How to convert golang byte to string
Golang is an efficient programming language that provides rich libraries and tools to meet various needs. When you need to convert byte type data to string, Golang provides a variety of convenient methods. In this article, we will introduce some methods to implement Golang byte to string conversion, including standard library methods and other related extension libraries.
1. Standard library methods
Golang’s standard library provides many methods for converting byte arrays or slices into strings. The most common method is to use the string() function.
- Use the string() function to convert:
This method is very simple, just pass the byte array or slice into the string() function. The sample code is as follows:
package main import "fmt" func main() { byteData := []byte{'h', 'e', 'l', 'l', 'o'} strData := string(byteData) fmt.Println(strData) }
The output result of this code block is: hello
- Use the ToString function in the bytes package for conversion:
bytes package The ToString() function is also provided, which can convert a byte array or slice into a string. Unlike the string() function, the ToString() function always returns the copied result and has no effect on the source data. The sample code is as follows:
package main import ( "bytes" "fmt" ) func main() { byteData := []byte{'h', 'e', 'l', 'l', 'o'} strData := bytes.ToString(byteData) fmt.Println(strData) }
The output result of this code block is: hello
- Use the Itoa() function in the strconv package for conversion:
When we need to convert byte type data into an integer represented by a string, we can use the strconv.Atoi() function to complete the conversion. The sample code is as follows:
package main import ( "fmt" "strconv" ) func main() { byteData := []byte{'1', '2', '3', '4', '5'} intData, _ := strconv.Atoi(string(byteData)) fmt.Println(intData) }
The output result of this code block is: 12345
2. Extension library methods
In addition to the standard library, there are many extensions in the Golang community Library that provides other efficient methods for byte to string conversion. Here, we introduce two extension function libraries: Babelfish and Go-Conversion.
- Babelfish library
Babelfish is a multi-language translation library for Golang. It provides a variety of conversion methods, including byte to multiple string format conversions . Babelfish provides functions such as ToUTF8String(), ToAsciiString() and ToString(). We take ToUTF8String() as an example. The sample code is as follows:
package main import ( "fmt" "github.com/axgle/babel" ) func main() { byteData := []byte{'h', 'e', 'l', 'l', 'o'} strData := babel.ToUTF8String(byteData) fmt.Println(strData) }
The output result of this code block is: hello
- Go-Conversion Library
Go-Conversion is a tool library for converting between byte arrays and other types. It provides functions such as FromBytesToString(), FromHexToString() and FromBase64ToString() for converting byte arrays into string format. This article takes the FromBytesToString() function as an example. The sample code is as follows:
package main import ( "fmt" "github.com/radovskyb/go-packages/convert" ) func main() { byteData := []byte{'h', 'e', 'l', 'l', 'o'} strData, err := convert.FromBytesToString(byteData) if err != nil { panic(err) } fmt.Println(strData) }
The output result of this code block is: hello
Conclusion
Convert byte to string in Golang Very easy, using standard library functions is the simplest way. However, some extension libraries provide methods that provide more functionality and flexibility. Choose the most suitable method according to your needs. No matter which method is used, remember to consider the errors that may occur during conversion and perform appropriate error handling.
The above is the detailed content of How to convert golang byte to string. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


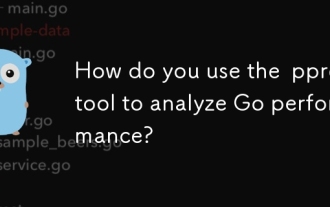
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
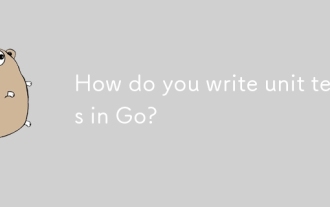
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
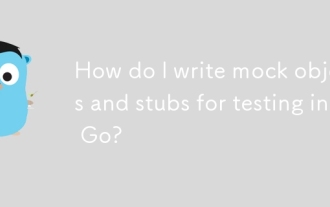
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
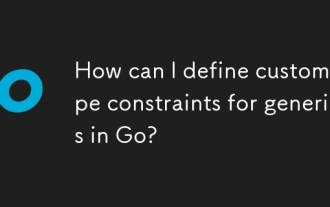
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
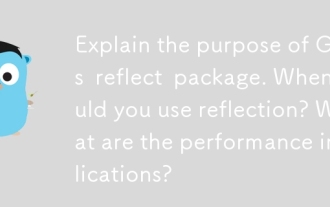
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
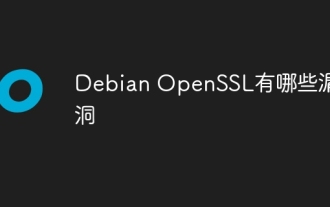
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
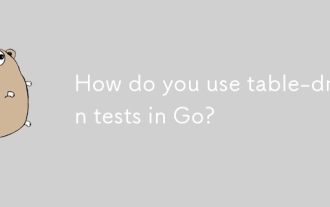
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
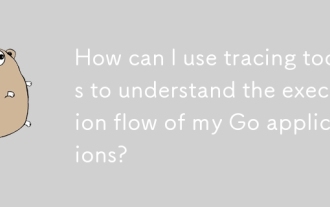
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
