


How to use php and mysql to add, delete, modify and check classmate records
In recent years, with the development of the Internet, various types of websites have emerged, among which educational websites have also attracted more and more attention. As a tool for recording student information, classmate directory has also become an indispensable feature of many school and community websites. When building the classmate record function, the combination of php and mysql allows us to easily add, delete, modify and check the classmate record.
1. Preparations before adding, deleting, modifying and checking classmate records
Before we start building the classmate record function, what we need to prepare is a basic template of a web page. In order to better reflect the role of php and mysql, some forms and buttons need to be added to the template to add, delete, modify and check the student records.
Add a table to the template to store various information in the classmate directory. Then you need to add some headers to the table to match the subsequent data.
2. To add classmates to the list
To add classmates, you need to create a form to input the information of the students to be added, including name, student number, major, class, etc. . In PHP, form submission and data storage can be achieved through code.
Code snippet:
<?php if(isset($_GET['submit'])){ $name = $_GET['name']; $id = $_GET['id']; $major = $_GET['major']; $class = $_GET['class']; $mysqli = new mysqli('localhost','root','','studentdatabase'); if(mysqli_connect_errno()){ echo mysqli_connect_error(); exit(); } $insert_sql = "INSERT INTO student (name,id,major,class) VALUES ('$name','$id','$major','$class')"; if($mysqli->query($insert_sql)){ echo "添加学生成功"; }else{ echo "添加学生失败"; } } ?> <form method="get" action="#"> 姓名:<input type="text" name="name"> 学号:<input type="text" name="id"> 专业:<input type="text" name="major"> 班级:<input type="text" name="class"> <input type="submit" name="submit" value="添加学生信息"> </form>
3. Editing the classmate record
The information in the classmate record can be edited. For this purpose, you need to add a Edit button. When the user clicks the edit button, an edit box should appear to modify the information. Similarly, in PHP, form submission and data storage are implemented through code.
Code snippet:
<?php if(isset($_GET['modify'])){ $id = $_GET['id']; $mysqli = new mysqli('localhost','root','','studentdatabase'); if(mysqli_connect_errno()){ echo mysqli_connect_error(); exit(); } $select_sql = "SELECT * FROM student WHERE id = '$id'"; if($result = $mysqli->query($select_sql)){ $row = $result->fetch_assoc(); ?> <form method="get" action="#"> 姓名:<input type="text" name="name" value="<?php echo $row['name']; ?>"> 学号:<input type="text" name="id" value="<?php echo $row['id']; ?>" readonly> 专业:<input type="text" name="major" value="<?php echo $row['major']; ?>"> 班级:<input type="text" name="class" value="<?php echo $row['class']; ?>"> <input type="submit" name="update" value="保存"> </form> <?php $result->free(); } } if(isset($_GET['update'])){ $name = $_GET['name']; $id = $_GET['id']; $major = $_GET['major']; $class = $_GET['class']; $mysqli = new mysqli('localhost','root','','studentdatabase'); if(mysqli_connect_errno()){ echo mysqli_connect_error(); exit(); } $update_sql = "UPDATE student SET name='$name',major='$major',class='$class' WHERE id='$id'"; if($mysqli->query($update_sql)){ echo "学生信息修改成功"; }else{ echo "学生信息修改失败"; } } ?>
4. Realize the deletion of the classmate record
The information in the classmate record can also be deleted. In php, the database is deleted by obtaining the data submitted by the user.
Code snippet:
<?php if(isset($_GET['delete'])){ $id = $_GET['id']; $mysqli = new mysqli('localhost','root','','studentdatabase'); if(mysqli_connect_errno()){ echo mysqli_connect_error(); exit(); } $delete_sql = "DELETE FROM student WHERE id = '$id'"; if($mysqli->query($delete_sql)){ echo "学生信息删除成功"; }else{ echo "学生信息删除失败"; } } ?>
5. Implement the query of the classmate record
The information in the classmate record can be searched by keywords. If you want to implement the query function of classmate records, you need to write query statements in PHP and provide users with a search box and search button on the web page.
Code snippet:
<?php if(isset($_POST['search'])){ $keyword = $_POST['keyword']; $mysqli = new mysqli('localhost','root','','studentdatabase'); if(mysqli_connect_errno()){ echo mysqli_connect_error(); exit(); } $select_sql = "SELECT * FROM student WHERE name LIKE '%$keyword%' OR id LIKE '%$keyword%' OR major LIKE '%$keyword%' OR class LIKE '%$keyword%'"; if($result = $mysqli->query($select_sql)){ while($row = $result->fetch_assoc()){ echo "<tr>"; echo "<td>".$row['name']."</td>"; echo "<td>".$row['id']."</td>"; echo "<td>".$row['major']."</td>"; echo "<td>".$row['class']."</td>"; echo "<td><a href='?modify=1&id=".$row['id']."'>修改</a> <a href='?delete=1&id=".$row['id']."'>删除</a></td>"; echo "</tr>"; } $result->free(); } } ?> <form method="post" action="#"> <input type="text" name="keyword" value=""> <input type="submit" name="search" value="搜索"> </form>
6. Summary
Through the above implementation, we can easily complete the functions of adding, editing, deleting and querying student records. The combination of php and mysql can greatly improve development efficiency and flexibility in implementing functions. I hope that the above content can provide reference and reference for beginners of PHP and MySQL.
The above is the detailed content of How to use php and mysql to add, delete, modify and check classmate records. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


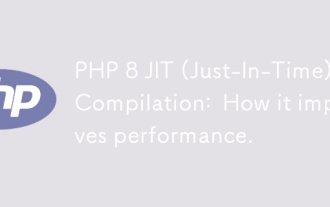
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
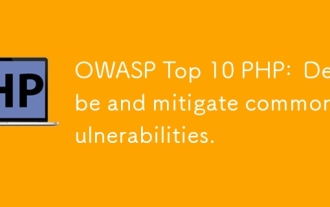
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
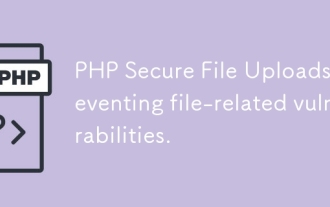
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
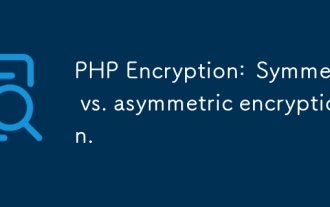
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
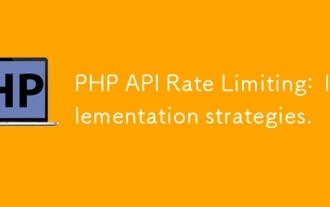
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
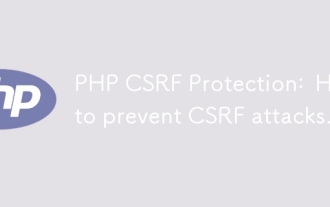
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
