How to implement NFS using Golang
Golang is a strongly typed programming language with the advantages of efficiency, security, and easy maintenance. With the popularity of cloud storage, NFS has become one of the most commonly used network file systems in cloud storage. This article will introduce how to use Golang to implement NFS.
1. Architecture and Principles of NFS
NFS (Network File System) is a distributed file system that allows files and directories to be shared between computers on the network. NFS adopts the client-server model. The client sends the request to the server through the network, and the server executes the request and returns the result to the client.
The NFS client sends the request to the NFS server through the NFS protocol, and the NFS server parses the request and returns the result to the NFS client. The NFS client and NFS server communicate through the network, and the communication between them uses the TCP protocol.
The implementation principle of NFS is as follows:
- The client sends a request to the server, and the request contains information such as file name, file operation type, etc.
- After the server receives the request, it determines the request type. If it is a read request, the data is read from the disk and sent to the client. If it is a write request, the data is written to the disk.
- After receiving the data returned by the server, the client operates the file through the file operation interface.
2. Golang implements NFS
In Golang, you can use the network programming library to implement NFS. Below is a simple NFS client program written in Golang.
package main import ( "fmt" "net" ) func main() { // 连接NFS服务器端 conn, err := net.Dial("tcp", "127.0.0.1:8888") if err != nil { fmt.Println("Connect error!") return } // 发送请求 conn.Write([]byte("filename=/test.txt&action=read")) // 接收响应 buf := make([]byte, 1024) n, err := conn.Read(buf) if err != nil { fmt.Println("Read error!") return } // 处理响应结果 result := string(buf[:n]) fmt.Println("Result:", result) // 关闭连接 conn.Close() }
The above program establishes a TCP connection with the NFS server through the Dial function in the net package, sends a request to the NFS server, receives the response result returned by the NFS server, and outputs it to the console.
3. Summary
This article briefly introduces the architecture and principles of NFS, and implements a simple NFS client program through Golang. Golang has the advantages of high efficiency, security, and easy maintenance, making it an excellent choice for implementing NFS. However, Golang's network programming library is not complete enough compared to the network programming libraries of other programming languages, and more developers are needed to improve it.
The above is the detailed content of How to implement NFS using Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
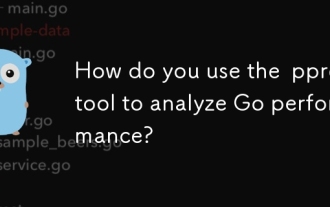
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
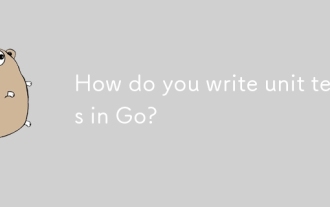
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
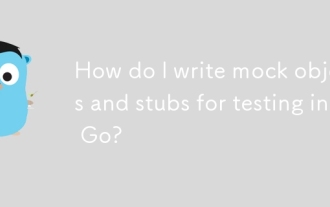
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
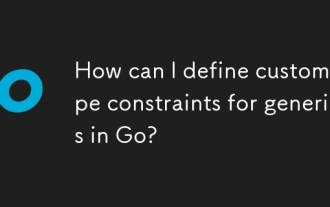
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
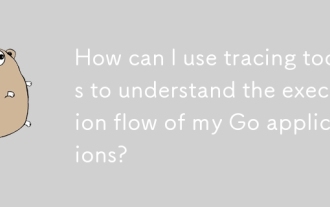
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
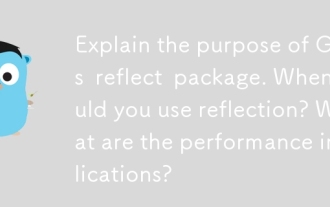
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
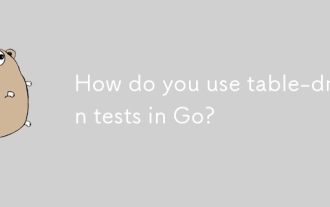
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
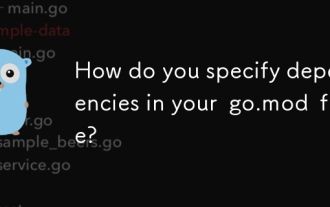
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
