize clicking on the progress bar to jump to the specified playback positiontimeupdate
Key api:event of the
audioelement, every time the current time changes, the DOM is also updated synchronously. After the final playback is completed, the state is initialized.
audio.currentTime
: Current playback time; unit (s)
audio.duration
: The total duration of the audio; unit (s)
timeupdate
:
currentTimeThis event will be triggered when it changes.
import dayjs from 'dayjs';const audioCurrentPlayTime = ref('00:00'); // 当前播放时长const audioCurrentPlayCountTime = ref('00:00'); // 总时长const pgsInnerEle = ref<HTMLDivElement | null>(null);/** * @description 更新进度条与当前播放时间 */const updateProgress = () => { const currentProgress = audioEle.value!.currentTime / audioEle.value!.duration; pgsInnerEle.value!.style.width = `${currentProgress * 100}%`; // 设置进度时长 if (audioEle.value) audioCurrentPlayTime.value = dayjs(audioEle.value.currentTime * 1000).format('mm:ss'); };/** * @description 播放完成重置播放状态 */const audioPlayEnded = () => { audioCurrentPlayTime.value = '00:00'; pgsInnerEle.value!.style.width = '0%'; audioIsPlaying.value = false; };onMounted(() => { pgsInnerEle.value = document.querySelector('.progress-inner'); // 设置总时长 if (audioEle.value) audioCurrentPlayCountTime.value = dayjs(audioEle.value.duration * 1000).format('mm:ss'); // 侦听播放中事件 audioEle.value?.addEventListener('timeupdate', updateProgress, false); // 播放结束 event audioEle.value?.addEventListener('ended', audioPlayEnded, false); });Copy after login
Idea: register a mouse click event for the scroll bar, and obtain the currentClick the dot to drag the scroll bar.offsetX each time it is clicked
Key api:And the width of the scroll bar, use width /
offsetXFinally, use the total duration * The previous quotient will get the progress we want, just update the progress bar again.
event.offsetX
: The x coordinate of the mouse pointer compared to the triggering event object.
/** * @description 点击滚动条同步更新音乐进度 */const clickProgressSync = (event: MouseEvent) => { if (audioEle.value) { // 保证是正在播放或者已经播放的状态 if (!audioEle.value.paused || audioEle.value.currentTime !== 0) { const pgsWrapperWidth = pgsWrapperEle.value!.getBoundingClientRect().width; const rate = event.offsetX / pgsWrapperWidth; // 同步滚动条和播放进度 audioEle.value.currentTime = audioEle.value.duration * rate; updateProgress(); } } };onMounted({ pgsWrapperEle.value = document.querySelector('.progress-wrapper'); // 点击进度条 event pgsWrapperEle.value?.addEventListener('mousedown', clickProgressSync, false); });// 别忘记统一移除侦听onBeforeUnmount(() => { audioEle.value?.removeEventListener('timeupdate', updateProgress); audioEle.value?.removeEventListener('ended', audioPlayEnded); pgsWrapperEle.value?.removeEventListener('mousedown', clickProgressSync); });Copy after login
Idea: UseFinally call thishook
When clicked: Get theto manage this dragging function and listen to the mouse click, mouse movement, and mouse lift events of this scroll bar.
x
When moving: Get thecoordinates of the mouse in the window, the
leftdistance of the dot from the window and the maximum right movement distance (scroll bar width - dot distance from the window of
left). In order to prevent the mobile from starting calculation casually, you can set a switch at the beginning
flagx
is lifted :Resetcoordinates of the mouse in the window in real time minus the click time Get the
xcoordinates. Then make a judgment based on the maximum moving distance and don't let it go out of bounds. Finally: Total duration* (
xcalculated from the distance between the dot and the
leftof the window / length of the scroll bar) The percentage is obtained to update the scroll bar and progress when
flag
.
