What are WaitGroups in go language? how to use?
What are WaitGroups? The following article will take you to understand WaitGroups in the go language and introduce how to use WaitGroups. I hope it will be helpful to you!
#What are WaitGroups?
WaitGroups
is an efficient way to synchronize your goroutines. Imagine you are traveling by car with your family. Your dad stops at a strip mall or fast food restaurant to buy some food and use the bathroom. You'd better want to wait until everyone gets back before driving to Horizon. WaitGroups
helps you do this.
WaitGroups
is defined by calling the sync
package in the standard library.
var wg sync.WaitGroup
So, what is WaitGroup
? WaitGroup
is a structure that contains certain information about how many goroutine
the program needs to wait for. It is a group containing the number of goroutines
you need to wait for.
WaitGroups has three most important methods: Add
, Done
and Wait
.
- Add: Add to the total number of goroutines you need to wait for.
- Done: Subtract one from the total number of goroutines you need to wait for.
- Wait: Blocks the code from continuing until there are no more goroutines to wait for.
How to use WaitGroups
Let’s take a look at a piece of code:
package main import ( "fmt" "sync" "time" ) func main() { var wg sync.WaitGroup wg.Add(1) go func() { defer wg.Done() fmt.Println(time.Now(), "start") time.Sleep(time.Second) fmt.Println(time.Now(), "done") }() wg.Wait() fmt.Println(time.Now(), "exiting...") }
2022-08-21 17:01:54.184744229 +0900 KST m=+0.000021800 start 2022-08-21 17:01:55.184932851 +0900 KST m=+1.000210473 done 2022-08-21 17:01:55.18507731 +0900 KST m=+1.000354912 exiting...
- We first initialize a
WaitGroup Instance of wg
. - Then we add 1 to
wg
because we want to wait for agoroutine
to complete. - Then we run this
goroutine
. Inside thegoroutine
, we make a delayed call towg.Done()
to ensure that we decrement the number ofgoroutine
to wait for. If we don't do this, then the code will wait forever for thegoroutine
to complete and will cause a deadlock. - After the
goroutine
call, we want to make sure to block the code untilWaitGroup
is empty. We do this by callingwg.Wait()
.
Why use WaitGroups instead of channels?
Now that we know how to use WaitGroups, a natural thought leads us to this question: Why use WaitGroups instead of channels?
Based on my experience, there are several reasons.
WaitGroups
tends to be more intuitive. When you read a piece of code, when you see aWaitGroup
, you immediately know what the code is doing. The method names are clear and get to the point. However, with channels, sometimes it's not so clear. Using channels is smart, but when you read a complex piece of code, it can be cumbersome to understand.- Sometimes, you don't need to use channels. For example, let's take a look at this code:
var wg sync.WaitGroup for i := 0; i < 5; i++ { wg.Add(1) go func() { defer wg.Done() fmt.Println(time.Now(), "start") time.Sleep(time.Second) fmt.Println(time.Now(), "done") }() } wg.Wait() fmt.Println(time.Now(), "exiting...")
You can see that this goroutine
does not communicate data with other goroutine
. If your goroutine
is a one-time job and you don't need to know the result, using WaitGroup
is preferable. Now look at this code:
ch := make(chan int) for i := 0; i < 5; i++ { go func() { randomInt := rand.Intn(10) ch <- randomInt }() } for i := 0; i < 5; i++ { fmt.Println(<-ch) }
Here, goroutine
is sending data to channel
. In these cases we don't need to use WaitGroup
as that would be redundant. If the receive has already done enough blocking, why wait for the goroutine
to complete?
WaitGroups
is specially used to handle waiting for goroutines
. I think the main purpose of channels is to communicate data. You can't use WaitGroup
to send and receive data, but you can use a channel
to synchronize your goroutines
.
Finally, there is no right answer. I know this can be annoying, but it depends on you and the team you work for. Whatever method is best, no answer is wrong. I personally prefer to use WaitGroups
for synchronization, but your situation may be different. Choose what feels most intuitive to you.
One thing to note
Sometimes, you may need to pass a WaitGroup
instance to a goroutine
. There may be several WaitGroup
to handle different goroutine
, or it may be a design choice. Whatever the reason, make sure to pass a pointer to WaitGroup
, like this:
var wg sync.WaitGroup for i := 0; i < 5; i++ { wg.Add(1) go func(wg *sync.WaitGroup) { defer wg.Done() fmt.Println(time.Now(), "start") time.Sleep(time.Second) fmt.Println(time.Now(), "done") }(&wg) } wg.Wait() fmt.Println(time.Now(), "exiting...")
The reason is that Go is a pass-by-value language. This means that whenever you pass an argument to a function, Go copies the argument and passes it instead of the original object. What happens in this case is that the entire WaitGroup
object will be copied, which means that the goroutine
will handle a completely different WaitGroup. wg.Done()
does not subtract from the original wg, but subtracts a copy of it, which only exists in goroutine
.
Summary
By using WaitGroups
, we can easily synchronize goroutines
, ensuring that our code is executed at the correct time. Although channels can also be used for synchronization, WaitGroups
are generally more intuitive and easier to read. When using WaitGroup
, be sure to pass the pointer to WaitGroup
correctly to prevent copy issues. No matter which method you choose, choose the one that's most intuitive and works best for you and your team.
Recommended learning: Golang tutorial
The above is the detailed content of What are WaitGroups in go language? how to use?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


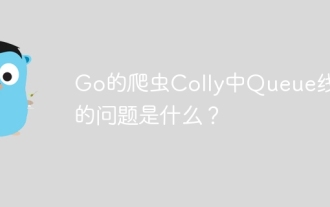
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
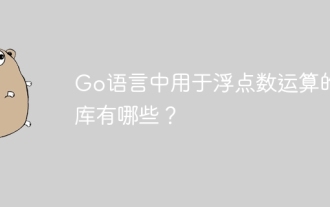
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
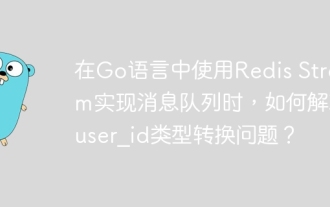
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
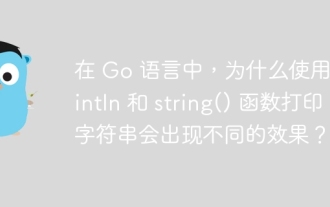
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
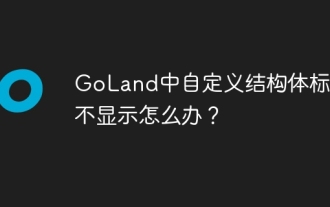
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
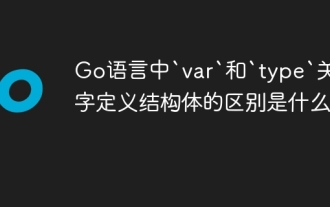
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
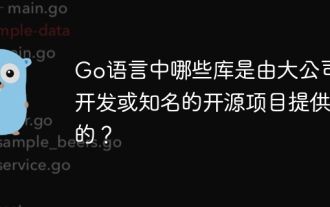
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
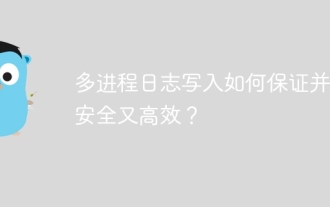
Efficiently handle concurrency security issues in multi-process log writing. Multiple processes write the same log file at the same time. How to ensure concurrency is safe and efficient? This is a...
