How to call object method in php
PHP (Hypertext Preprocessor) is a popular open source server-side scripting language that can run on a Web server and is used to create dynamic web content for websites. In PHP, calling methods can be object methods or ordinary methods. The following uses object methods as an example to introduce how to call methods in PHP.
1. Create an object
In PHP, when calling an object method, you first need to create an object. Object is a special data type defined by a class that encapsulates data and methods. To create an object, you first need to define a class.
You can use the class keyword to define a class, followed by the class name. Class names must be unique, if the class name is the same as another defined class name, an error will occur. In a class definition, you can define properties and methods.
class MyClass { // 定义属性 public $name; public $age; // 定义方法 public function sayHello() { echo "Hello!"; } }
After defining the class, you can create an object through the new keyword.
$object = new MyClass();
$object here is an object variable, which stores an instance of the MyClass class.
2. Call object methods
Once the object is created, the object method can be called through the object variable. The syntax for calling object methods is: $object->methodName(). Among them, $object is the object variable and methodName is the method name. Parameters can be passed in parentheses.
$object->sayHello(); // 输出 Hello!
When calling object methods, you need to pay attention to the following points:
- Object variables must be declared and initialized before use.
- Methods must be defined in the class before they can be called.
- The method name must be the same as the method name defined in the class and is case-sensitive.
- Method calls can have parameters or not.
3. Access object properties
In addition to calling object methods, you can also access object properties in PHP. Object properties are variables defined in a class that store state information about the object. Object properties can be declared using the keywords public, protected, or private, indicating different access rights.
- Public properties: Public properties that can be accessed both outside and inside the class.
- protected attribute: Protected attribute, which can only be accessed inside the class and subclasses.
- Private attribute: Private attribute, which can only be accessed inside the class.
The syntax for accessing object properties is: $object->propertyName. Among them, $object is the object variable and propertyName is the property name.
class MyClass { // 定义属性 public $name; protected $age; private $gender; // 定义方法 public function setAge($newAge) { $this->age = $newAge; } public function getAge() { return $this->age; } } $object = new MyClass(); $object->name = 'Tom'; $object->setAge(20); echo $object->name; // 输出 Tom echo $object->getAge(); // 输出 20
When accessing object properties, you need to pay attention to the following points:
- Object variables must be declared and initialized before use.
- Attributes must be defined in the class before they can be accessed.
- The attribute name must be the same as the attribute name defined in the class, and is case-sensitive.
- Inside the class, you can use the keyword $this to refer to the current object.
- When accessing properties, you need to pay attention to the access permissions of the properties.
4. Summary
This article introduces how to call object methods in PHP. First, you need to Create an object and then use object variables and method names to call object methods. The article also introduces how to access object attributes. Attributes with different access permissions have different access methods. By studying this article, readers can learn how to use object methods for programming in PHP and improve code reusability and maintainability.
The above is the detailed content of How to call object method in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


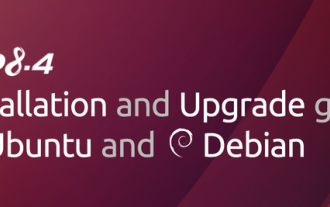
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
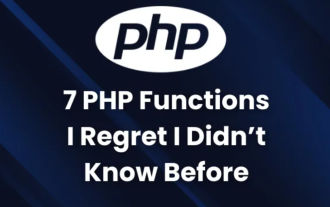
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
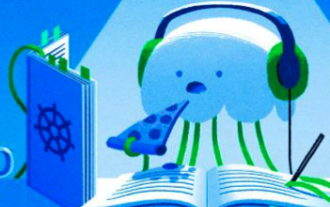
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
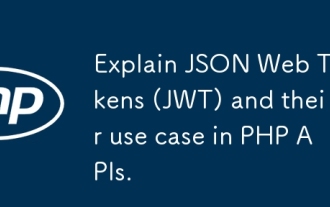
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
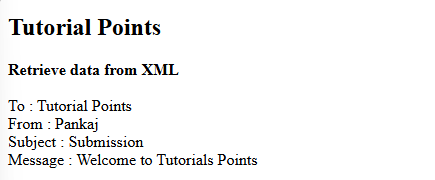
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
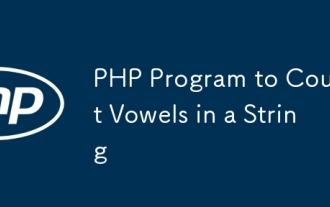
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
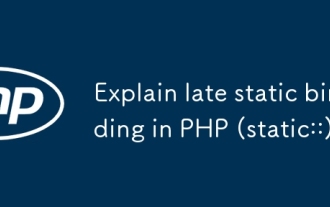
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