/** * @method useSongProgressDrag * @param audioEle * @param pgsWrapperEle * @param updateProgress 更新滚动条方法 * @param startSongDragDot 是否开启拖拽滚动 * @description 拖拽更新歌曲播放进度 */const useSongProgressDrag = ( audioEle: Ref<HTMLAudioElement | null>, pgsWrapperEle: Ref<HTMLDivElement | null>, updateProgress: () => void, startSongDragDot: Ref<boolean>) => { const audioPlayer = ref<HTMLDivElement | null>(null); const audioDotEle = ref<HTMLDivElement | null>(null); const dragFlag = ref(false); const position = ref({ startOffsetLeft: 0, startX: 0, maxLeft: 0, maxRight: 0, }); /** * @description 鼠标点击 audioPlayer */ const mousedownProgressHandle = (event: MouseEvent) => { if (audioEle.value) { if (!audioEle.value.paused || audioEle.value.currentTime !== 0) { dragFlag.value = true; position.value.startOffsetLeft = audioDotEle.value!.offsetLeft; position.value.startX = event.clientX; position.value.maxLeft = position.value.startOffsetLeft; position.value.maxRight = pgsWrapperEle.value!.offsetWidth - position.value.startOffsetLeft; } } event.preventDefault(); event.stopPropagation(); }; /** * @description 鼠标移动 audioPlayer */ const mousemoveProgressHandle = (event: MouseEvent) => { if (dragFlag.value) { const clientX = event.clientX; let x = clientX - position.value.startX; if (x > position.value.maxRight) x = position.value.maxRight; if (x < -position.value.maxLeft) x = -position.value.maxLeft; const pgsWidth = pgsWrapperEle.value?.getBoundingClientRect().width; const reat = (position.value.startOffsetLeft + x) / pgsWidth!; audioEle.value!.currentTime = audioEle.value!.duration * reat; updateProgress(); } }; /** * @description 鼠标取消点击 */ const mouseupProgressHandle = () => dragFlag.value = false; onMounted(() => { if (startSongDragDot.value) { audioPlayer.value = document.querySelector('.audio-player'); audioDotEle.value = document.querySelector('.progress-dot'); // 在捕获中去触发点击 dot 事件. fix: 点击原点 offset 回到原点 bug audioDotEle.value?.addEventListener('mousedown', mousedownProgressHandle, true); audioPlayer.value?.addEventListener('mousemove', mousemoveProgressHandle, false); document.addEventListener('mouseup', mouseupProgressHandle, false); } }); onBeforeUnmount(() => { if (startSongDragDot.value) { audioPlayer.value?.removeEventListener('mousedown', mousedownProgressHandle); audioPlayer.value?.removeEventListener('mousemove', mousemoveProgressHandle); document.removeEventListener('mouseup', mouseupProgressHandle); } }); };Copy after login
hook
// 是否显示可拖拽 dot// 可以在原点元素上增加 v-if 用来判定是否需要拖动功能const startSongDragDot = ref(true);useSongProgressDrag(audioEle, pgsWrapperEle, updateProgress, startSongDragDot);
vue.js video tutorial]
The above is the detailed content of Teach you step by step how to write a player using Vue3. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




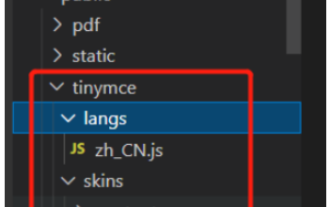
tinymce is a fully functional rich text editor plug-in, but introducing tinymce into vue is not as smooth as other Vue rich text plug-ins. tinymce itself is not suitable for Vue, and @tinymce/tinymce-vue needs to be introduced, and It is a foreign rich text plug-in and has not passed the Chinese version. You need to download the translation package from its official website (you may need to bypass the firewall). 1. Install related dependencies npminstalltinymce-Snpminstall@tinymce/tinymce-vue-S2. Download the Chinese package 3. Introduce the skin and Chinese package. Create a new tinymce folder in the project public folder and download the
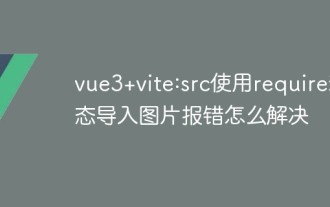
