How to query results from database using PHP
With the booming development of the Internet and mobile Internet, the development of websites and applications has become a top priority. And in these applications, interaction with the database is essential. Therefore, learning how to query data from the database has become one of the skills that every developer must master, especially for websites and applications developed using PHP.
PHP is a widely used server-side scripting language that is designed for web development and can be embedded in HTML. PHP can interact with various databases, such as MySQL, Oracle, and PostgreSQL. In this article, we will focus on how to use PHP to query results from the database.
The first step is to connect to the database. In PHP, we can use extension libraries such as mysqli or PDO to connect to the database. The following is an example of using the mysqli extension library to connect to the database:
<?php $servername = "localhost"; $username = "root"; $password = ""; $dbname = "myDB"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检测连接 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } echo "连接成功"; ?>
The $servername, $username, $password and $dbname variables in the code need to be replaced with your actual values. If the connection fails, the code returns a connection error message and terminates the program. If the connection is successful, "Connection successful" will be output.
The second step is to query the data from the database. In the mysqli extension library, we can use the query() method to execute queries. The following is a simple query example:
$sql = "SELECT * FROM myTable"; $result = $conn->query($sql); if ($result->num_rows > 0) { // 输出每行数据 while($row = $result->fetch_assoc()) { echo "id: " . $row["id"]. " - Name: " . $row["name"]. " - Email: " . $row["email"]. "<br>"; } } else { echo "0 结果"; }
The $sql variable in the code needs to be replaced with your actual query statement. If the query is successful, the code will output the id, name and email information of each row of data. If the query has no results, "0 results" will be output.
In the above code, the fetch_assoc() method returns a row of records and moves the pointer to the next row. If there are no more rows, return false.
If you are using the PDO extension library, you can use the query() method to perform queries. The following is a sample code using the PDO extension library:
$sql = 'SELECT * FROM myTable'; foreach ($conn->query($sql) as $row) { print $row['id'] . "\t"; print $row['name'] . "\t"; print $row['email'] . "<br>"; }
In the above code, the query() method returns a PDOStatement object, which can be iterated by the foreach loop. We can then use the $row variable to access the field values of each row of records.
The third step is to release the result set and close the connection. Once we have fetched the required data, we need to release the result set and close the database connection. This is a good programming practice and also helps to avoid memory leaks. The following is an example of releasing the result set and closing the database connection:
$result->free(); $conn->close();
This article describes how to use PHP to query results from the database. Many PHP developers have already mastered these skills, but for beginners, this is a great starting point. They are an important foundation for learning subsequent PHP development and are also critical steps in building web applications. Hope this article helps you!
The above is the detailed content of How to query results from database using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
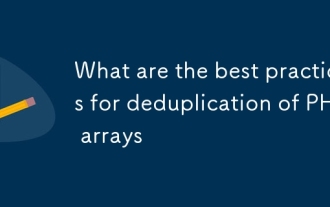
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
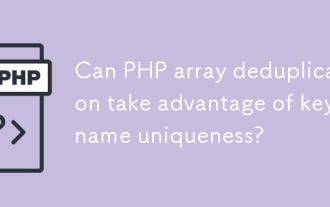
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
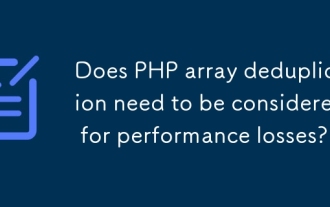
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
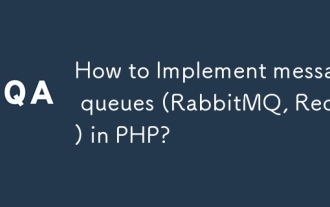
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
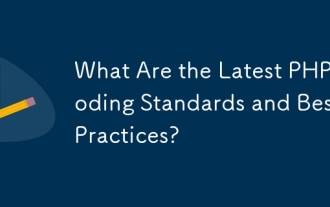
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
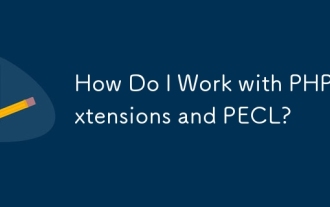
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
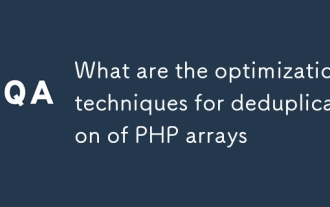
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
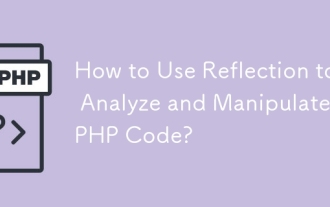
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
