Introducing some common Golang errors
Golang is a very popular programming language, but even the most experienced developers will inevitably encounter some mistakes. This article will introduce some common Golang errors and provide suggestions for solving them.
- Type mismatch
In Golang, type is very important. When you operate with mismatched types, the program will fail to compile or cause a runtime error. For example, the following code will not compile:
var x int = "hello"
Because x has been defined as an int, but as a string.
Solution: Make sure you are using the correct types in your code. If you are not sure about the type of a variable, use the var
or :=
keyword to specify the type.
- Null pointer reference
Null pointer reference is another very common error in Golang. For example, the following code will cause the program to crash:
var p *int fmt.Println(*p)
Because the pointer variable p does not point to any address, the program crashes when we try to access it.
Solution: Make sure you have allocated memory for a pointer when using it, and that it points to a valid address. You can use the new
function or the make
function to allocate memory for pointer variables.
- Array out of bounds
In Golang, an array is a fixed-size data structure. When you try to access an element that does not exist in the array, it will cause the program to crash.
For example, the following code will cause a crash when trying to access the 4th element of the array:
var arr [3]int = [3]int{1,2,3} fmt.Println(arr[3])
Solution: Make sure you don't go out of bounds when accessing the array. You can use the len
function to get the length of an array and then check it before accessing the array elements.
- Recursive Stack Overflow
Golang supports recursive calls, but if the recursion depth is too deep, a stack overflow error will occur.
For example, the following code will cause the program to crash:
func main(){ var n int = 10000 fmt.Println(factorial(n)) } func factorial(n int) int { if n == 0 { return 1 } return n * factorial(n-1) }
Solution: Please make sure that when you use recursion, the recursion depth is not too deep. You can replace recursion with a loop, or use tail recursion to avoid stack overflow.
- Concurrency race conditions
Concurrency is a core feature of Golang, but concurrent programs are prone to race conditions. A race condition occurs when multiple threads access a shared resource, which may lead to undefined behavior. For example, the following code will display the numbers in the list:
func main() { var wg sync.WaitGroup for i := 0; i < 10; i++ { wg.Add(1) go func() { fmt.Println(i) wg.Done() }() } wg.Wait() }
You might expect an output like this:
0 1 2 3 4 5 6 7 8 9
But in reality, the output might be:
9 9 9 9 9 9 9 9 9 9
This is because Multiple threads access the same variable i, and the value of this variable is continuously updated in the loop.
Solution: Please ensure that you access shared resources correctly and synchronously when using concurrency. You can use locks to protect shared resources or channels to avoid race conditions.
Summary
In Golang, errors are very common. However, if you follow best practices, you will be able to avoid most mistakes. Make sure you write your code with attention to detail and debug errors with patience and care.
The above is the detailed content of Introducing some common Golang errors. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
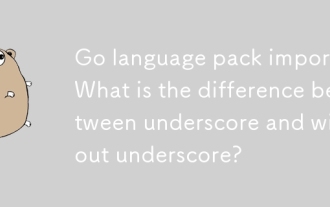
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t
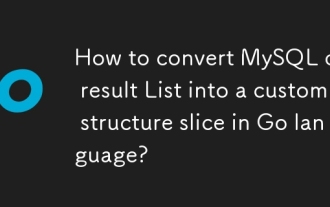
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
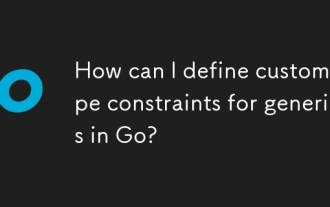
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
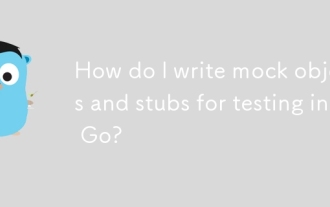
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
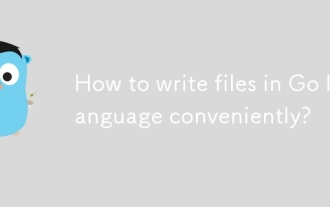
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
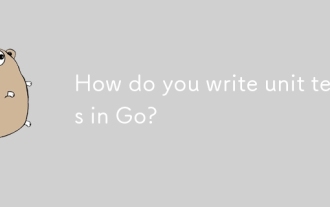
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
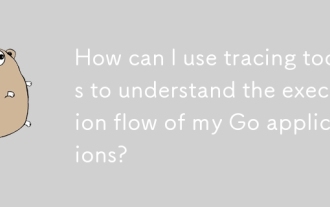
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
