How to remove spaces in content with golang crawler
Golang is an efficient programming language that is widely used in various application development, including web crawlers. This article will focus on how to use Golang to write a crawler and remove spaces from the crawled content.
- Crawling HTML pages
The crawler needs to initiate an HTTP request to obtain the website page. The following code snippet can achieve this function:
import ( "fmt" "net/http" ) func main() { response, err := http.Get("https://www.example.com") if err != nil { fmt.Println("HTTP请求错误:", err) } defer response.Body.Close() // 处理HTTP响应内容 }
- Processing HTTP response content
Processing HTTP response content requires the help of a third-party library. For example, use the goquery
library to parse the HTML page, and then use the strings
library. Function removes spaces. The specific code is as follows:
import ( "fmt" "github.com/PuerkitoBio/goquery" "net/http" "strings" ) func main() { response, err := http.Get("https://www.example.com") if err != nil { fmt.Println("HTTP请求错误:", err) } defer response.Body.Close() // 解析HTML页面 document, err := goquery.NewDocumentFromReader(response.Body) if err != nil { fmt.Println("解析HTML页面错误:", err) } // 获取HTML页面中的所有文本内容并去除空格 text := strings.TrimSpace(document.Text()) fmt.Println(text) }
goquery
library is a very easy-to-use HTML parsing library that can easily obtain any element in the page without worrying about pointers and memory management in the Go language. question.
- Write the processed text to a file
After processing the text content, you usually need to write it to a file, which can be achieved through the following code:
import ( "fmt" "github.com/PuerkitoBio/goquery" "io/ioutil" "net/http" "strings" ) func main() { response, err := http.Get("https://www.example.com") if err != nil { fmt.Println("HTTP请求错误:", err) } defer response.Body.Close() // 解析HTML页面 document, err := goquery.NewDocumentFromReader(response.Body) if err != nil { fmt.Println("解析HTML页面错误:", err) } // 获取HTML页面中的所有文本内容并去除空格 text := strings.TrimSpace(document.Text()) // 将文本内容写入文件 err = ioutil.WriteFile("output.txt", []byte(text), 0644) if err != nil { fmt.Println("写入文件错误:", err) } }
- Summary
The above is how to use Golang to write a crawler and remove spaces in the crawled content. Get the page through HTTP request, use the goquery
library to parse the HTML, then use the strings
library to remove spaces, and finally write the results to a file. Writing efficient crawlers requires experience, but using Golang allows developers to easily write efficient web crawlers.
The above is the detailed content of How to remove spaces in content with golang crawler. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
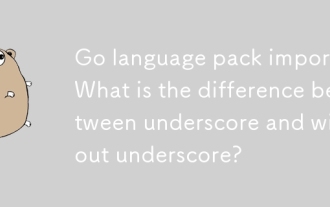
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
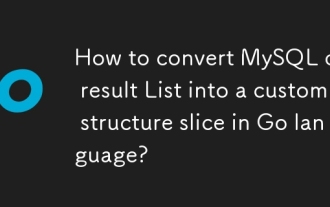
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
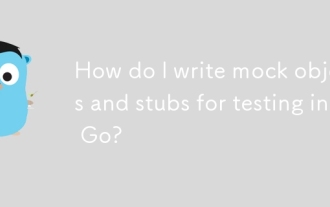
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
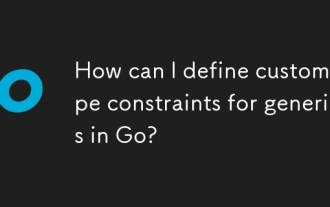
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
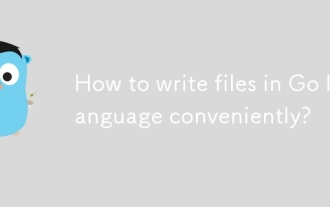
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
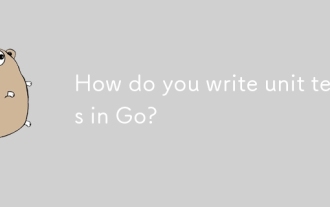
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
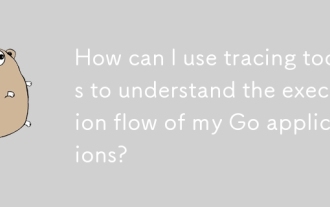
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
