How to implement Base64 encoding and decoding in php
Base64 algorithm is an encoding method that converts binary data into ASCII characters, so that the data can not be modified or damaged during transmission, and the true content of the data can also be hidden. In PHP, you can use built-in functions or manually write code to implement Base64 encoding and decoding.
- Use of PHP built-in functions
PHP provides two built-in functions base64_encode() and base64_decode(), which can easily perform Base64 Codec. The following is how to use them:
Base64 encoding:
$encoded_data = base64_encode($data);
Among them, $data is the binary data that needs to be encoded, and $encoded_data is the encoded string. Different from other encoding methods (such as URL encoding), the Base64-encoded string may contain special characters such as "/" and " ", which need to be escaped when transmitted in the URL.
Base64 decoding:
$decoded_data = base64_decode($encoded_data);
Among them, $encoded_data is the encoded string and $decoded_data is the decoded binary data.
This method is the simplest implementation, but when processing large binary data (such as image files), it will occupy a large amount of memory and cause performance problems.
- Manually write the Base64 algorithm
In order to process large binary data, we can manually write the implementation of the Base64 algorithm. The following is the code to manually implement Base64 encoding and decoding in PHP:
// Base64编码 function base64_encode_php($data) { $base64_map = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/"; $base64_data = ""; $data_len = strlen($data); for ($i = 0; $i < $data_len; $i += 3) { $chunk = ord(substr($data, $i, 3)); $base64_data .= $base64_map[($chunk >> 2) & 0x3F]; $base64_data .= $base64_map[(($chunk << 4) & 0x30) | ((ord(substr($data, $i + 1, 1)) >> 4) & 0x0F)]; $base64_data .= (($i + 2) < $data_len) ? $base64_map[(ord(substr($data, $i + 1, 1)) << 2) & 0x3C | (ord(substr($data, $i + 2, 1)) >> 6) & 0x03] : "="; $base64_data .= (($i + 2) < $data_len) ? $base64_map[ord(substr($data, $i + 2, 1)) & 0x3F] : "="; } return $base64_data; } // Base64解码 function base64_decode_php($data) { $base64_map = array( 'A' => 0, 'B' => 1, 'C' => 2, 'D' => 3, 'E' => 4, 'F' => 5, 'G' => 6, 'H' => 7, 'I' => 8, 'J' => 9, 'K' => 10, 'L' => 11, 'M' => 12, 'N' => 13, 'O' => 14, 'P' => 15, 'Q' => 16, 'R' => 17, 'S' => 18, 'T' => 19, 'U' => 20, 'V' => 21, 'W' => 22, 'X' => 23, 'Y' => 24, 'Z' => 25, 'a' => 26, 'b' => 27, 'c' => 28, 'd' => 29, 'e' => 30, 'f' => 31, 'g' => 32, 'h' => 33, 'i' => 34, 'j' => 35, 'k' => 36, 'l' => 37, 'm' => 38, 'n' => 39, 'o' => 40, 'p' => 41, 'q' => 42, 'r' => 43, 's' => 44, 't' => 45, 'u' => 46, 'v' => 47, 'w' => 48, 'x' => 49, 'y' => 50, 'z' => 51, '0' => 52, '1' => 53, '2' => 54, '3' => 55, '4' => 56, '5' => 57, '6' => 58, '7' => 59, '8' => 60, '9' => 61, '+' => 62, '/' => 63 ); $data_len = strlen($data); $padding_count = substr_count($data, "="); $binary_data = ""; $byte_count = 0; for ($i = 0; $i < $data_len; $i++) { $char = $data[$i]; if (isset($base64_map[$char])) { $byte_count++; $binary_data .= str_pad(decbin($base64_map[$char]), 6, "0", STR_PAD_LEFT); if ($byte_count == 4) { $byte_count = 0; $binary_data = substr($binary_data, 0, strlen($binary_data) - 8); } } } return substr($binary_data, 0, strlen($binary_data) - $padding_count * 8); }
This code is mainly divided into two parts: Base64 encoding and Base64 decoding.
In the Base64 encoding part, we first define a Base64 mapping table $base64_map, which is used to convert 6-bit binary data into corresponding Base64 characters. Then, for the input binary data, 3 bytes are taken out for processing at a time. For each 3-byte block of data, we will split it into 4 6-bit blocks. If the number of bytes currently processed is less than 3 bytes, the '=' character needs to be supplemented.
In the Base64 decoding part, we first define a Base64 mapping table $base64_map, this time it is used to convert each Base64 character into 6-bit binary data. For the input Base64 string, we convert it to binary data and then convert it to ASCII characters in groups of 8 bits.
Although this method is relatively complex, it can greatly improve performance because it can process large amounts of data in chunks.
Summary
Whether you use PHP built-in functions or manually write the Base64 algorithm, you can easily perform Base64 encoding and decoding operations. For small binary data, using built-in functions is sufficient, but for processing large binary data, hand-writing algorithms can improve performance.
The above is the detailed content of How to implement Base64 encoding and decoding in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


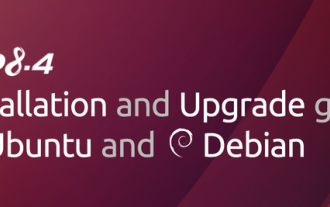
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
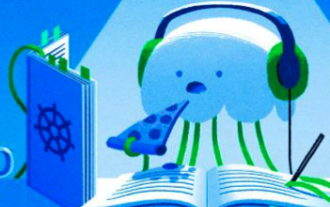
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
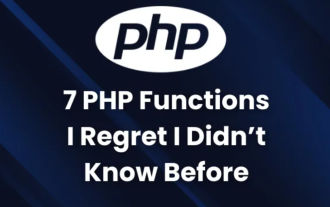
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
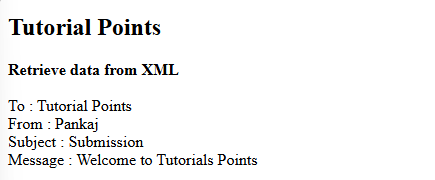
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
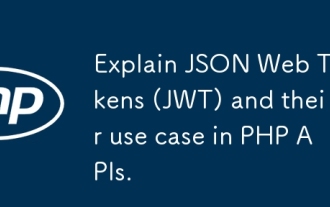
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
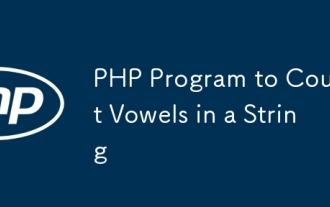
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
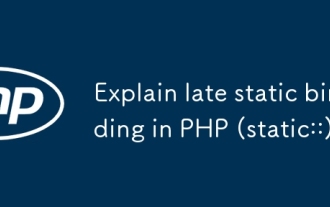
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
