What are methods in PHP? How to define and use it?
PHP is a very popular programming language, especially in the world of web development. Its power and flexibility make PHP one of the preferred languages for developers. Among them, the definition method is one of the most basic concepts in PHP programming and one of the most commonly used functions by developers. This article will introduce the syntax and usage of defining methods in PHP.
First, we need to understand what a method is. In programming, a method refers to a group of related codes that are organized together so that they can be executed when needed. These codes can access some specific data and operate on them in a predetermined way. In PHP, methods are also called functions. We can use functions to perform some specific operations and pass parameters through functions to achieve different inputs and outputs.
The basic syntax for defining methods in PHP is as follows:
function functionName($parameter1, $parameter2, …) { // 函数体 return $returnValue; }
Among them, functionName represents the function name, which needs to be used to reference the method when the function is called. $parameter1, $parameter2, … represent the parameter list of the method, and these parameters identify the data input to the function. The function body is the actual execution content of the defined method. Finally, the return statement is used to specify the return value of the function.
Methods in PHP can take many different parameter types. The most common are scalar data types such as strings, integers, and floating point numbers. In addition, you can also use arrays and objects as function parameters and return values. Parameters in PHP can also be passed by reference, which means functions can modify the value of the variable passed to them.
Here are some examples of methods defined in PHP:
function add($a, $b) { return $a + $b; } function helloWorld() { echo "Hello, World!"; } function sayHello($name) { echo "Hello, " . $name . "!"; } $myArray = array("one", "two", "three"); function countArray($array) { return count($array); } class Person { public $name; public function sayHello() { echo "Hello, " . $this->name . "!"; } } function addOne(&$value) { $value++; }
In the above example, we have defined some different methods and functions. The add() method accepts two numeric arguments and returns their sum. The helloWorld() method does not accept any parameters and only prints out the "Hello, World!" statement. The sayHello() method accepts a string parameter and outputs a statement like "Hello, {name}!" The countArray() method uses the count() function to get the number of elements in an array. The sayHello() method in the Person class uses the object property $name to output the name of the instantiated object. Finally, the addOne() method accepts a reference parameter and adds one to that parameter.
Now we can use these methods. In order to call these methods, we simply use the method name and pass them the correct parameters. For example, we can use the following code to call some of the above methods:
echo add(2, 3); // 输出 5 helloWorld(); // 输出 "Hello, World!" sayHello("Alice"); // 输出 "Hello, Alice!" echo countArray($myArray); // 输出 3 $person = new Person(); $person->name = "Bob"; $person->sayHello(); // 输出 "Hello, Bob!" $value = 1; addOne($value); echo $value; // 输出 2
In the above code, we first call the add() method, passing 2 and 3 to it, and print out the result 5. Next we call the helloWorld() method and print out "Hello, World!". We use the sayHello() method to print out "Hello, Alice!". We then call the countArray() method and pass an array argument to print out 3. Then, we create a Person object, set its name property to Bob, and call its sayHello() method to print out "Hello, Bob!". Finally, we use the addOne() method and pass the reference parameter, increment the parameter's value from 1 to 2, and print out 2.
To summarize, defining methods in PHP is a very basic programming concept. We can use methods to perform some specific operations and flexibly pass parameters and return values. It is very important for web developers to be proficient in the syntax and usage of defined methods in PHP. Whether you are writing simple scripts or building complex web applications, PHP's approach is one of the integral parts of its programming.
The above is the detailed content of What are methods in PHP? How to define and use it?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


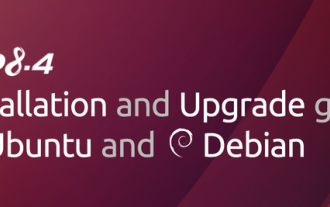
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
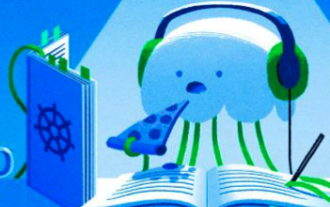
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
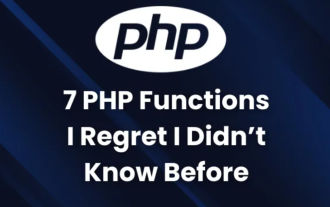
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
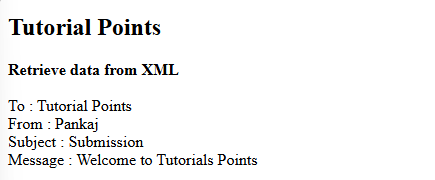
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
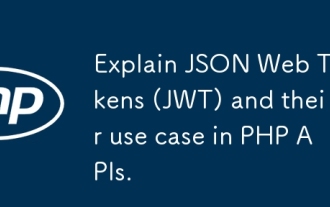
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
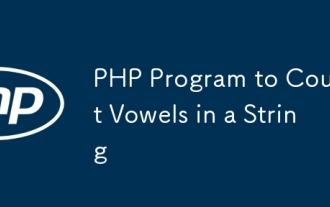
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
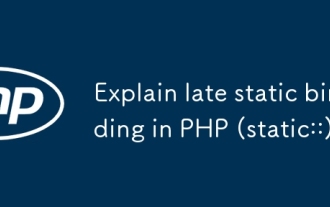
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