vue3+vite:src uses require to dynamically import images and error reports and solutions. vue3+vite dynamically imports multiple images. If vue3 is using typescript development, require will introduce image errors. requireisnotdefined cannot be used like vue2 such as imgUrl:require(' .../assets/test.png') is imported because typescript does not support require, so import is used. Here is how to solve it: use awaitimport
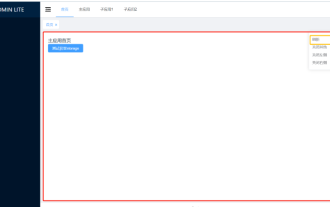
To achieve partial refresh of the page, we only need to implement the re-rendering of the local component (dom). In Vue, the easiest way to achieve this effect is to use the v-if directive. In Vue2, in addition to using the v-if instruction to re-render the local dom, we can also create a new blank component. When we need to refresh the local page, jump to this blank component page, and then jump back in the beforeRouteEnter guard in the blank component. original page. As shown in the figure below, how to click the refresh button in Vue3.X to reload the DOM within the red box and display the corresponding loading status. Since the guard in the component in the scriptsetup syntax in Vue3.X only has o
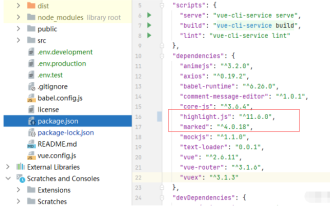
Vue implements the blog front-end and needs to implement markdown parsing. If there is code, it needs to implement code highlighting. There are many markdown parsing libraries for Vue, such as markdown-it, vue-markdown-loader, marked, vue-markdown, etc. These libraries are all very similar. Marked is used here, and highlight.js is used as the code highlighting library. The specific implementation steps are as follows: 1. Install dependent libraries. Open the command window under the vue project and enter the following command npminstallmarked-save//marked to convert markdown into htmlnpmins
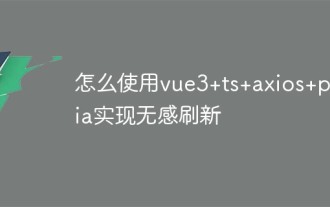
vue3+ts+axios+pinia realizes senseless refresh 1. First download aiXos and pinianpmipinia in the project--savenpminstallaxios--save2. Encapsulate axios request-----Download js-cookienpmiJS-cookie-s//Introduce aixosimporttype{AxiosRequestConfig ,AxiosResponse}from"axios";importaxiosfrom'axios';import{ElMess
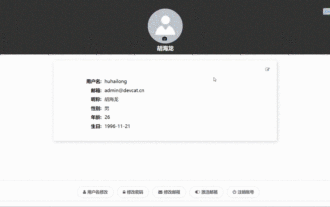
The final effect is to install the VueCropper component yarnaddvue-cropper@next. The above installation value is for Vue3. If it is Vue2 or you want to use other methods to reference, please visit its official npm address: official tutorial. It is also very simple to reference and use it in a component. You only need to introduce the corresponding component and its style file. I do not reference it globally here, but only introduce import{userInfoByRequest}from'../js/api' in my component file. import{VueCropper}from'vue-cropper&
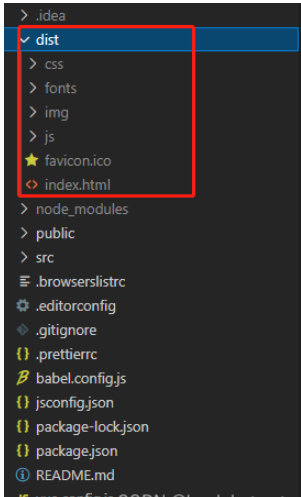
After the vue3 project is packaged and published to the server, the access page displays blank 1. The publicPath in the vue.config.js file is processed as follows: const{defineConfig}=require('@vue/cli-service') module.exports=defineConfig({publicPath :process.env.NODE_ENV==='production'?'./':'/&
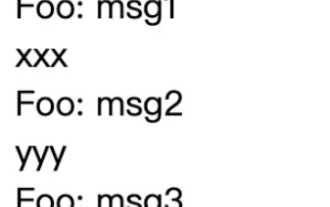
Preface Whether it is vue or react, when we encounter multiple repeated codes, we will think about how to reuse these codes instead of filling a file with a bunch of redundant codes. In fact, both vue and react can achieve reuse by extracting components, but if you encounter some small code fragments and you don’t want to extract another file, in comparison, react can be used in the same Declare the corresponding widget in the file, or implement it through renderfunction, such as: constDemo:FC=({msg})=>{returndemomsgis{msg}}constApp:FC=()=>{return(
