How to wrap text in php? (code example)
PHP is a widely used server-side scripting language commonly used to build dynamic websites and web applications. In PHP, text wrapping is a common operation. However, some PHP beginners may encounter some problems when dealing with text wrapping, so this article will introduce in detail several PHP text wrapping codes and their applications.
1. Introduction to PHP text line wrapping
For text line wrapping, PHP provides three methods, namely:
- "\n ": represents a carriage return, that is, moves the output cursor to the beginning of the next line;
- "\r": represents a line feed, that is, only moves the cursor to the next line without a line break;
- " \r\n": represents carriage return and line feed, that is, moves the cursor to the beginning of the next line and breaks the line.
It should be noted that in different operating systems, the way text is wrapped is also different. For example, Windows systems use "\r\n", while Unix, Linux, and macOS systems can use "\n" or "\r".
2. PHP text line wrapping code implementation
1. Use "\n" to implement text line wrapping
<?php echo "Hello World!\n";//使用\n实现文本换行 echo "My name is John."; ?>
Output result:
Hello World! My name is John.
2. Use "\r" to implement text line wrapping
Principle of implementation: Now move the cursor to the next line, and then move the cursor to the beginning of the line.
<?php echo "Hello World!\r";//使用\r实现文本换行 echo "My name is John."; ?>
Output result:
My name is John! World!
3. Use "\r\n" to implement text line wrapping
Principle of implementation: Move the cursor to the beginning of the next line and wrap the text.
<?php echo "Hello World!\r\n";//使用\r\n实现文本换行 echo "My name is John."; ?>
Output result:
Hello World! My name is John.
3. Examples of application of PHP text line wrapping
- Text line wrapping in PHP email sending
In PHP email sending, text wrapping is very important. Suppose we want to send an email through PHP code, and the text needs to be wrapped in the email body. Please see the code implementation below.
<?php $to = 'john@example.com';//收件人邮箱 $subject = '测试邮件发送';//邮件主题 $message = "这是一封测试邮件。\r\n感谢您的支持!";//邮件正文 $headers = 'From: webmaster@example.com' . "\r\n" . 'Reply-To: webmaster@example.com' . "\r\n" . 'X-Mailer: PHP/' . phpversion(); mail($to, $subject, $message, $headers); ?>
- Text wrapping in PHP text file writing
In PHP, we can write a string into a file through the fwrite() function. If you need to wrap text in the file, use "\n" or "\r\n". The code below demonstrates how to write a multiline string to a file.
<?php $filename = "test.txt"; $file = fopen($filename,"w");//打开文件 $txt = "Hello World!\n"; fwrite($file,$txt);//写入文本换行 $txt = "My name is John.\n"; fwrite($file,$txt); fclose($file);//关闭文件 ?>
The above code will generate a file named test.txt and write the text content into the file.
4. Summary
This article briefly introduces several ways of text wrapping in PHP and its application scenarios. For beginners, it is very important to understand and master these knowledge points, so that functions such as text output, email sending, and text file writing can be easily and quickly implemented.
The above is the detailed content of How to wrap text in php? (code example). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


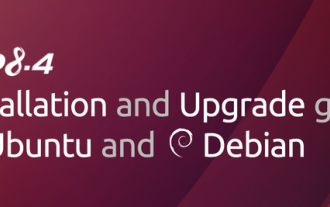
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
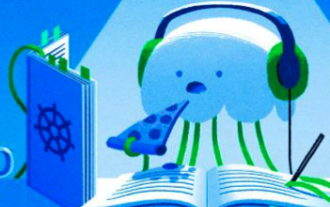
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
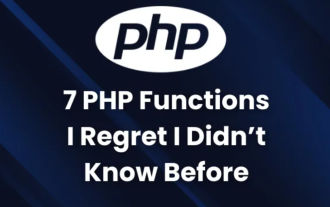
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
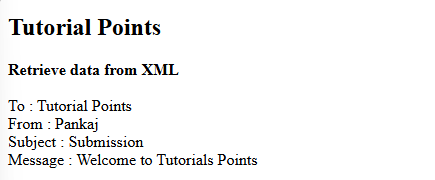
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
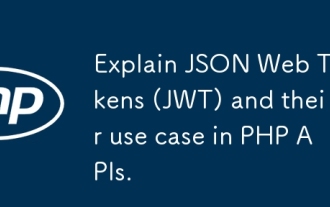
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
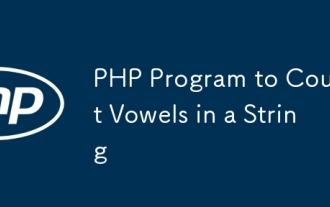
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
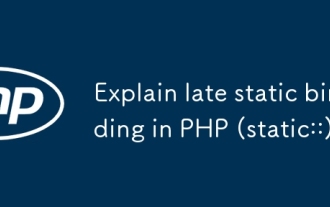
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
