


How to correctly read and write Session data in multiple files with PHP
When you are using a PHP session (Session), sometimes you will find that the Session can be read normally in one file, but cannot be read in another file. This may confuse you since session data is supposed to be shared across the entire application.
This article will explain how to correctly read and write PHP session data in multiple files.
-
Confirm whether the Session has been started
In files where Session data cannot be obtained, you must first ensure that the Session has been started. In PHP, you can use the session_start() function to start the Session. This function can be placed directly at the beginning of the PHP program. -
Confirm the consistency of Session ID
Session ID is a unique identifier used to identify the user's session. If the Session IDs are inconsistent, different files will not be able to read the same Session data. The Session ID is usually stored in a cookie called "PHPSESSID".
A common mistake is to use different Session IDs in different files. To solve this problem, it is better to store the Session ID in a PHP variable and use the same variable name in every file.
For example, in the first file:
session_start(); $session_id = session_id();
Then, in the second file:
session_id($session_id); session_start();
This ensures that both files use the same Session ID .
-
Check whether the Session data has been cleared
The Session data may have expired or been cleared. You can use the session_status() function to check the status of the Session.
If the Session is active, this function will return PHP_SESSION_ACTIVE. If the Session has expired or been cleared, PHP_SESSION_NONE is returned. If the Session is started but not activated, PHP_SESSION_DISABLED is returned.
To check if the Session is active, write the code as follows:
if (session_status() == PHP_SESSION_ACTIVE) { // Session is active } else { // Session is not active }
If you find that the Session has been cleared, you can destroy it using the session_destroy() function:
session_start(); session_destroy();
-
Confirm Session save path
Session data is stored in the server's temporary directory by default. However, if the server is configured with its own storage location, the path needs to be specified in the file. You can use the session_save_path() function to set the Session save path.
For example, in the first file:
session_save_path('/my/custom/session/path'); session_start();
Then, in the second file:
session_save_path('/my/custom/session/path'); session_start();
This ensures that both files use the same Session saving path. If the Session saving path is not specified, the session data may be saved on different servers, causing read failure.
-
Check Session file permissions
Session data is stored in a file, so you need to ensure that the file can be read and written. If the correct permissions are not configured, it may result in the inability to read or write Session data.
Make sure the correct permissions are set for the Session saving directory. If you're not sure how to set permissions, contact your hosting provider for help.
Summary
This article introduces five methods to correctly read and write PHP session data in multiple files. Please follow the above steps to check one by one to solve the problem of unable to read Session data.
The above is the detailed content of How to correctly read and write Session data in multiple files with PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


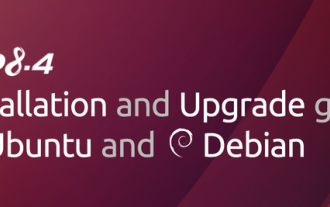
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
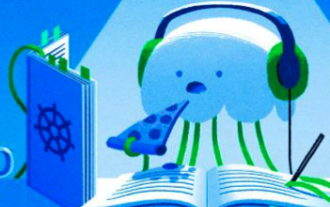
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
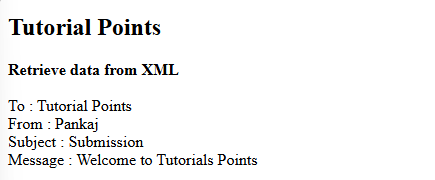
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
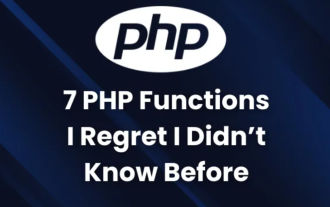
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
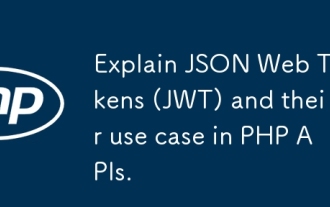
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
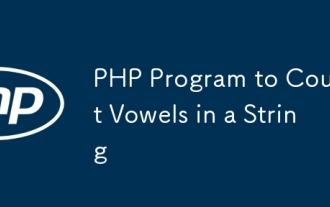
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
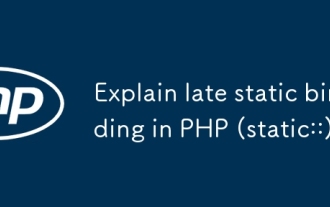
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
