How to transmit data in golang
Golang (also known as the Go language) is a programming language for creating efficient and scalable web applications. In web development, transferring data is a very common operation. So, how do we transfer data in Golang? Next, this article will show some methods of transferring data.
1. Transmitting data through HTTP protocol
HTTP is an application-level protocol, mostly used to transmit Web pages and related content. Go language has a built-in HTTP package, which can easily use HTTP to transmit data. The following is a simple HTTP data transmission example:
package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) } func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, %s!", r.URL.Path[1:]) }
The above code uses the http.HandleFunc() function to specify the HTTP routing processing function, and then uses the http.ListenAndServe() function to start the specified TCP port. monitor. The handler() function is used to specifically handle requests in HTTP routing. Here it simply replies with a "Hello" string. Run the program and enter http://localhost:8080/ in the web browser. You can see "Hello, /!" displayed on the browser, indicating that the data is successfully transmitted through the HTTP protocol.
2. Transmitting data through TCP protocol
TCP (Transmission Control Protocol) is usually used to transmit data streams in the network, and is sometimes called a connection-oriented protocol. The Go language network package includes TCP support. The following is a simple TCP data transmission example:
package main import ( "fmt" "net" ) func main() { lis, _ := net.Listen("tcp", "localhost:8080") defer lis.Close() for { conn, _ := lis.Accept() go handleConnection(conn) } } func handleConnection(conn net.Conn) { buffer := make([]byte, 1024) n, _ := conn.Read(buffer) fmt.Println(string(buffer[:n])) conn.Write([]byte("Hello from server")) conn.Close() }
The above code starts a TCP service on local port 8080 through the net.Listen() function. When the client requests to establish a TCP connection with the server, the server generates a new goroutine process to handle the TCP connection. In the handleConnection() function, the data from the client is read through the conn.Read() function, and then the data is output on the server. Then, reply to the client with a "Hello from server" string through the conn.Write() function. After the client receives the reply, the socket is closed. At this point, the data is successfully transmitted through the TCP protocol.
3. Transmitting data through UDP protocol
UDP is the user datagram protocol. This protocol is connectionless-oriented, so it can be used to transmit datagrams across the network without confirming the recipient. Are you ready to receive data? The Go language network package supports the UDP protocol. The following is a simple UDP data transmission example:
package main import ( "fmt" "net" ) func main() { addr, _ := net.ResolveUDPAddr("udp", ":8080") conn, _ := net.ListenUDP("udp", addr) defer conn.Close() buffer := make([]byte, 1024) n, addr, _ := conn.ReadFromUDP(buffer) fmt.Println(string(buffer[0:n])) conn.WriteToUDP([]byte("Hello from server"), addr) }
The above code uses the net.ResolveUDPAddr() function to parse the UDP address, and uses the net.ListenUDP() function to create a read-write UDP data connection. . When the client sends data to the server through this connection, the server reads the data through the conn.ReadFromUDP() function, then performs some processing, and replies with a message through the conn.WriteToUDP() function. After the data is successfully transferred, the UDP connection will be closed.
To sum up, Golang can transmit data through different protocols such as HTTP, TCP and UDP. Programmers can choose appropriate data transmission methods according to different application scenarios and specific needs.
The above is the detailed content of How to transmit data in golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
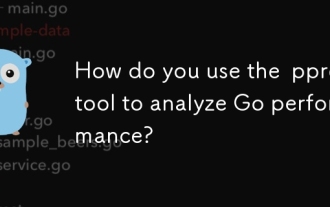
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
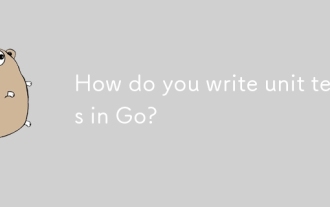
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
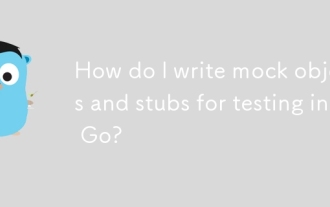
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
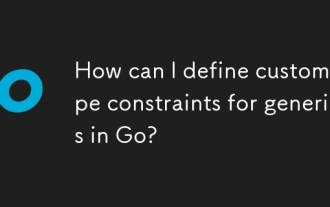
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
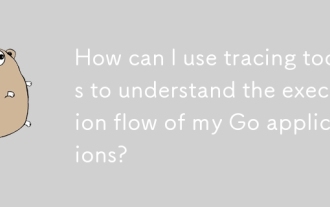
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
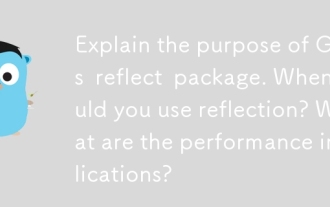
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
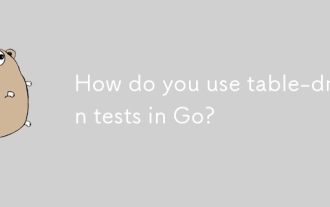
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
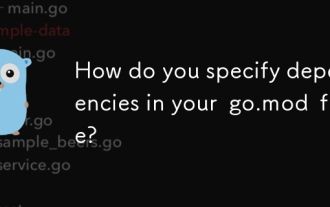
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
