Detailed explanation of Map modification operations in Golang
Golang is a strongly typed compiled language, and its map data type is a very powerful and commonly used data structure. Map can be used to store a set of key-value pairs, where each key can appear only once. Map in Golang can add or update operations through assignment statements. However, some developers may encounter some problems, such as how to safely modify the value of the Map when looping through the Map, or how to prevent concurrent modification of the Map in the program. This article will explore the modification operations of Map in Golang and the precautions.
1. Golang Map adds a value to an existing Key
In Golang, adding a value to an existing Key is very simple. This can be achieved through assignment statements. For example:
map1 := make(map[string]string) map1["key1"] = "value1" map1["key2"] = "value2" map1["key1"] = "newvalue1"
In the above example, we declared a Map object map1, and then added two key-value pairs to map1. Then we modified the value corresponding to the key key1. At this time, all key-value pairs in map1 are as follows:
map1 = map[string]string{ "key1": "newvalue1", "key2": "value2", }
2. Pitfalls in Golang Map
Although adding values to existing Keys is very simple, in actual use , you still need to understand some basic Map operation methods and issues that need attention.
- Map is unordered
In Golang, the key-value pairs of Map are unordered, so there is no guarantee that the order of map1 during the traversal is the same as ours The order of addition is consistent. If you need to traverse the Map in order, you can convert it into an ordered data type.
- The type of Key in Map must be the same
The Key in Map must be of the same type. For example, if map1 is a map of string keys and int values, you cannot use float64 keys to add or get the corresponding values. Otherwise a runtime error will occur. If you need a different type of key, you can use the interface type as the Key.
- The value in the Map is a direct reference
In Golang, the value in the Map is a direct reference, not a copy. This means that if you modify the content of a value, the modification will also affect the value in the Map corresponding to the value. For example:
map2 := make(map[string][]int) map2["key1"] = []int{1, 2, 3} slice := map2["key1"] slice[0] = 100
In the above example, we declared a Map object map2. Then we assign an array to the value corresponding to the key key1, then we declare a slice, and then assign the value in map2 to this slice. Finally, we modified the first element in this slice, not the value corresponding to the key Key1 in Map2. However, after this modification, the value corresponding to Key1 in Map2 has also changed. Therefore, special attention should be paid when operating Map.
3. Concurrent modification of Golang Map
When using Map, you may face the situation where multiple Go coroutines modify the same Map at the same time. In this case, we must take some measures to prevent program crashes or data errors. The following are several solutions to concurrent modification of Map in Golang:
- Use sync.Map
In Golang, sync.Map is a thread-safe Map type . Unlike ordinary Map, sync.Map can be safely shared and modified among multiple coroutines, and can effectively reduce lock competition in the case of a large number of concurrent reads and writes.
- Use channels to operate asynchronously Map
Channel is a powerful tool for concurrency control in Golang. Channels can be used to pass Map operations to other coroutines to avoid conflicting multi-threaded access to the Map.
- Using Mutex (Mutex)
Mutex (Mutex) is a mechanism to achieve thread safety in Golang. A mutex can be used to protect concurrent modifications to the Map. During the operation of Map, the security of concurrent access is controlled through the Lock and Unlock methods.
4. Summary
In Golang, Map is a very powerful and commonly used data type that can be used to store a set of key-value pairs. In the process of modifying Map, you need to pay attention to issues such as disorder, same type, direct reference to value, and concurrent modification. You can use sync.Map, channels, mutexes, etc. to ensure the thread safety of Map. Failure to understand these issues well can lead to program anomalies and inefficient code. Therefore, when using Map, you need to have sufficient understanding and understanding to be able to correctly apply concurrent operation technology to ensure the stability and performance of the program.
The above is the detailed content of Detailed explanation of Map modification operations in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


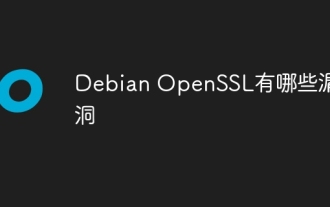
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
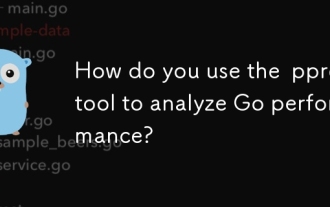
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
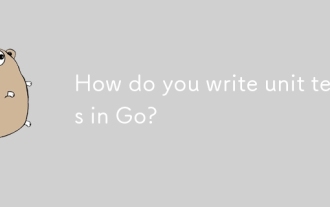
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
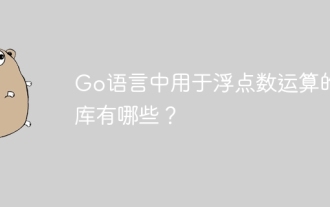
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
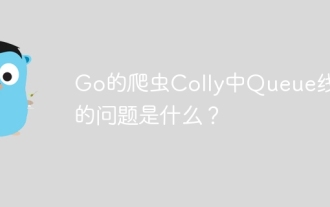
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
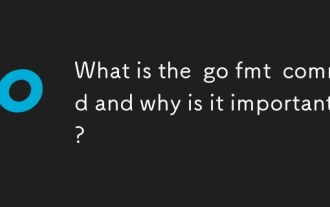
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
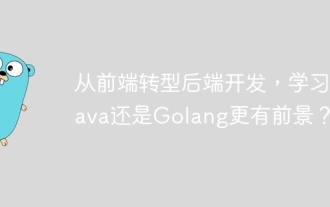
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
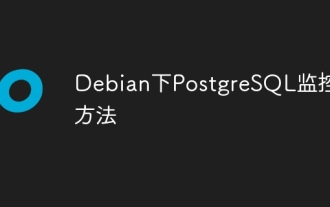
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
